Master-Worker Pattern in NodeJS: Everything You Need to Know
James Reed
Infrastructure Engineer · Leapcell
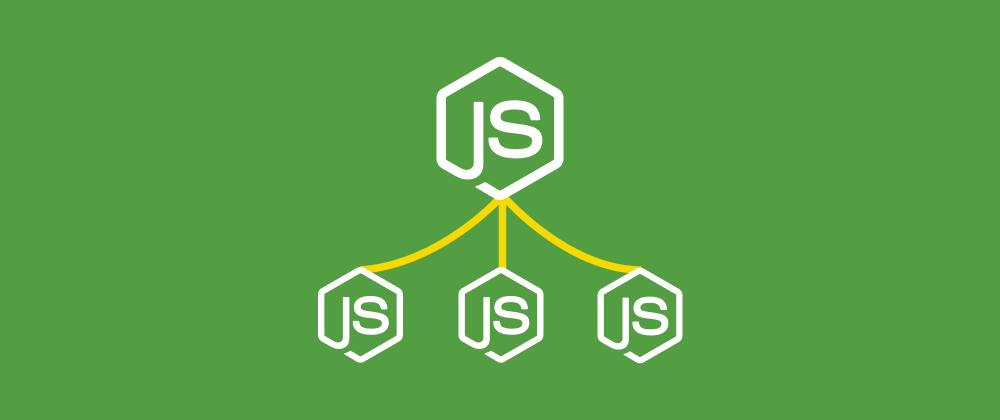
NodeJS is a development platform based on JavaScript that allows developers to write server-side applications using JavaScript. One of NodeJS's key features is its single-threaded, event-driven model, enabling efficient handling of numerous concurrent requests without requiring multiple threads or processes.
Why Use the Master-Worker Pattern
However, the single-threaded nature of NodeJS has its limitations, such as:
- CPU-Intensive Tasks: For tasks like image processing or encryption/decryption, the single thread can block other requests, leading to performance degradation.
- Error Handling: If an error or exception occurs, the single thread may crash, causing the entire application to stop running.
- Multi-Core Utilization: The single-threaded model cannot fully leverage multi-core CPUs.
To address these issues, NodeJS offers a Master-Worker Pattern, also known as the Cluster Mode. This distributed system design pattern comprises a master process and multiple worker processes. The master process manages and monitors the workers, while the workers handle specific business logic.
How to Implement the Master-Worker Pattern
In NodeJS, the Master-Worker Pattern can be implemented using the cluster
module. This module allows developers to easily create multiple child processes and provides methods and events for control and communication. The basic steps to use the cluster
module are:
- Import the
cluster
module and determine if the current process is the master or a worker. - If it’s the master, use the
cluster.fork()
method to create multiple worker processes and bind events to monitor their state and messages. - If it’s a worker, execute the specific business logic and send messages to the master process using
process.send()
.
Basic Usage
Here is a basic example of using the cluster
module:
const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { console.log(`Master ${process.pid} is running`); // Fork workers. for (let i = 0; i < numCPUs; i++) { cluster.fork(); } cluster.on('exit', (worker, code, signal) => { console.log(`Worker ${worker.process.pid} died`); }); } else { // Workers can share any TCP connection. // In this case, it is an HTTP server. http .createServer((req, res) => { res.writeHead(200); res.end('Hello from Worker!'); }) .listen(8000); console.log(`Worker ${process.pid} started`); }
In this code, the master process first checks if it is the master (cluster.isMaster
). If true, it creates as many workers as the system's CPU core count. Each worker is an independent instance of the master process, with its own memory and V8 instance. The workers create an HTTP server using http.createServer
and begin listening for requests.
If a worker crashes or is killed, the exit
event triggers, allowing the master to restart the worker.
Advanced Usage: Integrating with Nginx Load Balancing
Use Nginx as a reverse proxy and load balancer. Nginx can distribute incoming requests across multiple Node.js processes.
Nginx configuration example:
http { upstream node_cluster { server 127.0.0.1:8000; server 127.0.0.1:8001; server 127.0.0.1:8002; # ... other Node.js processes } server { listen 80; location / { proxy_pass http://node_cluster; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } } }
In this configuration, Nginx distributes incoming requests evenly among the Node.js processes defined in node_cluster
.
Benefits of Using the Master-Worker Pattern
- Load Balancing: Running multiple Node.js processes on a single server ensures incoming requests are evenly distributed, increasing throughput.
- Fault Tolerance and High Availability: If a worker crashes, the master can restart it without affecting other workers.
- CPU-Intensive Tasks: Multiple workers can handle CPU-intensive tasks, preventing the main thread from being blocked.
Considerations
- State Sharing: Each worker process is independent and does not share memory. If your application requires state sharing (e.g., session state), you need to use external services or databases.
In conclusion, Node.js's Master-Worker Pattern, implemented via the cluster
module, offers a simple yet powerful way to create multi-process applications, enhancing performance and reliability.
We are Leapcell, your top choice for hosting Node.js projects
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ