Logging in Go: Practices and Libraries
James Reed
Infrastructure Engineer · Leapcell
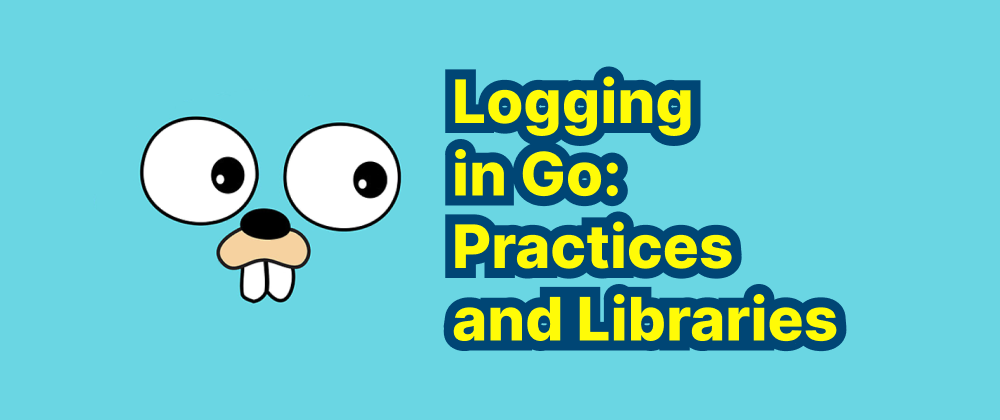
Key Takeaways
- Go provides a built-in
log
package for basic logging needs. - Third-party libraries like
logrus
,zap
,zerolog
, andslog
offer advanced logging features. - Choosing the right logging library depends on performance, structured logging, and community support.
Logging is a fundamental aspect of software development, providing insights into application behavior and aiding in debugging and monitoring. In Go (Golang), developers have access to both the standard library's logging package and a variety of third-party libraries, each offering unique features and capabilities. This article explores how to implement logging in Go and reviews some of the most popular logging libraries available.
Using Go's Standard Library log
Package
Go's standard library includes the log
package, which offers basic logging functionalities. It allows developers to output log messages with timestamps and provides three main sets of functions:
- Print: Logs general information.
- Panic: Logs messages and triggers a panic.
- Fatal: Logs messages and terminates the program using
os.Exit(1)
.
Here's a simple example of using the log
package:
package main import ( "log" ) func main() { log.Println("This is a standard log message.") log.Printf("Logging with a formatted string: %d", 42) }
By default, the log
package outputs to the standard error and includes the date and time in each log entry. Developers can customize the logger's behavior using various flags:
Ldate
: Adds the date (e.g., 2009/01/23).Ltime
: Adds the time (e.g., 01:23:23).Lmicroseconds
: Adds microsecond precision to the time.Llongfile
: Adds the full file path and line number.Lshortfile
: Adds the file name and line number.LUTC
: Uses UTC time instead of the local time zone.
For example, to include the date, time, and short file name in log messages:
log.SetFlags(log.Ldate | log.Ltime | log.Lshortfile)
Third-Party Logging Libraries
While the standard log
package is suitable for basic logging needs, more complex applications may require advanced features such as structured logging, log levels, and better performance. Several third-party libraries address these needs:
1. logrus
logrus
is a structured logger for Go, providing a flexible API and allowing developers to define fields in their log messages. It supports various log levels and hooks for integrating with different logging systems.
Example:
package main import ( log "github.com/sirupsen/logrus" ) func main() { log.WithFields(log.Fields{ "event": "event_name", "topic": "topic_name", }).Info("Event occurred") }
2. zap
Developed by Uber, zap
is known for its high performance and structured logging capabilities. It offers both a "sugared" logger for ergonomic logging and a more performant, type-safe logger.
Example:
package main import ( "go.uber.org/zap" ) func main() { logger, _ := zap.NewProduction() defer logger.Sync() logger.Info("This is an info message", zap.String("key", "value"), ) }
3. zerolog
zerolog
aims to provide zero-allocation JSON logging, making it extremely efficient. It's designed for applications where performance is critical.
Example:
package main import ( "github.com/rs/zerolog/log" ) func main() { log.Info(). Str("key", "value"). Msg("This is an info message") }
4. slog
Introduced in Go 1.21, slog
is a structured logging package added to the standard library. It provides a simple and efficient way to handle structured logs without external dependencies.
Example:
package main import ( "log/slog" ) func main() { logger := slog.New(slog.NewJSONHandler(os.Stdout)) logger.Info("This is an info message", "key", "value") }
Choosing the Right Logging Library
When selecting a logging library for your Go application, consider the following factors:
- Performance: For high-performance applications, libraries like
zap
andzerolog
are optimized for speed and low memory allocation. - Structured Logging: If you require structured logging (e.g., JSON format), libraries like
logrus
,zap
,zerolog
, and the standard library'sslog
offer these capabilities. - Community and Maintenance: Opt for libraries that are actively maintained and have a strong community presence to ensure ongoing support and updates.
Conclusion
Go provides a versatile ecosystem for logging, from the basic functionalities of the standard log
package to advanced features offered by third-party libraries like logrus
, zap
, zerolog
, and the newly introduced slog
. Assess your application's requirements to choose the most appropriate logging solution, ensuring effective monitoring and debugging capabilities.
FAQs
Third-party libraries provide structured logging, better performance, and log level support.
zap
and zerolog
are optimized for speed and low memory allocation.
slog
is Go’s new structured logging package, introduced in Go 1.21 for better JSON logging.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ