Understanding Go Build Tags
Daniel Hayes
Full-Stack Engineer · Leapcell
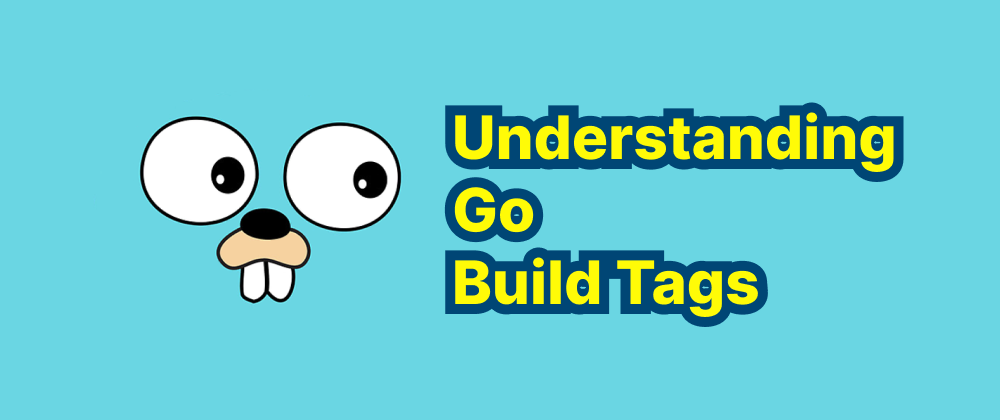
Key Takeaways
- Go build tags control file inclusion during the build process based on specified conditions.
- They are commonly used for platform-specific code and feature toggling.
- Use the
//go:build
syntax for consistency, especially in Go 1.17 and later versions.
Go build tags are a powerful feature that allows developers to include or exclude files from the build process based on specific conditions. This mechanism is particularly useful for managing platform-specific code, enabling or disabling features, and controlling dependencies.
What Are Build Tags?
Build tags are special comments in Go source files that instruct the Go toolchain on when to include or exclude a file during the build process. They are placed at the top of the file, preceding the package declaration, and follow this syntax:
//go:build <expression>
Prior to Go 1.17, the syntax used was:
// +build <expression>
The new //go:build
syntax is now the standard, but the old // +build
form is still supported for backward compatibility.
How to Use Build Tags
To use build tags, add the //go:build
comment at the top of your Go source file, specifying the conditions under which the file should be included in the build. For example:
//go:build linux package main import "fmt" func main() { fmt.Println("This code is for Linux systems.") }
In this example, the file will only be included in the build when targeting a Linux system.
Common Use Cases
Platform-Specific Code
Build tags are often used to include or exclude code based on the target operating system or architecture. For instance:
//go:build darwin package main func platformSpecificFunction() { // macOS-specific implementation }
This file will only be included when building for macOS (darwin
).
Conditional Compilation
You can define custom build tags to enable or disable features. For example:
//go:build debug package main func debugLog(message string) { // Debug logging implementation }
To include this file in the build, you would use the -tags
flag:
go build -tags=debug
Excluding Files from Build
To exclude a file from the build, you can use a negation in the build tag:
//go:build !release package main func nonReleaseFunction() { // This code is excluded in release builds }
Combining Multiple Conditions
Build tags support logical expressions to combine multiple conditions. For example:
//go:build (linux || darwin) && amd64 package main func main() { // This code is for Linux or macOS on amd64 architecture. }
In this case, the file is included only when building for Linux or macOS on the amd64
architecture.
Best Practices
- Consistency: Use the
//go:build
syntax introduced in Go 1.17 for new codebases. - Documentation: Clearly document the purpose of each build tag to maintain code readability.
- Testing: Ensure that all code paths controlled by build tags are properly tested to prevent platform-specific issues.
Conclusion
Go build tags provide a flexible way to control the inclusion of files in the build process, enabling developers to manage platform-specific code and conditional features effectively. By understanding and utilizing build tags, you can create more adaptable and maintainable Go applications.
FAQs
They manage the inclusion or exclusion of files during the build based on conditions like OS or custom tags.
Use the -tags
flag during build, e.g., go build -tags=debug
.
Yes, you can use logical expressions like //go:build (linux || darwin) && amd64
.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ