Understanding `try` and `except` in Python
James Reed
Infrastructure Engineer · Leapcell
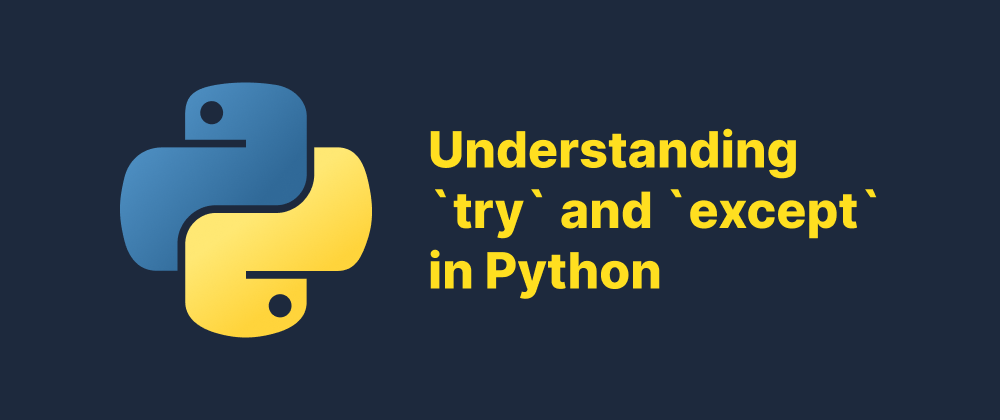
Key Takeaways
try
andexcept
allow Python programs to handle errors gracefully.- Catching specific exceptions improves clarity and debuggability.
else
andfinally
provide additional control over error handling flow.
Error handling is a critical aspect of writing robust and fault-tolerant Python programs. In Python, the try
and except
block provides a clean and readable way to manage exceptions and prevent your code from crashing unexpectedly.
Why Use try
and except
?
When you write code that interacts with the outside world—such as reading files, accessing a database, or making network requests—there's always a chance that something can go wrong. Instead of letting your program crash when it encounters an error, you can use try
and except
to gracefully handle the situation.
Basic Syntax
The basic structure of a try
-except
block in Python is as follows:
try: # Code that might raise an exception result = 10 / 0 except ZeroDivisionError: # Code that runs if the exception occurs print("You can't divide by zero!")
In this example, Python attempts to execute the code inside the try
block. When it encounters a ZeroDivisionError
, it jumps to the except
block and prints an error message.
Catching Multiple Exceptions
You can handle multiple exception types by specifying multiple except
clauses:
try: user_input = int(input("Enter a number: ")) result = 100 / user_input except ValueError: print("Invalid input! Please enter a number.") except ZeroDivisionError: print("You can't divide by zero.")
Each except
clause handles a specific type of error. This allows your program to respond appropriately to different kinds of problems.
Using else
and finally
Python also supports optional else
and finally
clauses to provide even more control:
else
: Executes if no exceptions occur.finally
: Executes no matter what, even if an exception was raised.
try: number = int(input("Enter a number: ")) print("You entered:", number) except ValueError: print("That's not a valid number.") else: print("Great! No errors occurred.") finally: print("This message always shows up.")
Catching All Exceptions (Not Always Recommended)
You can catch all exceptions using a bare except
, but this is discouraged unless absolutely necessary:
try: risky_operation() except: print("An unexpected error occurred.")
This catches all exceptions, including system-exiting ones like KeyboardInterrupt
, which might not be what you want.
Best Practices
- Catch specific exceptions: This makes your code easier to debug and understand.
- Avoid bare
except
: Unless you’re logging or handling truly unknown errors. - Use
finally
for cleanup: Ideal for closing files or network connections. - Don’t hide bugs: Handle errors meaningfully rather than silently skipping over them.
Conclusion
The try
and except
construct is a powerful tool in Python for building resilient applications. By using it thoughtfully, you can write programs that are both user-friendly and maintainable. Mastering exception handling is a key step in becoming a proficient Python developer.
FAQs
The program will crash unless the error is also caught by another try
-except
block.
It's not recommended unless you're handling truly unexpected errors; it can hide bugs.
Use finally
for cleanup tasks like closing files or releasing resources.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ