Understanding the `goto` Statement in Go
Grace Collins
Solutions Engineer · Leapcell
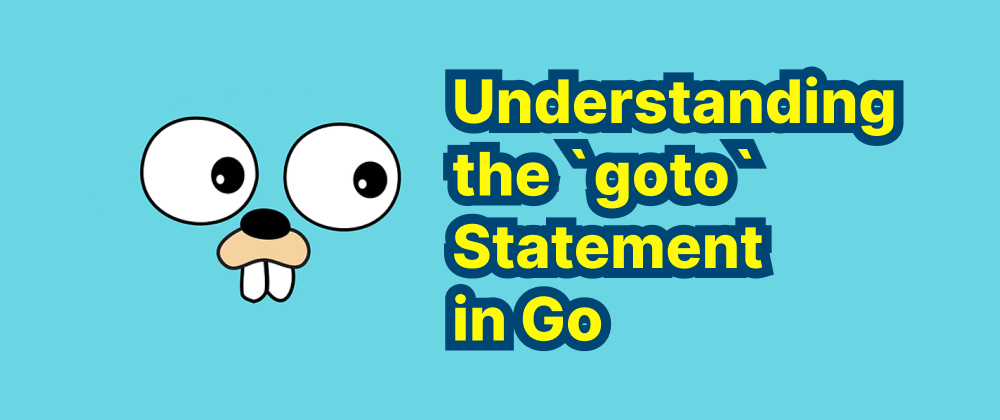
Key Takeaways
- The
goto
statement allows for an unconditional jump within a function. - It can be useful for exiting nested loops or simplifying error handling.
- Overuse of
goto
can make code difficult to read and maintain.
In Go, the goto
statement provides a way to jump to a labeled statement within the same function. While its use is generally discouraged in favor of structured control flow constructs, understanding how it works can be beneficial in certain scenarios.
Syntax of goto
The syntax for the goto
statement in Go is straightforward:
goto labelName
Here, labelName
is an identifier that marks the destination of the jump. The label is defined by placing the identifier followed by a colon (:
) before a statement in the code:
labelName: // statement
Example Usage
Consider the following example where goto
is used to exit nested loops when a specific condition is met:
package main import "fmt" func main() { found := false for i := 0; i < 10; i++ { for j := 0; j < 10; j++ { if i*j > 50 { found = true goto exitLoops } } } exitLoops: if found { fmt.Println("Found a pair where i*j > 50") } else { fmt.Println("No such pair found") } }
In this example, the goto
statement allows for an immediate exit from both loops once the condition i*j > 50
is satisfied, jumping to the exitLoops
label.
Considerations When Using goto
While goto
can simplify certain control flows, especially when dealing with multiple nested loops or error handling, it should be used sparingly. Overuse of goto
can lead to code that is difficult to read and maintain, often referred to as "spaghetti code." It's generally advisable to use structured control flow constructs like loops and conditionals to manage program flow.
Conclusion
The goto
statement in Go offers a way to perform unconditional jumps within a function. Although it can be useful in specific cases, it's important to use it judiciously to maintain code readability and structure.
FAQs
Use it sparingly, mainly for breaking out of deeply nested loops or simplifying error handling.
Yes, goto
can only jump within the same function; it cannot cross function boundaries.
Use break
, continue
, or return statements where possible for better readability.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ