Understanding Generic Functions in Go
Grace Collins
Solutions Engineer · Leapcell
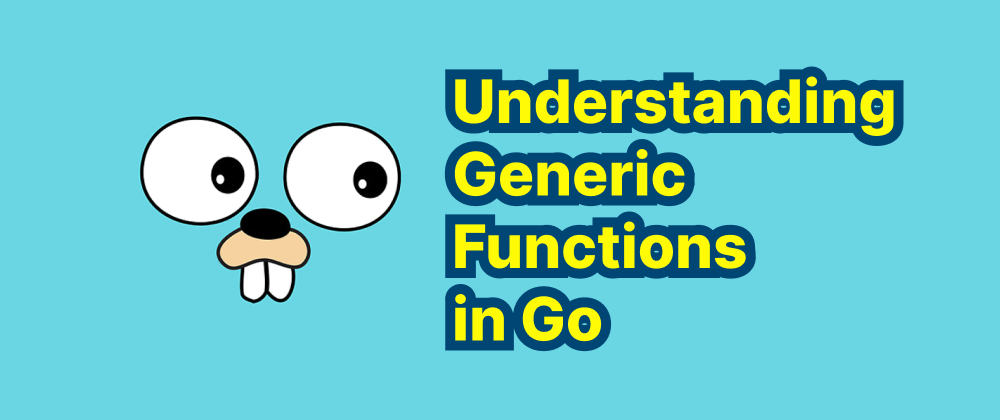
Key Takeaways
- Generic functions improve code reusability and type safety in Go.
- Type constraints help ensure correct operations on generic parameters.
- Generics reduce code duplication and enhance maintainability.
Go, often referred to as Golang, introduced support for generics in version 1.18, marking a significant evolution in the language's capabilities. Generics enable developers to write flexible and reusable functions or data structures that can operate with any data type, enhancing code efficiency and type safety.
What Are Generic Functions?
Generic functions are functions defined with type parameters, allowing them to process inputs of various types while ensuring type safety. This means you can write a function that works with different data types without compromising the integrity of the type system.
Defining a Generic Function in Go
To define a generic function in Go, you introduce type parameters within square brackets []
after the function name. Here's a basic example:
func Print[T any](value T) { fmt.Println(value) }
In this example, Print
is a generic function with a type parameter T
. The any
keyword is a type constraint that allows any type, effectively making T
a placeholder for any data type.
Utilizing Constraints in Generic Functions
While the any
constraint offers flexibility, there are scenarios where you want to restrict the types that a generic function can accept. This is achieved using type constraints. For instance, to create a function that adds two numbers, you can define a constraint that includes all numeric types:
type Number interface { type int, int8, int16, int32, int64, uint, uint8, uint16, uint32, uint64, uintptr, float32, float64, complex64, complex128 } func Add[T Number](a, b T) T { return a + b }
Here, the Number
interface specifies a set of types that support the addition operation. The Add
function is then constrained to only accept types that satisfy the Number
interface, ensuring that the addition operation is valid for the provided types.
Benefits of Using Generic Functions
-
Code Reusability: Generic functions allow you to write functions that can operate on different data types, reducing code duplication.
-
Type Safety: By specifying constraints, you ensure that only appropriate types are used with your functions, catching potential errors at compile time.
-
Maintainability: With generics, your codebase becomes more maintainable as you can implement generalized solutions without sacrificing clarity or performance.
Conclusion
The introduction of generics in Go has significantly enhanced the language's flexibility and expressiveness. By leveraging generic functions, developers can write more abstract, reusable, and type-safe code, leading to cleaner and more efficient programs.
For a more in-depth exploration of Go's generics, consider referring to the official tutorial:
FAQs
Generic functions allow defining functions with type parameters, enabling them to work with multiple data types safely.
Constraints restrict type parameters to specific types, ensuring valid operations and maintaining type safety.
Generics were introduced in Go 1.18.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ