Handling Optional Parameters in Golang
James Reed
Infrastructure Engineer · Leapcell
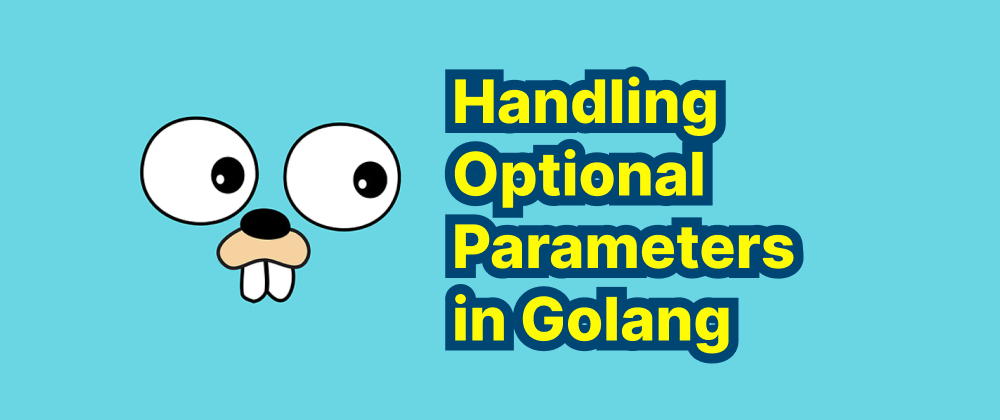
Key Takeaways
- Golang does not support native optional parameters but offers alternative solutions.
- Variadic parameters, structs with defaults, and functional options provide flexibility.
- The functional options pattern is widely used for scalable configurations.
Golang does not support optional parameters in the same way as languages like Python or JavaScript. However, developers can use various techniques to simulate optional parameters and achieve flexibility in function definitions. This article explores different methods to handle optional parameters in Go.
Using Variadic Parameters
One common approach to handle optional parameters in Go is to use variadic parameters. This method allows passing a variable number of arguments to a function.
package main import "fmt" func greet(names ...string) { if len(names) == 0 { fmt.Println("Hello, Guest!") } else { fmt.Println("Hello,", names[0]) } } func main() { greet() greet("Alice") }
In this example, the greet
function can be called with or without a name argument.
Using Structs with Default Values
Another approach is to use structs to encapsulate parameters and provide default values.
package main import "fmt" type Config struct { Host string Port int } func NewConfig(config *Config) { if config.Host == "" { config.Host = "localhost" } if config.Port == 0 { config.Port = 8080 } fmt.Printf("Host: %s, Port: %d\n", config.Host, config.Port) } func main() { NewConfig(&Config{}) NewConfig(&Config{Host: "example.com"}) }
This approach allows flexibility and readability, making it easy to manage multiple optional parameters.
Using Functional Options
A more advanced technique is the functional options pattern, which provides better scalability and customization.
package main import "fmt" type Server struct { host string port int } type Option func(*Server) func WithHost(host string) Option { return func(s *Server) { s.host = host } } func WithPort(port int) Option { return func(s *Server) { s.port = port } } func NewServer(opts ...Option) *Server { server := &Server{host: "localhost", port: 8080} for _, opt := range opts { opt(server) } return server } func main() { s1 := NewServer() s2 := NewServer(WithHost("example.com"), WithPort(9090)) fmt.Printf("Server 1: %s:%d\n", s1.host, s1.port) fmt.Printf("Server 2: %s:%d\n", s2.host, s2.port) }
This pattern is widely used in Go libraries to manage configurations dynamically while keeping the API clean.
Conclusion
Although Golang does not have built-in support for optional parameters, developers can use variadic parameters, structs with default values, or functional options to achieve similar functionality. Each approach has its advantages, and the choice depends on the complexity and maintainability of the codebase.
FAQs
Golang emphasizes simplicity and explicitness, avoiding function overloading and optional parameters.
Use it for complex configurations requiring multiple optional settings.
No, they work well for single-type parameters but may reduce readability for complex cases.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ