How to Add Elements to a Set in Python
James Reed
Infrastructure Engineer · Leapcell
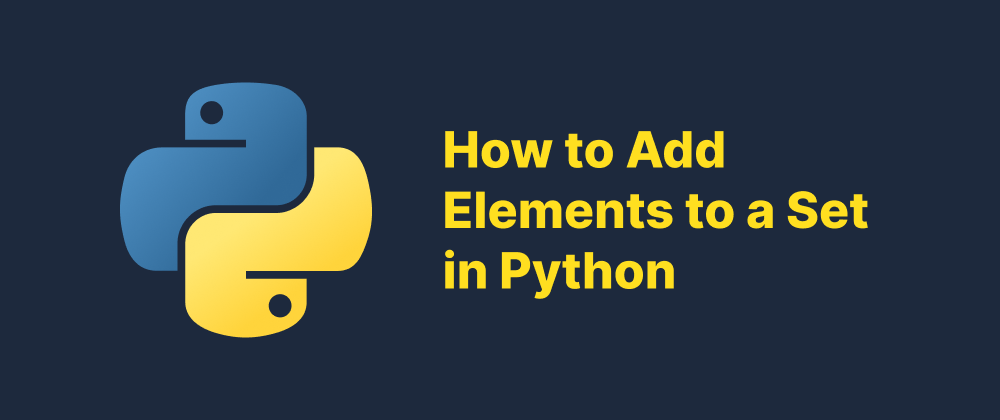
Key Takeaways
- Use
add()
to insert a single element into a set. - Use
update()
to add multiple elements from an iterable. - Sets automatically ignore duplicate elements.
In Python, a set is an unordered collection of unique elements. This means that each element in a set must be immutable and cannot appear more than once. Sets are particularly useful when you need to eliminate duplicate values or perform mathematical set operations like union, intersection, and difference.
Using the add()
Method
To add a single element to a set, you can use the add()
method. This method adds the specified element to the set if it's not already present. If the element is already in the set, the set remains unchanged.
Syntax:
your_set.add(element)
Example:
# Creating a set of fruits fruits = {"apple", "banana", "cherry"} # Adding a new fruit to the set fruits.add("orange") print(fruits) # Output: {'apple', 'banana', 'cherry', 'orange'}
In this example, the string "orange"
is added to the fruits
set. Since sets are unordered collections, the order of elements in the output may vary.
It's important to note that if you attempt to add an element that already exists in the set, the set will not change:
# Attempting to add a duplicate element fruits.add("apple") print(fruits) # Output: {'apple', 'banana', 'cherry', 'orange'}
The set remains the same because "apple"
was already an element of the set.
Adding Multiple Elements
If you want to add multiple elements to a set at once, you can use the update()
method. This method takes an iterable (like a list, tuple, or another set) and adds all its elements to the set.
Example:
# Creating a set of fruits fruits = {"apple", "banana", "cherry"} # Adding multiple fruits to the set fruits.update(["mango", "grape", "pineapple"]) print(fruits) # Output: {'apple', 'banana', 'cherry', 'mango', 'grape', 'pineapple'}
In this case, the elements "mango"
, "grape"
, and "pineapple"
are added to the fruits
set. As with the add()
method, any duplicate elements are ignored, and the order of elements in the set is not guaranteed.
Important Considerations
-
Uniqueness: Sets automatically handle duplicates. If you add an element that already exists in the set, it will not be added again.
-
Immutability of Elements: The elements of a set must be immutable (e.g., numbers, strings, tuples). Mutable data types like lists or dictionaries cannot be added to a set.
-
Unordered Collection: Sets do not maintain any specific order. When you print a set or iterate over its elements, the order may differ from the order in which elements were added.
By understanding and utilizing the add()
and update()
methods, you can effectively manage and manipulate sets in Python to suit your programming needs.
FAQs
The set remains unchanged, as duplicates are ignored.
No, only immutable types (like strings or tuples) can be added.
No, sets are unordered collections; element order is not maintained.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ