Boosting Go Testing Efficiency with Mockery
Daniel Hayes
Full-Stack Engineer · Leapcell
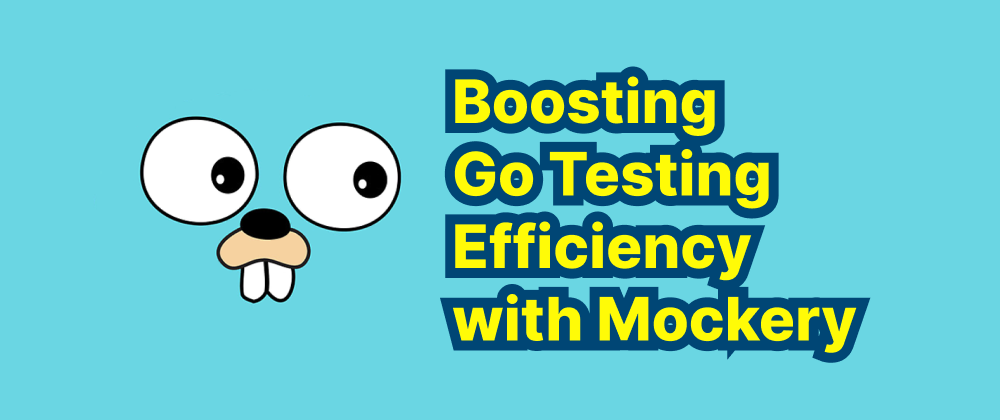
Key Takeaways
- Mockery automates mock generation for Go interfaces, reducing manual effort and improving testing efficiency.
- It integrates seamlessly with Testify, allowing for flexible and reliable unit testing.
- Mockery’s CLI simplifies mock generation, making it easy to use in CI/CD pipelines.
Mockery is a powerful and user-friendly code generation tool designed for Go (Golang) developers to create mock objects for testing purposes. By automating the generation of mock implementations for Go interfaces, Mockery streamlines the testing process, reduces boilerplate code, and enhances overall code quality.
Key Features of Mockery:
-
Automated Mock Generation: Mockery analyzes Go interfaces and automatically generates mock implementations, saving developers time and effort in writing mocks manually.
-
Integration with Testify: The tool seamlessly integrates with the popular stretchr/testify testing framework, ensuring compatibility and ease of use in existing test suites.
-
Customization Options: Developers can customize the generated mocks to fit specific testing requirements, allowing for flexible and tailored mock behaviors.
-
Command-Line Interface (CLI): Mockery offers a straightforward CLI, enabling developers to generate mocks with simple commands, which can be easily integrated into build scripts or CI/CD pipelines.
Getting Started with Mockery:
-
Installation: Install Mockery using Go's package manager:
go install github.com/vektra/mockery/v2@latest
-
Generating Mocks: Navigate to your Go project directory and run Mockery to generate mocks for your interfaces:
mockery --all
This command generates mock implementations for all interfaces in your project.
-
Using Generated Mocks in Tests: Import the generated mocks into your test files and use them to simulate behaviors and assert expectations.
import ( "testing" "github.com/stretchr/testify/assert" "github.com/your_project/mocks" ) func TestExample(t *testing.T) { mockService := new(mocks.YourInterface) // Set up expectations mockService.On("YourMethod", "input").Return("output", nil) // Use mockService in your tests result, err := mockService.YourMethod("input") assert.NoError(t, err) assert.Equal(t, "output", result) // Assert that the expectations were met mockService.AssertExpectations(t) }
By incorporating Mockery into your Go development workflow, you can enhance testing efficiency, maintain cleaner codebases, and ensure robust application performance.
For more information and advanced usage, refer to the Mockery GitHub repository.
FAQs
It auto-generates mock implementations of interfaces, reducing boilerplate code and improving test coverage.
Yes, developers can customize mock behaviors to fit specific test scenarios.
Yes, its CLI makes it easy to integrate into automated build and test workflows.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ