Understanding Floor Division in Python
Olivia Novak
Dev Intern · Leapcell
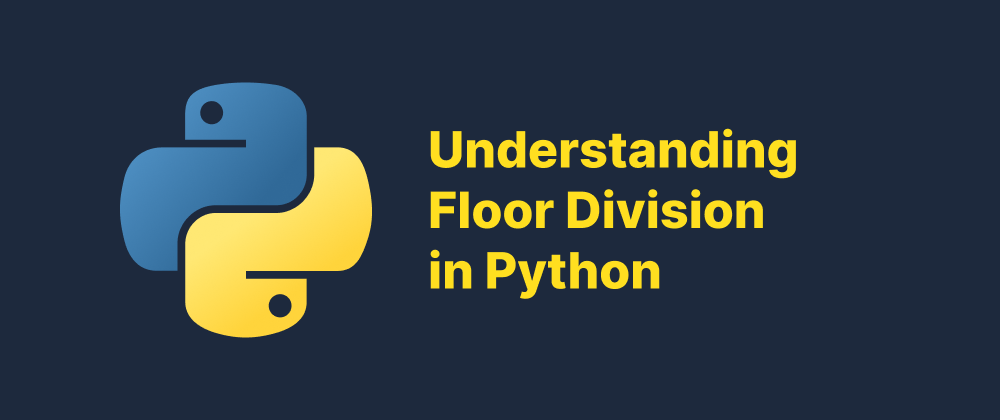
Key Takeaways
- Floor division uses
//
to return the largest integer less than or equal to the result. - It behaves differently with negative numbers compared to regular division.
- It is useful for integer-based calculations like indexing or pagination.
In Python, division can be performed in two main ways: regular division and floor division. While regular division returns a floating-point result, floor division returns the largest possible integer that is less than or equal to the result. This distinction is important for developers who need precise control over integer results in calculations.
What is Floor Division?
Floor division is performed using the double slash operator //
. It divides two numbers and truncates the result to the nearest lower integer (also known as the mathematical floor of the division). This behavior is consistent for both positive and negative numbers, although it can yield less intuitive results when dealing with negatives.
Syntax
result = a // b
Where a
and b
are numbers (integers or floats), and result
is the floored quotient.
Examples
print(7 // 2) # Output: 3 print(7 / 2) # Output: 3.5 print(-7 // 2) # Output: -4 print(-7 / 2) # Output: -3.5
As you can see:
7 // 2
gives3
because 3 is the largest integer less than or equal to 3.5.-7 // 2
gives-4
because -4 is the largest integer less than or equal to -3.5.
Use Cases
Floor division is commonly used when:
- You need to calculate index positions in arrays or lists.
- You're implementing pagination logic.
- You want to avoid floating-point numbers in mathematical models.
For example:
# Calculating page number from item index index = 17 items_per_page = 5 page_number = index // items_per_page print(page_number) # Output: 3
Floor Division with Floats
Even when both operands are floats, the //
operator still applies floor division logic:
print(7.5 // 2.0) # Output: 3.0 print(-7.5 // 2.0) # Output: -4.0
The result remains a float, but it's still floored to the nearest lower number.
Conclusion
Floor division in Python is a powerful tool when you need integer results that are always rounded down. Whether you're working with positive or negative numbers, understanding how the //
operator behaves can help you write more accurate and efficient code.
FAQs
/
returns a float result, while //
returns the floored integer result.
It rounds the result down to the nearest lower integer, which can be more negative than expected.
Yes, it works with floats and returns a floored float value.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ