How to Determine if a String Starts with a Substring in Go
Grace Collins
Solutions Engineer · Leapcell
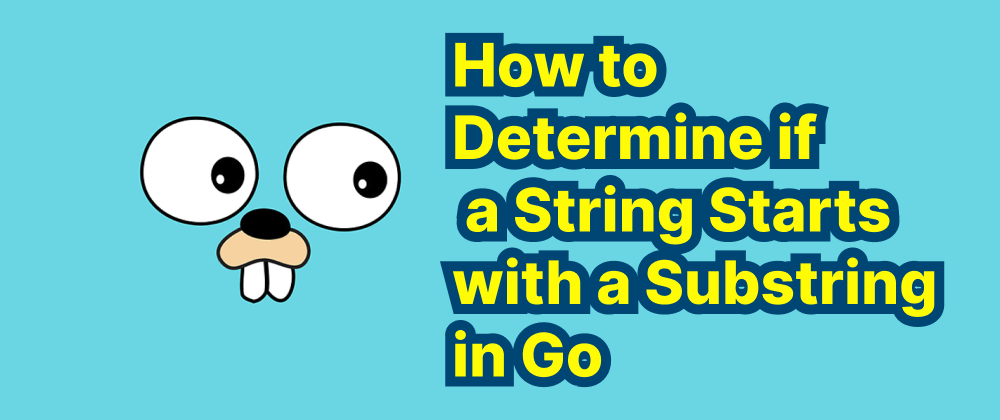
Key Takeaways
- Use
strings.HasPrefix
to check if a string starts with a specific substring efficiently. - An alternative method is slicing and comparing the string manually.
strings.HasPrefix
is the recommended approach for readability and reliability.
In Go, determining whether a string begins with a specific substring can be efficiently achieved using the strings
package, which offers a variety of functions for string manipulation. This article explores the primary method to check for a prefix in a string, along with an alternative approach using slicing.
Using strings.HasPrefix
Function
The strings
package provides the HasPrefix
function, which is specifically designed to check if a string starts with a given prefix. This function returns a boolean value: true
if the string starts with the specified prefix, and false
otherwise.
Syntax:
func HasPrefix(s, prefix string) bool
Parameters:
s
: The string to be checked.prefix
: The substring to look for at the beginning ofs
.
Example:
package main import ( "fmt" "strings" ) func main() { str := "Hello, Gophers!" prefix := "Hello" if strings.HasPrefix(str, prefix) { fmt.Printf("The string \"%s\" starts with the prefix \"%s\".\n", str, prefix) } else { fmt.Printf("The string \"%s\" does not start with the prefix \"%s\".\n", str, prefix) } }
Output:
The string "Hello, Gophers!" starts with the prefix "Hello".
In this example, strings.HasPrefix
checks if the variable str
starts with the substring prefix
. Since it does, the program outputs a confirmation message.
Alternative Approach: Using String Slicing
Another method to determine if a string starts with a specific substring is by using string slicing and comparison. This approach involves extracting a portion of the string that matches the length of the prefix and then comparing it to the desired prefix.
Example:
package main import ( "fmt" ) func main() { str := "Hello, Gophers!" prefix := "Hello" if len(str) >= len(prefix) && str[:len(prefix)] == prefix { fmt.Printf("The string \"%s\" starts with the prefix \"%s\".\n", str, prefix) } else { fmt.Printf("The string \"%s\" does not start with the prefix \"%s\".\n", str, prefix) } }
Output:
The string "Hello, Gophers!" starts with the prefix "Hello".
In this code, we first ensure that the length of str
is at least as long as prefix
to avoid slicing beyond the string's bounds. Then, we compare the initial segment of str
(up to the length of prefix
) with prefix
. If they are equal, it confirms that str
starts with prefix
.
Conclusion
To check if a string starts with a particular substring in Go, the most straightforward and idiomatic method is to use the strings.HasPrefix
function from the standard library. This function is optimized for such operations and enhances code readability. Alternatively, string slicing can be employed for this purpose, but it requires additional checks to prevent potential runtime errors.
FAQs
Using strings.HasPrefix
, as it is optimized and built into the standard library.
Yes, but you must ensure the string length is sufficient to avoid runtime errors.
Yes, it performs a case-sensitive comparison.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ