How to Round Numbers in Python
Daniel Hayes
Full-Stack Engineer · Leapcell
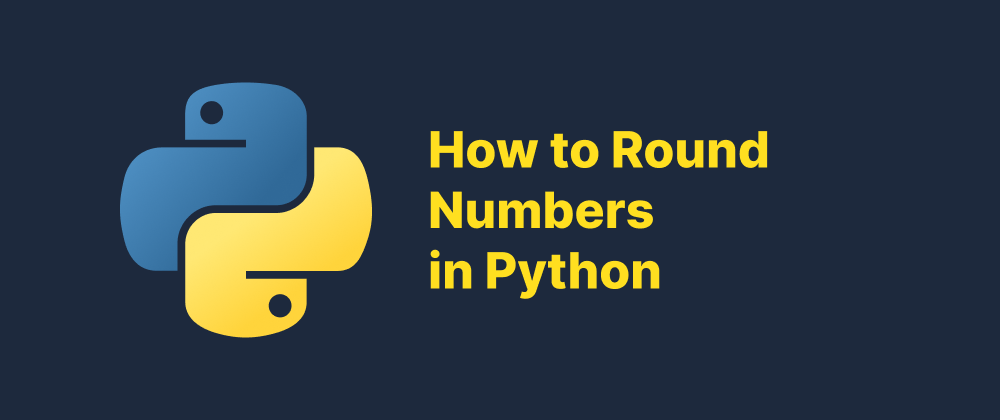
Key Takeaways
- Python’s built-in
round()
uses “round half to even” strategy. - Use
math.ceil()
ormath.floor()
for always rounding up or down. - The
decimal
module provides precise rounding for financial use cases.
Rounding numbers is a fundamental operation in programming, especially when dealing with floating-point arithmetic, financial calculations, or data presentation. Python offers several methods to round numbers, each suited for different scenarios. This article explores various rounding techniques in Python, including the built-in round()
function, the math
module, and the decimal
module.
The Built-in round()
Function
Python's built-in round()
function is the most straightforward way to round numbers.
Syntax
round(number, ndigits)
number
: The number you want to round.ndigits
(optional): The number of decimal places to round to. If omitted, the number is rounded to the nearest integer.
Examples
round(3.14159) # Output: 3 round(3.14159, 2) # Output: 3.14 round(2.5) # Output: 2 round(3.5) # Output: 4
Note: Python uses "round half to even" (also known as "bankers' rounding") as its default rounding strategy. This means that numbers exactly halfway between two integers are rounded to the nearest even integer. For example, round(2.5)
returns 2
, and round(3.5)
returns 4
Rounding Up and Down with the math
Module
For more control over rounding, especially when you need to always round up or down, Python's math
module provides ceil()
and floor()
functions.
Rounding Up with math.ceil()
import math math.ceil(3.2) # Output: 4 math.ceil(-3.2) # Output: -3
math.ceil()
returns the smallest integer greater than or equal to the given number.
Rounding Down with math.floor()
import math math.floor(3.8) # Output: 3 math.floor(-3.8) # Output: -4
math.floor()
returns the largest integer less than or equal to the given number.
Precise Rounding with the decimal
Module
When dealing with financial applications or requiring high precision, the decimal
module is preferable. It allows for more accurate decimal representation and various rounding strategies.
Example: Rounding Using decimal
from decimal import Decimal, ROUND_HALF_UP number = Decimal('2.675') rounded_number = number.quantize(Decimal('0.01'), rounding=ROUND_HALF_UP) print(rounded_number) # Output: 2.68
In this example, ROUND_HALF_UP
rounds the number away from zero if it's exactly halfway between two possibilities. This is the standard rounding method taught in schools.
Rounding Arrays with NumPy
For numerical computations involving arrays, NumPy offers efficient rounding functions.
Example: Rounding with NumPy
import numpy as np array = np.array([1.234, 5.6789, 9.8765]) rounded_array = np.round(array, 2) print(rounded_array) # Output: [1.23 5.68 9.88]
NumPy's round()
function rounds each element in the array to the specified number of decimal places.
Summary
Python provides multiple methods for rounding numbers:
- Use
round()
for basic rounding needs. - Use
math.ceil()
andmath.floor()
when you need to always round up or down. - Use the
decimal
module for precise decimal rounding, especially in financial applications. - Use NumPy's rounding functions for efficient operations on arrays.
Choosing the appropriate method depends on the specific requirements of your application, such as the desired rounding strategy and the need for precision.
FAQs
Because Python uses “round half to even” (bankers’ rounding), and 2 is the nearest even number.
Use decimal
when you need high-precision rounding, such as in financial or scientific applications.
Yes, use NumPy's round()
function to efficiently round arrays.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ