Advanced Python Concurrency: Multithreading and AsyncIO
Grace Collins
Solutions Engineer ยท Leapcell
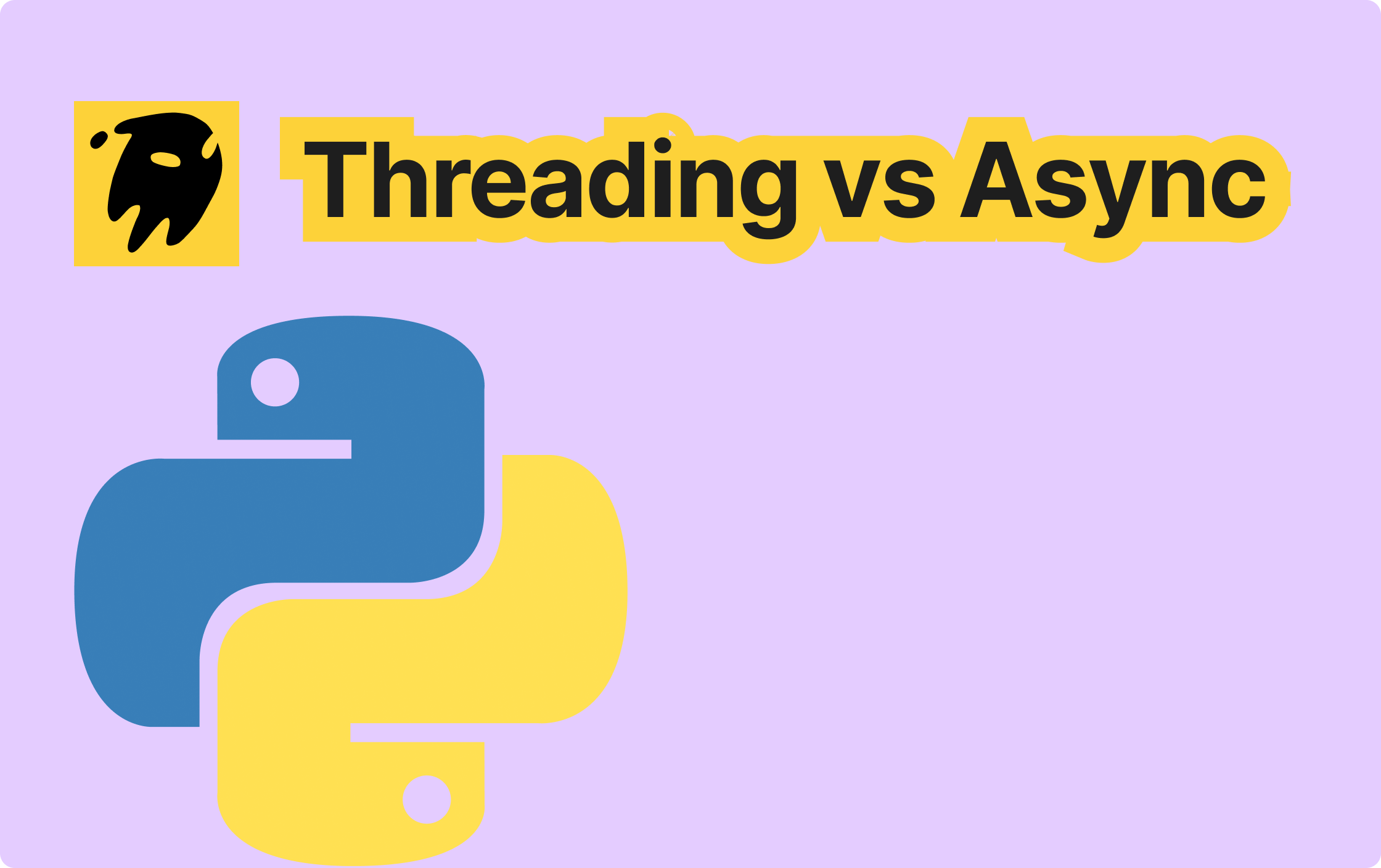
Exploration of Python Concurrent Programming
In Python programming, multithreading is a commonly used means of concurrent programming, which can effectively improve the execution efficiency of the program, especially when dealing with I/O-intensive tasks. Python makes multithreading programming relatively easy with the help of the threading
module. This article will delve into the basic knowledge of the threading
module and demonstrate the application of multithreading through examples.
1. Basic Concepts of Multithreading
Before starting, let's first understand some basic concepts of multithreading programming:
- Thread: It is the smallest unit for the operating system to perform operation scheduling, usually existing inside a process.
- Multithreading: It refers to running multiple threads simultaneously in the same program.
- GIL (Global Interpreter Lock): This is the global interpreter lock of the Python interpreter, which restricts that only one thread can execute Python bytecode at the same time. Therefore, in CPU-intensive tasks, multithreading cannot make full use of multi-core processors.
2. Basics of the threading Module
The threading
module provides tools for creating and managing threads. Here are some commonly used classes and functions in the threading
module:
- Thread class: A class used to create threads. Define the execution logic of the thread by inheriting the
Thread
class and implementing therun
method. - start() method: Starts the thread.
- join() method: Waits for the thread to finish executing.
- active_count() function: Gets the number of currently active threads.
3. Code Practice: Multithreaded Image Downloading
The following demonstrates the application of multithreading through an example. We will use multithreading to download a series of images.
import threading import requests from queue import Queue class LeapCellImageDownloader: def __init__(self, urls): self.urls = urls self.queue = Queue() def download_image(self, url): response = requests.get(url) if response.status_code == 200: filename = url.split("/")[-1] with open(filename, "wb") as f: f.write(response.content) print(f"Downloaded: {filename}") def worker(self): while True: url = self.queue.get() if url is None: break self.download_image(url) self.queue.task_done() def start_threads(self, num_threads=5): threads = [] for _ in range(num_threads): thread = threading.Thread(target=self.worker) thread.start() threads.append(thread) for url in self.urls: self.queue.put(url) self.queue.join() for _ in range(num_threads): self.queue.put(None) for thread in threads: thread.join() if __name__ == "__main__": image_urls = ["url1", "url2", "url3"] # Replace with the actual image URLs downloader = LeapCellImageDownloader(image_urls) downloader.start_threads()
In this example, we created a LeapCellImageDownloader
class, which contains a worker
method for downloading images. Through multithreading, we can download multiple images in parallel, improving the download efficiency.
4. Code Analysis
- download_image method: Responsible for the specific implementation of image downloading.
- worker method: As the execution logic of the thread, it continuously takes the image URL to be downloaded from the queue and calls the
download_image
method. - start_threads method: Starts a specified number of threads, puts the image URLs into the queue, and waits for all threads to finish executing.
6. Thread Safety and Lock Mechanism
In multithreading programming, since multiple threads access shared resources simultaneously, a race condition may occur. To avoid this situation, a lock mechanism can be used to ensure that only one thread can access the shared resource at a certain moment.
The threading
module provides the Lock
class. Through it, a lock can be created. Use the acquire
method to obtain the lock and the release
method to release the lock. Here is a simple example:
import threading leapcell_counter = 0 leapcell_counter_lock = threading.Lock() def increment_counter(): global leapcell_counter for _ in range(1000000): with leapcell_counter_lock: leapcell_counter += 1 def main(): thread1 = threading.Thread(target=increment_counter) thread2 = threading.Thread(target=increment_counter) thread1.start() thread2.start() thread1.join() thread2.join() print("LeapCell Counter:", leapcell_counter) if __name__ == "__main__": main()
In this example, we created a global variable leapcell_counter
and used a lock to ensure that there will be no race condition when the two threads modify leapcell_counter
simultaneously.
7. Applicable Scenarios of Multithreading
Multithreading is suitable for handling I/O-intensive tasks, such as network requests, file reading and writing, etc. In these scenarios, the thread can yield the CPU while waiting for I/O, allowing other threads to have the opportunity to execute and improving the overall efficiency of the program.
However, when dealing with CPU-intensive tasks, due to Python's GIL, multithreading cannot make full use of multi-core processors, which may lead to performance bottlenecks. For CPU-intensive tasks, consider using multiprocessing programming or other concurrent models.
9. Exception Handling and Multithreading
In multithreading programming, exception handling may become more complex. Since each thread has its own execution context, an exception may be raised in one thread but caught in another thread. To handle exceptions effectively, we need to use appropriate exception handling mechanisms in each thread.
import threading def leapcell_thread_function(): try: # Some operations that may raise an exception result = 10 / 0 except ZeroDivisionError as e: print(f"Exception in LeapCell thread: {e}") if __name__ == "__main__": thread = threading.Thread(target=leapcell_thread_function) thread.start() thread.join() print("Main thread continues...")
In this example, the division operation in the thread leapcell_thread_function
may raise a ZeroDivisionError
exception. To catch and handle this exception, we used a try-except
statement in the thread's code block.
10. Precautions for Multithreading
When doing multithreading programming, there are some common precautions that need special attention:
- Thread safety: Ensure that data races and inconsistencies will not occur when multiple threads access shared resources simultaneously.
- Deadlock: Deadlock may occur when multiple threads wait for each other to release the lock, and careful design and use of locks are required.
- GIL limitations: Python's global interpreter lock may limit the performance improvement of multithreading in CPU-intensive tasks.
- Exception handling: Exceptions need to be properly handled in each thread to prevent an exception from being raised in one thread but not caught in other threads.
11. Performance Optimization of Multithreading
In some cases, we can optimize the performance of multithreaded programs through some techniques:
- Thread pool: Use
ThreadPoolExecutor
in theconcurrent.futures
module to create a thread pool and improve the reusability of threads. - Queue: Use a queue to coordinate the work between multiple threads and implement the producer-consumer model.
- Avoid GIL limitations: For CPU-intensive tasks, consider using other concurrent models such as multiprocessing and
asyncio
.
13. Object-Oriented Multithreading Design
In practical applications, we usually face more complex problems and need to combine multithreading with object-oriented design. The following is a simple example demonstrating how to design a multithreaded program in an object-oriented way:
import threading import time class LeapCellWorkerThread(threading.Thread): def __init__(self, name, delay): super().__init__() self.name = name self.delay = delay def run(self): print(f"{self.name} started.") time.sleep(self.delay) print(f"{self.name} completed.") if __name__ == "__main__": thread1 = LeapCellWorkerThread("LeapCell Thread 1", 2) thread2 = LeapCellWorkerThread("LeapCell Thread 2", 1) thread1.start() thread2.start() thread1.join() thread2.join() print("Main thread continues...")
In this example, we created a LeapCellWorkerThread
class, which inherits from the Thread
class and overrides the run
method to define the execution logic of the thread. Each thread is given a name and a delay time.
14. Multithreading and Resource Manager
Consider a scenario where we need to create a resource manager responsible for managing the allocation and release of a certain resource. At this time, we can use multithreading to achieve asynchronous management of resources. The following is an example of a simple resource manager:
import threading import time class LeapCellResourceManager: def __init__(self, total_resources): self.total_resources = total_resources self.available_resources = total_resources self.lock = threading.Lock() def allocate(self, request): with self.lock: if self.available_resources >= request: print(f"Allocated {request} LeapCell resources.") self.available_resources -= request else: print("Insufficient LeapCell resources.") def release(self, release): with self.lock: self.available_resources += release print(f"Released {release} LeapCell resources.") class LeapCellUserThread(threading.Thread): def __init__(self, name, resource_manager, request, release): super().__init__() self.name = name self.resource_manager = resource_manager self.request = request self.release = release def run(self): print(f"{self.name} started.") self.resource_manager.allocate(self.request) time.sleep(1) # Simulate some work with allocated resources self.resource_manager.release(self.release) print(f"{self.name} completed.") if __name__ == "__main__": manager = LeapCellResourceManager(total_resources=5) user1 = LeapCellUserThread("LeapCell User 1", manager, request=3, release=2) user2 = LeapCellUserThread("LeapCell User 2", manager, request=2, release=1) user1.start() user2.start() user1.join() user2.join() print("Main thread continues...")
In this example, the LeapCellResourceManager
class is responsible for managing the allocation and release of resources, and the LeapCellUserThread
class represents a user thread that uses resources. By using a lock, the safe allocation and release of resources are ensured.
16. Debugging and Performance Analysis of Multithreading
When doing multithreading programming, debugging and performance analysis are important aspects that cannot be ignored. Python provides some tools and techniques to help us better understand and debug multithreaded programs.
Debugging Multithreaded Programs
- Using print statements: Insert
print
statements at appropriate positions to output key information to help track the execution flow of the program. - Logging module: Use Python's
logging
module to record information during program runtime, including the start, end, and key operations of threads. - pdb debugger: Insert breakpoints in the code and use Python's built-in debugger
pdb
for interactive debugging.
import pdb # Insert a breakpoint in the code pdb.set_trace()
Performance Analysis of Multithreaded Programs
- Using the timeit module: By embedding timing code in the code, use the
timeit
module to measure the execution time of specific operations or functions.
import timeit def my_function(): # The code to be tested # Test the execution time of the function execution_time = timeit.timeit(my_function, number=1) print(f"Execution time: {execution_time} seconds")
- Using the cProfile module:
cProfile
is Python's performance analysis tool, which can help view function calls and execution times.
import cProfile def my_function(): # The code to be tested # Run the performance analysis cProfile.run("my_function()")
- Using third-party tools: Some third-party tools, such as
line_profiler
,memory_profiler
, etc., can provide more detailed performance analysis information to help find performance bottlenecks.
# Install line_profiler pip install line_profiler # Use line_profiler for performance analysis kernprof -l script.py python -m line_profiler script.py.lprof
17. Safety and Risks of Multithreading
Although multithreading programming can improve program performance, it also brings some potential security issues. The following are some aspects that need attention:
- Thread safety: Ensure that the access to shared resources is thread-safe, which can be controlled by means of lock mechanisms, atomic operations, etc.
- Deadlock: When using locks, be careful of the occurrence of deadlock, that is, multiple threads wait for each other to release resources, resulting in the program being unable to continue execution.
- Resource leakage: In multithreading programming, it is easy to have the situation where resources are not properly released, such as threads not being properly closed or locks not being properly released.
- GIL limitations: In CPU-intensive tasks, the global interpreter lock (GIL) may become a performance bottleneck, and careful selection of multithreading or other concurrent models is required.
18. Exploring Other Concurrent Models
Although multithreading is a commonly used concurrent programming model, it is not the only choice. Python also provides some other concurrent models, including:
- Multiprocessing programming: Implemented through the
multiprocessing
module. Each process has an independent interpreter and GIL, which is suitable for CPU-intensive tasks. - Asynchronous programming: Implemented through the
asyncio
module, based on the event loop and coroutines, suitable for I/O-intensive tasks, which can improve the concurrency of the program. - Parallel computing: Use
ProcessPoolExecutor
andThreadPoolExecutor
in theconcurrent.futures
module to execute tasks in parallel.
19. Continuous Learning and Practice
Multithreading programming is a vast and complex field, and this article only provides you with an introductory guide. Continuous learning and practice are the keys to mastering multithreading programming in depth.
It is recommended to read the Python official documentation and related books to deeply understand the various features and usage of the threading
module. Participating in open-source projects and reading other people's source code are also good ways to improve your skills.
21. Asynchronousization of Multithreading and Coroutines
In modern programming, asynchronous programming and coroutines have become important tools for handling high-concurrency scenarios. Python provides the asyncio
module to implement asynchronous programming through coroutines. Compared with traditional multithreading, asynchronous programming can more efficiently handle a large number of I/O-intensive tasks without creating a large number of threads.
Basics of Asynchronous Programming
Asynchronous programming uses the async
and await
keywords to define coroutines. A coroutine is a lightweight thread that can be paused and resumed during runtime.
import asyncio async def leapcell_my_coroutine(): print("Start LeapCell coroutine") await asyncio.sleep(1) print("LeapCell Coroutine completed") async def leapcell_main(): await asyncio.gather(leapcell_my_coroutine(), leapcell_my_coroutine()) if __name__ == "__main__": asyncio.run(leapcell_main())
In the above example, leapcell_my_coroutine
is a coroutine, and asyncio.sleep
is used to simulate an asynchronous operation. Multiple coroutines are run simultaneously through asyncio.gather
.
Comparison between Asynchronous and Multithreading
- Performance: Asynchronous programming can more efficiently handle a large number of I/O-intensive tasks compared to multithreading, because asynchronous tasks can yield control when waiting for I/O without blocking the execution of other tasks.
- Complexity: Asynchronous programming may be more difficult to write and understand than multithreading, and requires familiarity with the concepts of coroutines and the asynchronous programming model.
Example: Asynchronous Image Downloading
The following is a simple example of using asynchronous programming to implement image downloading:
import asyncio import aiohttp async def leapcell_download_image(session, url): async with session.get(url) as response: if response.status == 200: filename = url.split("/")[-1] with open(filename, "wb") as f: f.write(await response.read()) print(f"LeapCell Downloaded: {filename}") async def leapcell_main(): image_urls = ["url1", "url2", "url3"] # Replace with the actual image URLs async with aiohttp.ClientSession() as session: tasks = [leapcell_download_image(session, url) for url in image_urls] await asyncio.gather(*tasks) if __name__ == "__main__": asyncio.run(leapcell_main())
In this example, asynchronous HTTP requests are created through the aiohttp
library, and multiple coroutines are executed concurrently through asyncio.gather
.
22. Exception Handling in Asynchronous Programming
In asynchronous programming, the way of handling exceptions is also different. In a coroutine, we usually use a try-except
block or methods like asyncio.ensure_future
to handle exceptions.
import asyncio async def leapcell_my_coroutine(): try: # Asynchronous operation await asyncio.sleep(1) raise ValueError("An error occurred") except ValueError as e: print(f"LeapCell Caught an exception: {e}") async def leapcell_main(): task = asyncio.ensure_future(leapcell_my_coroutine()) await asyncio.gather(task) if __name__ == "__main__": asyncio.run(leapcell_main())
In this example, asyncio.ensure_future
wraps the coroutine into a Task
object. By using await asyncio.gather
to wait for the task to complete, the exception is caught.
23. Advantages and Precautions of Asynchronous Programming
Advantages
- High Concurrency: Asynchronous programming is suitable for a large number of I/O-intensive tasks. It can handle concurrent requests more efficiently and improve the throughput of the system.
- Resource Efficiency: Compared with multithreading, asynchronous programming usually saves more resources because coroutines are lightweight and multiple coroutines can run in a single thread.
Precautions
- Blocking Operations: In asynchronous programming, blocking operations will affect the entire event loop, and blocking calls should be avoided as much as possible.
- Exception Handling: Exception handling in asynchronous programming may be more complex, and the exception situations in coroutines need to be carefully handled.
- Applicable Scenarios: Asynchronous programming is more suitable for I/O-intensive tasks rather than CPU-intensive tasks.
24. Exploring More Asynchronous Programming Tools and Libraries
In addition to asyncio
and aiohttp
, there are some other powerful asynchronous programming tools and libraries:
- asyncpg: An asynchronous PostgreSQL database driver.
- aiofiles: An asynchronous file operation library.
- aiohttp: An asynchronous HTTP client and server framework.
- aiomysql: An asynchronous MySQL database driver.
- uvloop: A high-performance event loop used to replace the standard event loop.
25. Continuous Learning and Practice
Asynchronous programming is a broad and in-depth topic, and this article only provides you with a brief introduction. It is recommended to study the documentation of the asyncio
module in depth to understand concepts such as the event loop, coroutines, and asynchronous operations.
At the same time, through practical projects, you will better understand and master the techniques and best practices of asynchronous programming.
Conclusion
This article has deeply explored multithreading programming and asynchronous programming in Python, covering the basic knowledge of the multithreading module (threading
), code practice, as well as the basic concepts and usage of the asynchronous programming module (asyncio
). We started from the basics of multithreading, such as the Thread
class, lock mechanism, thread safety, etc., and gradually demonstrated the application scenarios and precautions of multithreading in practical applications. Through an example, the process of multithreaded image downloading was shown, emphasizing the importance of thread safety and exception handling.
Leapcell: The Best of Serverless Web Hosting
Finally, I would like to recommend a platform that is most suitable for deploying Python services: Leapcell
๐ Build with Your Favorite Language
Develop effortlessly in JavaScript, Python, Go, or Rust.
๐ Deploy Unlimited Projects for Free
Only pay for what you useโno requests, no charges.
โก Pay-as-You-Go, No Hidden Costs
No idle fees, just seamless scalability.
๐ Explore Our Documentation
๐น Follow us on Twitter: @LeapcellHQ