Checking If a Key Exists in a Go Map
James Reed
Infrastructure Engineer · Leapcell
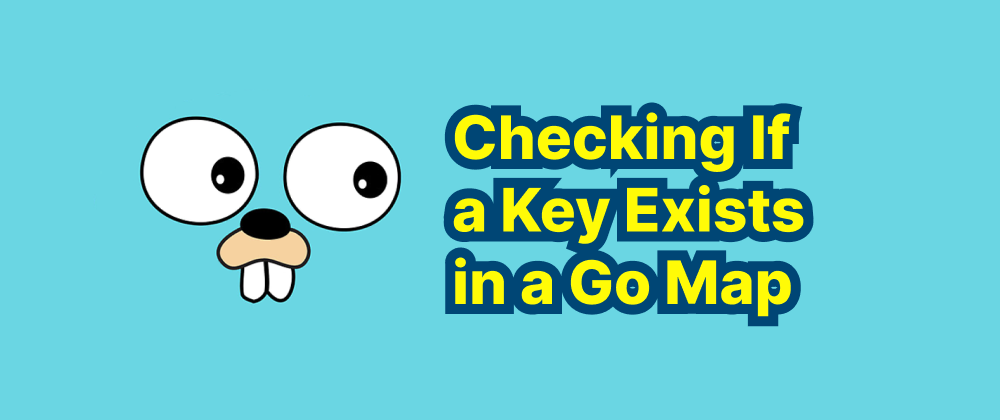
Key Takeaways
- Use the "comma ok" idiom (
value, ok := myMap[key]
) to check for key existence. - If only existence matters, use
_
to ignore the value (_, ok := myMap[key]
). - This method ensures efficient and readable code when working with Go maps.
In Go, maps are essential data structures that store key-value pairs, allowing for efficient data retrieval. A common requirement when working with maps is to determine whether a specific key exists. Go provides a concise and efficient way to perform this check using the "comma ok" idiom.
Checking for Key Existence in a Map
To verify if a key is present in a map, you can utilize the following syntax:
value, ok := myMap[key]
In this expression:
-
value
is assigned the value associated withkey
if it exists; otherwise, it receives the zero value for the map's value type. -
ok
is a boolean that istrue
ifkey
is found in the map, andfalse
if it is not.
This approach allows you to distinguish between a key that doesn't exist and a key whose value is the zero value of the map's value type.
Practical Example
Consider the following example demonstrating how to check for a key's existence in a map:
package main import "fmt" func main() { // Initialize a map with string keys and int values myMap := map[string]int{ "apple": 2, "banana": 5, "cherry": 7, } // Key to check key := "banana" // Check if the key exists in the map if value, ok := myMap[key]; ok { fmt.Printf("Key '%s' exists with value %d.\n", key, value) } else { fmt.Printf("Key '%s' does not exist.\n", key) } }
Output:
Key 'banana' exists with value 5.
In this example, the program checks for the existence of the key "banana"
in myMap
. Since "banana"
is present, it prints the corresponding value.
Using the Blank Identifier
If you are only interested in whether a key exists and do not need its associated value, you can use the blank identifier (_
) to ignore the value:
if _, ok := myMap[key]; ok { // Key exists // Perform necessary actions } else { // Key does not exist // Handle the absence accordingly }
This method is efficient when the value associated with the key is not required for subsequent operations.
Conclusion
Checking for the existence of a key in a Go map is straightforward using the "comma ok" idiom. This technique enhances code readability and efficiency by allowing developers to handle the presence or absence of keys gracefully. By leveraging this feature, you can write robust Go programs that effectively manage map data structures.
FAQs
It is true
if the key exists, otherwise false
.
The value
is assigned the zero value of the map's value type.
Yes, use _
in place of value
: _, ok := myMap[key]
.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ