Understanding `strconv.Atoi` in Golang
Daniel Hayes
Full-Stack Engineer · Leapcell
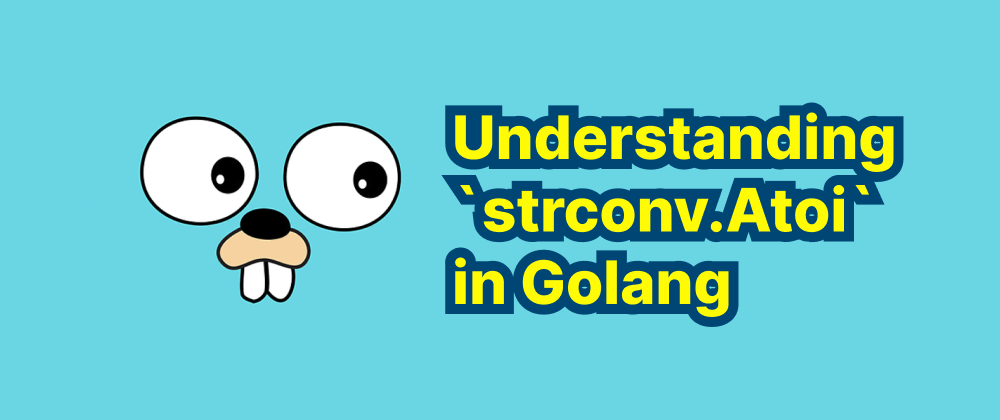
Key Takeaways
strconv.Atoi
converts numeric strings to integers in Go.- Proper error handling is essential to avoid conversion failures.
- For advanced conversions,
strconv.ParseInt
offers more control.
Golang provides a robust standard library for handling various data conversions, and one of the most commonly used functions for converting strings to integers is strconv.Atoi
. This function is essential when dealing with user input, configuration values, or data parsing where numbers are represented as strings.
What is strconv.Atoi
?
The strconv.Atoi
function is part of the strconv
package in Go. It is specifically designed to convert a string containing numerical characters into an integer.
Syntax:
func Atoi(s string) (int, error)
This function takes a string s
as input and returns an integer representation of the string along with an error
value. If the conversion is successful, the error is nil
; otherwise, an error is returned indicating the failure.
Example Usage
Below is a simple example demonstrating how to use strconv.Atoi
:
package main import ( "fmt" "strconv" ) func main() { str := "123" num, err := strconv.Atoi(str) if err != nil { fmt.Println("Conversion failed:", err) } else { fmt.Println("Converted integer:", num) } }
Output:
Converted integer: 123
In this example, the string "123"
is successfully converted into the integer 123
. If the string contained invalid characters (e.g., "123abc"
), an error would be returned.
Handling Errors in Atoi
Error handling is crucial when using strconv.Atoi
since improper input can cause conversion failures.
Consider the following example:
package main import ( "fmt" "strconv" ) func main() { str := "123abc" num, err := strconv.Atoi(str) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Converted integer:", num) } }
Output:
Error: strconv.Atoi: parsing "123abc": invalid syntax
Since "123abc"
contains non-numeric characters, Atoi
fails and returns an error.
Alternative: Using strconv.ParseInt
While strconv.Atoi
is convenient, it is a simplified wrapper around strconv.ParseInt
. If you need more control over the conversion (such as specifying the base or bit size), you can use strconv.ParseInt
:
num, err := strconv.ParseInt("123", 10, 32)
This function allows specifying:
- Base: The number system (e.g., 10 for decimal, 16 for hexadecimal).
- Bit size: The target integer size (e.g., 32-bit or 64-bit).
When to Use Atoi
- When converting simple numerical strings to integers.
- When working with user input that should be an integer.
- When parsing configuration values stored as strings.
Conclusion
strconv.Atoi
is a useful function in Go for converting numeric strings into integers. However, since it does not handle non-numeric characters gracefully, proper error handling is essential. When dealing with more complex conversions, strconv.ParseInt
may be a better choice.
By understanding the strengths and limitations of strconv.Atoi
, you can write more reliable and efficient Go programs.
FAQs
It converts a numeric string to an integer in Go.
Atoi
returns an error indicating invalid syntax.
Use it when needing to specify the base or bit size for conversion.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ