Understanding Timers in Go
Grace Collins
Solutions Engineer · Leapcell
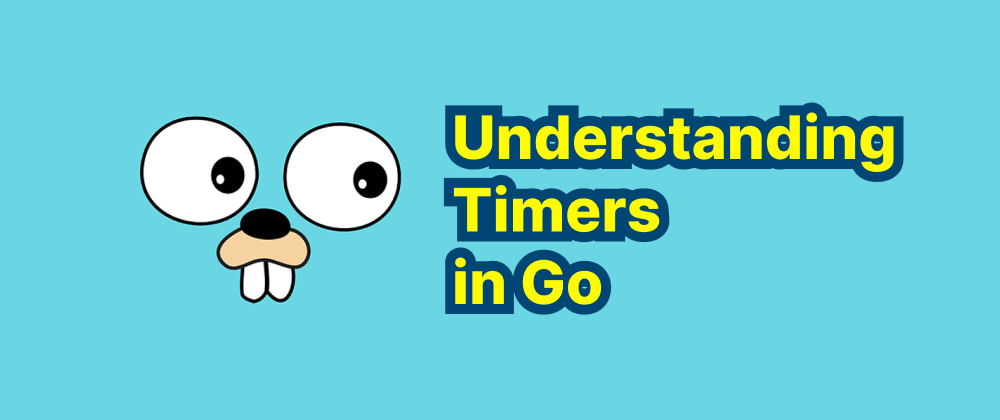
Key Takeaways
- Go's
time.NewTimer
andtime.After
are used to execute code after a specified duration. - Timers can be stopped with
.Stop()
to prevent unnecessary operations. - Properly resetting timers is crucial to avoid race conditions.
Timers are essential components in concurrent programming, enabling the execution of code at specific intervals or after a designated duration. In Go, the time
package offers robust support for timer functionalities, facilitating precise control over time-dependent operations.
Creating a Timer
To create a timer in Go, you can use the time.NewTimer
function, which returns a Timer
object set to expire after a specified duration:
timer := time.NewTimer(2 * time.Second)
This timer will send the current time on its channel C
after approximately two seconds. You can wait for the timer to fire by reading from this channel:
<-timer.C fmt.Println("Timer expired")
Alternatively, for a one-off event after a duration, time.After
can be used:
<-time.After(2 * time.Second) fmt.Println("2 seconds have passed")
Stopping a Timer
A running timer can be stopped before it expires using the Stop
method. This is particularly useful to prevent the timer from firing if its event is no longer needed:
timer := time.NewTimer(2 * time.Second) if stop := timer.Stop(); stop { fmt.Println("Timer stopped before expiration") }
Stopping a timer ensures that its channel C
will not receive a value, which can help prevent goroutine leaks in programs.
Resetting a Timer
Timers can be reset to a new duration using the Reset
method. It's important to note that Reset
should only be called on stopped or expired timers with drained channels to avoid unexpected behavior:
timer := time.NewTimer(2 * time.Second) if !timer.Stop() { <-timer.C // Drain the channel if the timer has expired } timer.Reset(1 * time.Second) <-timer.C fmt.Println("Timer reset and expired")
Improper use of Reset
without stopping or draining the timer can lead to race conditions or unexpected timer behavior.
Practical Use Cases
Timers are versatile tools in Go and are commonly used in various scenarios:
- Timeouts in Network Operations: Implementing timeouts to prevent indefinite blocking during network calls.
- Periodic Tasks: Scheduling tasks to run at regular intervals using timers or tickers.
- Rate Limiting: Controlling the rate of operations to conform to desired thresholds.
- Task Scheduling: Executing tasks at specific times or after certain delays.
- Game Development: Managing time-based events, such as turn durations or cooldown periods.
Conclusion
Understanding and utilizing timers in Go is fundamental for effective time management in applications. By leveraging the time
package's timer functionalities, developers can implement precise and efficient time-based operations, enhancing the responsiveness and reliability of their programs.
FAQs
Use time.NewTimer(duration)
or time.After(duration)
for single-use timers.
Stopping a timer with .Stop()
prevents it from firing, saving resources and avoiding unintended behavior.
Ensure the timer is stopped and its channel is drained before calling .Reset()
.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ