Session, JWT, SSO, OAuth 2.0: Pros, Cons, and Use Cases
James Reed
Infrastructure Engineer · Leapcell
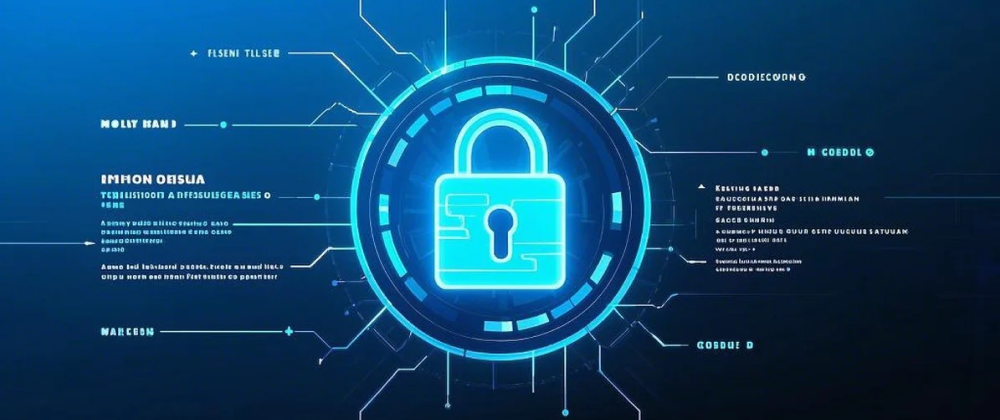
In frontend project development, there are four main methods for user authentication: Session, JWT, SSO, and OAuth 2.0.
So, what are the advantages and disadvantages of these four methods? Today, let's compare them!
Classic Session-Based Authentication
What is Session-Based Authentication?
Session-based authentication is a commonly used user authentication method in frontend and backend systems.
It primarily relies on the server to create and manage user sessions.
How Session Works
The session authentication process consists of six steps:
- User Login: The user enters their credentials (e.g., username and password) on the login page. These credentials are sent from the frontend to the backend server for validation.
- Create a Session: After validating the credentials, the backend server creates a session, usually including a unique session ID, which is stored on the server.
- Return the Session ID: The server sends the session ID back to the frontend, usually via a cookie. This cookie is stored in the user's browser and will be automatically sent with subsequent requests.
- Store the Session ID: The browser saves the cookie and automatically includes it in every request sent to the server. This allows the server to recognize the user's session and authenticate them.
- Session Validation: The server looks up the session ID and verifies the associated session information to determine the user's identity. It can also use session data for permission checks and access control.
- Session Expiration and Management: The server can set session expiration times and periodically clear expired sessions. When a user logs out or the session times out, the server deletes or invalidates the session.
From the above process, we can see that in session-based authentication, the frontend does not need to actively participate. The key operations are handled between the browser and the server.
Pros and Cons
Advantages
- Simple and Easy to Use: Managing sessions and user authentication is relatively straightforward for developers.
- Good Compatibility: Most browsers support cookies, enabling automatic sending and receiving.
Disadvantages
- Poor Scalability: In distributed systems, multiple servers may need to share session storage, increasing complexity.
- Requires HTTPS: If cookies are stolen, it could lead to session hijacking. Therefore, HTTPS should be used to secure data transmission, along with additional security measures (e.g., setting cookies with
HttpOnly
andSecure
attributes).
Example Code
Here’s an example of implementing session authentication using Express:
const express = require('express'); const session = require('express-session'); const app = express(); // Configure and use express-session middleware app.use( session({ secret: 'your-secret-key', // Key used to sign the session ID cookie, ensuring session security resave: false, // Whether to save the session on every request, even if it hasn’t changed saveUninitialized: true, // Whether to save an uninitialized session cookie: { secure: true, // Whether to send cookies only over HTTPS (requires HTTPS support) maxAge: 24 * 60 * 60 * 1000, // Cookie expiration time (set to 24 hours) }, }) ); // Login route handler app.post('/login', (req, res) => { // Authenticate user (assume the user has been validated) const user = { id: 123 }; // Example user ID req.session.userId = user.id; // Store user ID in session res.send('Login successful'); }); app.get('/dashboard', (req, res) => { if (req.session.userId) { // If the session contains a user ID, the user is logged in res.send('Dashboard content...'); } else { // If no user ID is found in the session, the user is not logged in res.send('Please log in...'); } }); app.listen(3000, () => { console.log('Server is listening on port 3000...'); });
JWT (JSON Web Token) Authentication
What is JWT Authentication?
JWT authentication is currently one of the most commonly used authentication methods.
The server returns a token representing the user identity. In requests, the token is added to the request headers for user verification.
Since HTTP requests are stateless, this method is also known as stateless authentication.
How JWT Works
- User Login: The user enters their credentials (e.g., username and password) on the login page, and these credentials are sent to the backend server for validation.
- Generate JWT: After validating the user credentials, the backend server generates a JWT. This token typically contains basic user information (e.g., user ID) and metadata (e.g., expiration time).
- Return JWT: The server sends the generated JWT back to the frontend, usually in a JSON response.
- Store JWT: The frontend stores the JWT on the client side, typically in
localStorage
. In rare cases, it may be stored in cookies, but this carries security risks such as Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF). - Use JWT for Requests: When making API calls, the frontend attaches the JWT token in the
Authorization
header (format:Bearer <token>
) and sends it to the server. - Validate JWT: Upon receiving the request, the server extracts the JWT, verifies its validity (e.g., checking the signature and expiration time). If valid, the server processes the request and returns the corresponding resource or data.
- Respond to the Request: The server handles the request and returns a response, which the frontend can use as needed.
Pros and Cons
Advantages
- Stateless: JWTs are self-contained, meaning the server does not need to store session information, simplifying scalability and load balancing.
- Cross-Domain Support: JWT can be used in cross-origin requests (e.g., when APIs and frontends are separated).
Disadvantages
- Security Concerns: The security of JWT depends on key protection and token expiration management. If a JWT is stolen, it could pose security risks.
Example Code
Here’s an example of implementing JWT authentication using Express:
const express = require('express'); const jwt = require('jsonwebtoken'); const bodyParser = require('body-parser'); const app = express(); app.use(bodyParser.json()); const secretKey = 'your-secret-key'; // Secret key for signing and verifying JWTs // Login route that generates a JWT app.post('/login', (req, res) => { const { username, password } = req.body; // User authentication (assuming the user is valid) const user = { id: 1, username: 'user' }; // Example user data const token = jwt.sign(user, secretKey, { expiresIn: '24h' }); // Generate JWT res.json({ token }); // Return JWT }); // Protected route app.get('/dashboard', (req, res) => { const token = req.headers['authorization']?.split(' ')[1]; if (!token) { return res.status(401).send('No token provided'); } jwt.verify(token, secretKey, (err, decoded) => { if (err) { return res.status(401).send('Invalid token'); } res.send('Dashboard content'); }); }); app.listen(3000, () => { console.log('Server is listening on port 3000...'); });
SSO (Single Sign-On) Authentication
What is SSO Authentication?
SSO authentication is commonly used in "suite-based" applications. Through a central login system, users can log in once and gain access to multiple applications without needing to reauthenticate.
How SSO Works
- User Accesses an Application: The user attempts to access an application that requires authentication (called the Service Provider (SP)).
- Redirect to Identity Provider (IdP): Since the user is not logged in, the application redirects them to an SSO Identity Provider (IdP) (commonly referred to as the Login Center). The Login Center handles user authentication.
- User Logs In: The user enters their credentials at the Login Center. If they are already logged in (e.g., logged into the company's internal SSO system), they may bypass this step.
- SSO Token Generation: Once authenticated, the Identity Provider generates an SSO token (e.g., an OAuth token or a SAML assertion) and redirects the user back to the original application, attaching the token.
- Token Validation: The application (Service Provider) receives the token and sends it to the SSO Identity Provider for validation. The IdP verifies the token and returns the user’s identity information.
- User Gains Access: After successful authentication, the application grants access based on the user’s identity information. The user can now access the protected resources of the application.
- Accessing Other Applications: If the user accesses other applications that also use the same Login Center, they are redirected there. Since they are already logged in, the Login Center automatically authenticates them and redirects them back to the target application, enabling seamless login.
Pros and Cons
Advantages
- Simplifies User Experience: Users only need to log in once to access multiple applications, reducing repetitive login efforts.
- Centralized Management: Administrators can centrally manage user identities and access permissions, improving efficiency and security.
- Enhanced Security: Reduces the risk of password leaks, as users only need to remember one password. Stronger authentication mechanisms (e.g., Multi-Factor Authentication, MFA) can also be enforced.
Disadvantages
- Single Point of Failure: If the Login Center (SSO Identity Provider) encounters an issue, all applications relying on it may be affected.
- Complex Implementation: Deploying and maintaining an SSO solution can be complex, requiring proper security configurations and interoperability between systems.
Common SSO Implementation Technologies
SAML (Security Assertion Markup Language)
- An XML-based standard used for exchanging authentication and authorization data between Identity Providers (IdPs) and Service Providers (SPs).
- Commonly used in enterprise environments.
OAuth 2.0 & OpenID Connect
- OAuth 2.0 is an authorization framework for granting third-party access to user resources.
- OpenID Connect is built on top of OAuth 2.0, adding an identity layer for user authentication.
- Often used in web and mobile applications.
CAS (Central Authentication Service)
- An open-source SSO solution for web applications, allowing users to authenticate once and access multiple services.
OAuth 2.0 Authentication
What is OAuth 2.0 Authentication?
OAuth 2.0 is a standard protocol used for authorizing third-party applications to access user resources. Examples include:
- Facebook Login
- Discord Login
- App QR Code Login
OAuth 2.0 is primarily designed for authorization rather than authentication, but it is often combined with authentication to enable user login.
How OAuth 2.0 Works
OAuth 2.0 is complex, so before understanding its flow, we need to clarify some key concepts.
Key Concepts in OAuth 2.0
- Resource Owner: Typically the user who owns the protected resource (e.g., personal data, files).
- Resource Server: The server that hosts the protected resources and ensures they are only accessible to authorized users.
- Client: The application or service that wants to access the protected resource. The client must obtain the resource owner's authorization.
- Authorization Server: The server responsible for authenticating the resource owner and granting authorization to the client. It issues access tokens that allow the client to access the resource server.
OAuth 2.0 Workflow
- User Grants Authorization: When the user interacts with a client application, the application requests authorization to access the user’s resources. The user is redirected to the Authorization Server.
- Obtain Authorization Code: If the user consents, the Authorization Server generates an Authorization Code and sends it back to the client (via a redirect URL).
- Obtain Access Token: The client exchanges the Authorization Code for an Access Token by making a request to the Authorization Server.
- Access Resources: The client uses the Access Token to request protected resources from the Resource Server. The Resource Server validates the token and returns the requested data.
Common OAuth 2.0 Grant Types (Authorization Flows)
-
Authorization Code Flow (Most Common)
- Suitable for web applications that require user interaction.
- The client exchanges the authorization code for an access token after obtaining user consent.
-
Implicit Flow (Not Recommended)
- Designed for public clients (e.g., Single-Page Applications).
- The user directly receives an access token instead of an authorization code.
- Due to security concerns, this flow is no longer recommended.
-
Resource Owner Password Credentials Flow (Limited Use)
- The user provides their username and password directly to the client.
- The client requests an access token using these credentials.
- Not recommended for public-facing applications due to security risks.
-
Client Credentials Flow (Machine-to-Machine)
- Used when a client application needs to access its own resources, rather than acting on behalf of a user.
- Common for API access between services.
Pros and Cons
Advantages
- Flexibility: Supports multiple authorization flows, making it suitable for different client types and application scenarios.
- Security: By separating authorization and authentication, OAuth 2.0 enhances security. Instead of using usernames and passwords, it relies on tokens for access control.
Disadvantages
- Complexity: Implementing and configuring OAuth 2.0 can be challenging, requiring proper token management and security configurations.
- Security Risks: If a token is leaked, it can pose a security risk. Therefore, proper security measures (e.g., using HTTPS and secure token management strategies) must be in place.
Example Code
Here’s an Express implementation of OAuth 2.0 authentication:
const express = require('express'); const axios = require('axios'); const app = express(); // OAuth 2.0 Configuration const clientId = 'your-client-id'; const clientSecret = 'your-client-secret'; const redirectUri = 'http://localhost:3000/callback'; const authorizationServerUrl = 'https://authorization-server.com'; const resourceServerUrl = 'https://resource-server.com'; // Login route - Redirects user to the Authorization Server app.get('/login', (req, res) => { const authUrl = `${authorizationServerUrl}/authorize?response_type=code&client_id=${clientId}&redirect_uri=${redirectUri}&scope=read`; res.redirect(authUrl); }); // Callback route - Handles Authorization Code exchange app.get('/callback', async (req, res) => { const { code } = req.query; if (!code) { return res.status(400).send('Authorization code is missing'); } try { // Exchange authorization code for an access token const response = await axios.post(`${authorizationServerUrl}/token`, { grant_type: 'authorization_code', code, redirect_uri: redirectUri, client_id: clientId, client_secret: clientSecret, }); const { access_token } = response.data; // Use the access token to fetch protected resources const resourceResponse = await axios.get(`${resourceServerUrl}/user-info`, { headers: { Authorization: `Bearer ${access_token}` }, }); res.json(resourceResponse.data); } catch (error) { res.status(500).send('Error during token exchange or resource access'); } }); app.listen(3000, () => { console.log('Server is listening on port 3000...'); });
Conclusion
Each of these four authentication methods has its own advantages, disadvantages, and suitable use cases:
- Session: Ideal for simple server-rendered applications.
- JWT: Suitable for modern stateless architectures and mobile apps.
- SSO: Best for enterprise environments with multiple related services.
- OAuth 2.0: The preferred choice for third-party integrations and API access.
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ