How to Square a Number in Python
Daniel Hayes
Full-Stack Engineer · Leapcell
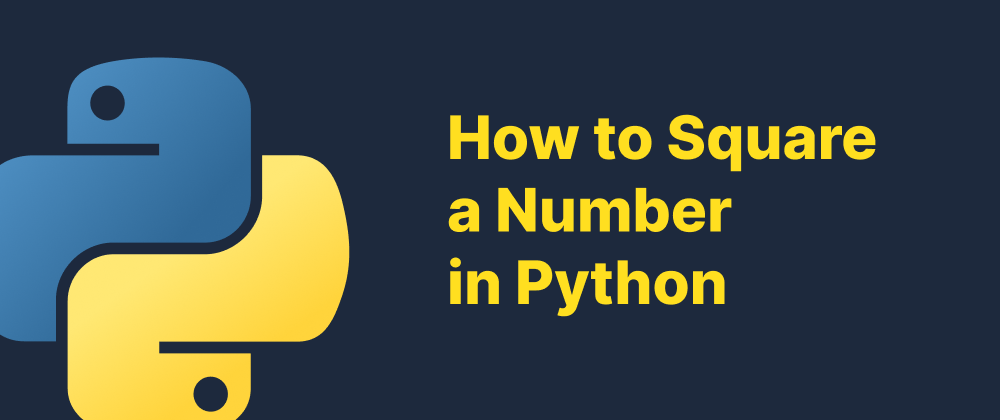
Key Takeaways
- You can square a number using
**
,*
, orpow()
in Python. math.pow()
always returns a float.- Simple multiplication (
*
) is fastest and clearest for most cases.
Squaring a number is a fundamental mathematical operation, and it's a very common task in programming as well. In Python, there are several simple ways to square a number. This article will guide you through the most commonly used methods to square a number in Python.
What Does "Squaring a Number" Mean?
Squaring a number means multiplying the number by itself. For example:
- ( 2^2 = 4 )
- ( 5^2 = 25 )
- ( (-3)^2 = 9 )
In Python, there are multiple ways to achieve this.
Method 1: Using the Exponentiation Operator (**
)
Python uses the **
operator for exponentiation. To square a number, raise it to the power of 2:
number = 4 squared = number ** 2 print(squared) # Output: 16
This method is straightforward and works with both integers and floats.
Method 2: Using Multiplication
You can also simply multiply the number by itself:
number = 4 squared = number * number print(squared) # Output: 16
This approach is also very clear and easy to read, and it's slightly faster in performance for simple squaring.
Method 3: Using the math.pow()
Function
The math
module provides the pow()
function, which can also be used to raise a number to a specific power:
import math number = 4 squared = math.pow(number, 2) print(squared) # Output: 16.0
Note that math.pow()
always returns a float, even if the input is an integer.
Method 4: Using the Built-in pow()
Function
Python also has a built-in pow()
function, which behaves similarly to **
but has some additional features like modular exponentiation:
number = 4 squared = pow(number, 2) print(squared) # Output: 16
This is functionally equivalent to number ** 2
but allows a third argument for modulus, which is useful in some advanced cases.
Conclusion
There are several ways to square a number in Python, including using the **
operator, simple multiplication, or built-in functions like pow()
and math.pow()
. Each method has its own advantages depending on your needs:
- Use
**
or*
for simplicity and speed. - Use
pow()
when you need more flexibility or are working with modular arithmetic.
No matter which method you choose, Python makes it easy to perform this basic operation with just one line of code.
FAQs
Using number ** 2
or number * number
is the simplest way.
math.pow()
returns a float; built-in pow()
can return integers and supports modular arithmetic.
Yes, all methods correctly handle negative numbers.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ