Understanding Go Timers: Timer and Ticker in Action
Daniel Hayes
Full-Stack Engineer · Leapcell
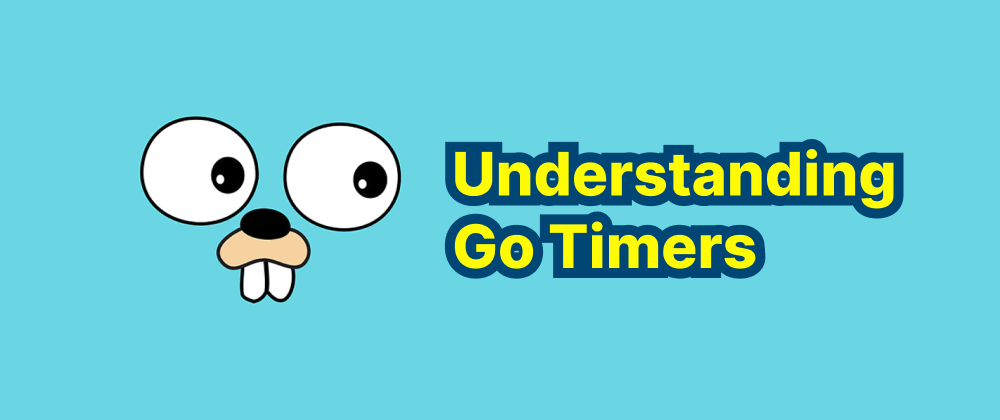
Key Takeaways
- Go's
Timer
is used for single-event delays, whileTicker
is for repeated executions. - Timers and tickers must be stopped when no longer needed to avoid resource leaks.
- Proper handling of timer channels prevents goroutine leaks in concurrent applications.
In Go, timers are essential for managing operations that require execution after a specific duration or at regular intervals. The time
package offers two primary types: Timer
and Ticker
.
Timer
A Timer
represents a single event that sends the current time on its channel after a specified duration.
package main import ( "fmt" "time" ) func main() { timer := time.NewTimer(2 * time.Second) <-timer.C fmt.Println("Timer expired") }
In this example, a Timer
is set for 2 seconds. The program waits to receive from the C
channel, unblocking when the timer expires.
To stop a timer before it expires, use the Stop
method:
if timer.Stop() { fmt.Println("Timer stopped") }
Ticker
A Ticker
delivers the current time on its channel at regular intervals.
package main import ( "fmt" "time" ) func main() { ticker := time.NewTicker(1 * time.Second) done := make(chan bool) go func() { time.Sleep(5 * time.Second) done <- true }() for { select { case t := <-ticker.C: fmt.Println("Tick at", t) case <-done: ticker.Stop() fmt.Println("Ticker stopped") return } } }
Here, the Ticker
ticks every second. After 5 seconds, the done
channel signals to stop the ticker.
Underlying Implementation
Both Timer
and Ticker
utilize Go's runtime timer
structure, which manages timing events efficiently. The runtimeTimer
struct includes fields like when
(the time the event should fire) and period
(for recurring events). Functions such as startTimer
, stopTimer
, and resetTimer
control these timers. For a deeper understanding, refer to the Go source code and related discussions.
Best Practices
-
Resource Management: Always stop timers and tickers when they're no longer needed to free resources.
-
Channel Operations: Be cautious with channel operations to prevent goroutine leaks. Ensure that goroutines listening to timer channels can exit appropriately.
By effectively utilizing Timer
and Ticker
, Go developers can handle time-dependent operations with precision and efficiency.
FAQs
Timer
fires once after a delay, while Ticker
repeats at fixed intervals.
Use Stop()
to halt execution and release resources.
Improper handling can lead to blocked goroutines and memory leaks.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ