Understanding Floating-Point Numbers in Go
James Reed
Infrastructure Engineer · Leapcell
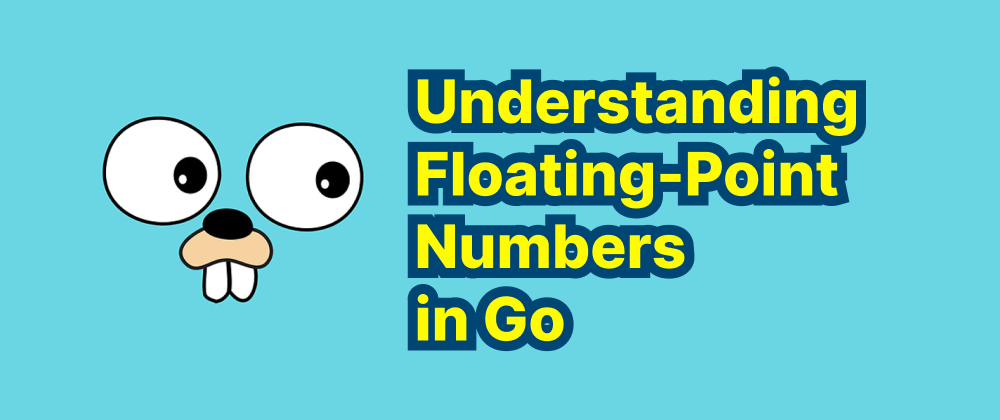
Key Takeaways
- Go provides
float32
andfloat64
, withfloat64
being preferred for precision. - Floating-point arithmetic can introduce precision errors due to binary representation.
- Use precise libraries like
decimal
for critical calculations requiring exact values.
Floating-point numbers are essential in programming for representing real numbers, especially those with fractional parts. In Go, understanding how these numbers are represented and manipulated is crucial for accurate and efficient computations.
Floating-Point Types in Go
Go provides two primary floating-point types: float32
and float64
. These types follow the IEEE-754 standard for floating-point arithmetic, which is widely supported by modern CPUs. The float32
type, also known as single-precision, occupies 4 bytes and offers approximately 6 decimal digits of precision. On the other hand, float64
, or double-precision, occupies 8 bytes and provides about 15 decimal digits of precision.
The range of values these types can represent is vast. For instance, the maximum value for float32
is approximately 3.4e38, while for float64
, it's around 1.8e308. These ranges enable Go to handle both very large and very small numbers effectively.
Precision and Representation
While floating-point numbers can represent a wide range of values, they come with limitations in precision. For example, not all decimal fractions can be represented exactly in binary form, leading to potential precision issues. A classic example is the number 0.1, which cannot be represented precisely in binary, resulting in small representation errors.
Consider the following Go code:
package main import "fmt" func main() { a := 0.1 b := 0.2 c := a + b fmt.Println(c) // Expected 0.3, but outputs 0.30000000000000004 }
In this example, adding 0.1 and 0.2 does not yield the exact result of 0.3 due to the inherent limitations of binary floating-point representation.
Handling Precision Issues
To mitigate precision issues, especially in financial calculations where accuracy is paramount, developers can use the decimal
package. This package provides arbitrary-precision fixed-point decimal numbers, allowing for precise representation and manipulation of decimal numbers without the pitfalls of binary floating-point arithmetic.
Here's how you can use the decimal
package:
package main import ( "fmt" "github.com/shopspring/decimal" ) func main() { a := decimal.NewFromFloat(0.1) b := decimal.NewFromFloat(0.2) c := a.Add(b) fmt.Println(c) // Outputs 0.3 }
By utilizing the decimal
package, the addition of 0.1 and 0.2 yields the expected result of 0.3, ensuring precision in calculations.
Best Practices
-
Prefer
float64
overfloat32
: Due to its higher precision and wider range,float64
is generally the preferred choice unless memory constraints dictate otherwise. -
Be cautious with equality comparisons: Directly comparing floating-point numbers can lead to unexpected results due to precision issues. Instead, consider checking if the numbers are within a small epsilon value of each other.
-
Utilize precise libraries for critical calculations: For applications requiring exact decimal representation, such as financial software, use libraries like
decimal
to avoid the pitfalls of floating-point arithmetic.
Conclusion
Understanding the intricacies of floating-point numbers in Go is vital for developing reliable and accurate applications. By recognizing the limitations of binary representation and employing appropriate strategies, developers can effectively manage precision and ensure the correctness of their computations.
FAQs
Floating-point numbers in Go follow IEEE-754, where some decimal fractions cannot be exactly represented in binary, leading to precision errors.
Use float32
only when memory constraints are critical, as it has lower precision and a smaller range than float64
.
Use third-party libraries like decimal
to handle arithmetic operations requiring exact decimal precision.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ