How to Use Python `requests` for POST Requests
Daniel Hayes
Full-Stack Engineer · Leapcell
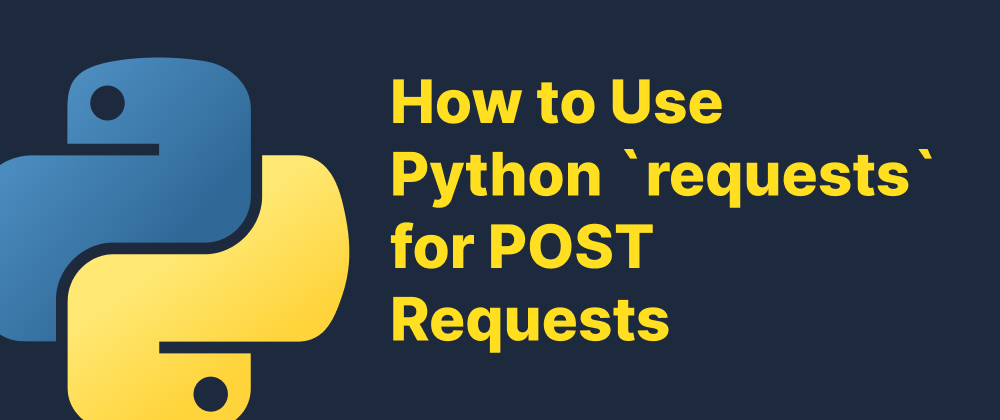
Key Takeaways
- The
requests.post()
method simplifies sending form and JSON data. - You can easily upload files and set custom headers.
- Handling the response object properly is crucial for robust applications.
Python's requests
library is a powerful tool for making HTTP requests, including sending data to servers using the POST method. This article provides an overview of how to use the requests
library to perform POST requests, covering various data formats and configurations.
Installing the requests
Library
Before using the requests
library, ensure it is installed in your Python environment. You can install it using pip:
pip install requests
Making a Basic POST Request
To send a POST request, use the requests.post()
method, specifying the target URL and the data to be sent. By default, the data is sent with a Content-Type
header of application/x-www-form-urlencoded
.
import requests url = 'https://httpbin.org/post' data = {'key': 'value'} response = requests.post(url, data=data) print(response.text)
In this example, the dictionary data
is form-encoded and sent in the body of the POST request. The server's response is then printed.
Sending JSON Data
When interacting with APIs that expect JSON-formatted data, you can use the json
parameter in the post()
method. This approach automatically encodes the data as JSON and sets the Content-Type
header to application/json
.
import requests url = 'https://httpbin.org/post' json_data = {'key': 'value'} response = requests.post(url, json=json_data) print(response.text)
This method simplifies the process of sending JSON data compared to manually encoding the data and setting headers.
Uploading Files
The requests
library also supports file uploads using the files
parameter. To upload a file, pass a dictionary with the filename as the key and a file object as the value.
import requests url = 'https://httpbin.org/post' files = {'file': open('example.txt', 'rb')} response = requests.post(url, files=files) print(response.text)
This sends the file as a multipart/form-data request.
Setting Custom Headers
To include custom headers in your POST request, use the headers
parameter. This is useful for setting headers like Authorization
or custom Content-Type
.
import requests url = 'https://httpbin.org/post' data = {'key': 'value'} headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'} response = requests.post(url, data=data, headers=headers) print(response.text)
Ensure that the headers align with the server's expectations to avoid issues.
Handling Response
The response from a POST request is a Response
object containing useful attributes and methods:
response.status_code
: HTTP status code of the response.response.text
: Content of the response, in Unicode.response.json()
: Parses the response as JSON and returns the result.
import requests url = 'https://httpbin.org/post' data = {'key': 'value'} response = requests.post(url, data=data) if response.status_code == 200: print('Success!') print(response.json()) else: print('An error occurred.')
Properly handling the response ensures that your application can react appropriately to different outcomes.
Conclusion
The requests
library in Python simplifies the process of making HTTP POST requests. Whether you're sending form data, JSON, or files, requests
provides a straightforward and flexible API to handle these tasks efficiently.
FAQs
Use the json=
parameter in requests.post()
instead of data=
.
Yes, by passing a file object with the files
parameter.
Include it in the headers
dictionary and pass it to the post()
call.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ