Working with Base64 in Go
Grace Collins
Solutions Engineer · Leapcell
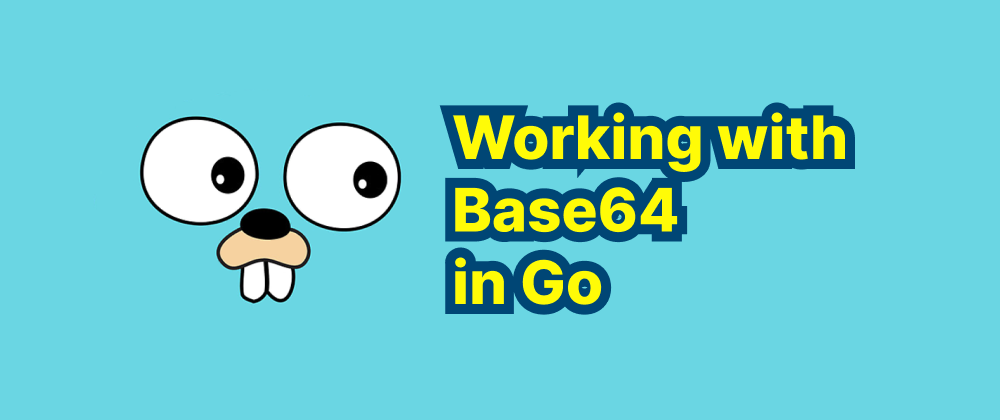
Key Takeaways
- Go's
encoding/base64
package simplifies encoding and decoding operations. - Use
URLEncoding
for safe encoding in URLs and filenames. - Base64 is ideal for handling binary data like images and files.
Base64 encoding is a common technique used to represent binary data in an ASCII string format. This is particularly useful when transmitting data over media that are designed to handle textual data. In Go, the encoding/base64
package provides comprehensive support for Base64 encoding and decoding operations. This article explores how to utilize this package effectively.
Importing the Base64 Package
To begin working with Base64 in Go, you need to import the encoding/base64
package:
import ( "encoding/base64" )
Encoding Data to Base64
Encoding data to Base64 involves converting your binary or textual data into a Base64 encoded string. Here's how you can do it:
package main import ( "encoding/base64" "fmt" ) func main() { data := "Hello, Gophers!" encoded := base64.StdEncoding.EncodeToString([]byte(data)) fmt.Println("Encoded:", encoded) }
In this example:
- We define a string
data
containing "Hello, Gophers!". - The
EncodeToString
function frombase64.StdEncoding
encodes the byte slice ofdata
into a Base64 encoded string. - The encoded string is then printed to the console.
Decoding Base64 Data
Decoding a Base64 encoded string back to its original form is straightforward:
package main import ( "encoding/base64" "fmt" ) func main() { encoded := "SGVsbG8sIEdvcGhlcnMh" decoded, err := base64.StdEncoding.DecodeString(encoded) if err != nil { fmt.Println("Error decoding string:", err) return } fmt.Println("Decoded:", string(decoded)) }
Here:
- We have a Base64 encoded string
encoded
. - The
DecodeString
function decodes the encoded string back into its original byte slice. - We handle any potential errors that might occur during decoding.
- The decoded byte slice is converted back to a string and printed.
URL and Filename Safe Base64 Encoding
The standard Base64 encoding uses characters (+
and /
) that can be problematic in URLs and filenames. Go provides a URL and filename safe Base64 encoding that replaces these characters with -
and _
respectively:
package main import ( "encoding/base64" "fmt" ) func main() { data := "Hello, Gophers!" encoded := base64.URLEncoding.EncodeToString([]byte(data)) fmt.Println("URL Encoded:", encoded) decoded, err := base64.URLEncoding.DecodeString(encoded) if err != nil { fmt.Println("Error decoding string:", err) return } fmt.Println("URL Decoded:", string(decoded)) }
In this snippet:
- We use
base64.URLEncoding
to encode the data, making it safe for URLs and filenames. - Decoding is performed using the same
URLEncoding
to ensure consistency.
Handling Binary Data
Base64 encoding is often used to handle binary data, such as images or files. Here's how you can encode and decode binary data:
package main import ( "encoding/base64" "fmt" "io/ioutil" ) func main() { // Read binary data from a file data, err := ioutil.ReadFile("example.png") if err != nil { fmt.Println("Error reading file:", err) return } // Encode binary data to Base64 encoded := base64.StdEncoding.EncodeToString(data) fmt.Println("Encoded:", encoded) // Decode Base64 back to binary data decoded, err := base64.StdEncoding.DecodeString(encoded) if err != nil { fmt.Println("Error decoding string:", err) return } // Write decoded data back to a file err = ioutil.WriteFile("decoded_example.png", decoded, 0644) if err != nil { fmt.Println("Error writing file:", err) return } }
In this example:
- We read binary data from a file named
example.png
. - The binary data is encoded to a Base64 string.
- The Base64 string is then decoded back to binary data.
- Finally, we write the decoded data to a new file named
decoded_example.png
.
Conclusion
The encoding/base64
package in Go provides robust functions for encoding and decoding data to and from Base64. Whether you're working with textual data or binary files, understanding how to use this package is essential for tasks that involve data transmission or storage in text-based formats.
FAQs
Use base64.StdEncoding.EncodeToString([]byte(data))
to encode a string.
Use base64.URLEncoding.EncodeToString
to avoid unsafe URL characters.
Yes, binary data can be encoded and decoded using the same methods.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ