Blockchain Development with Chainstack and Python
James Reed
Infrastructure Engineer ยท Leapcell
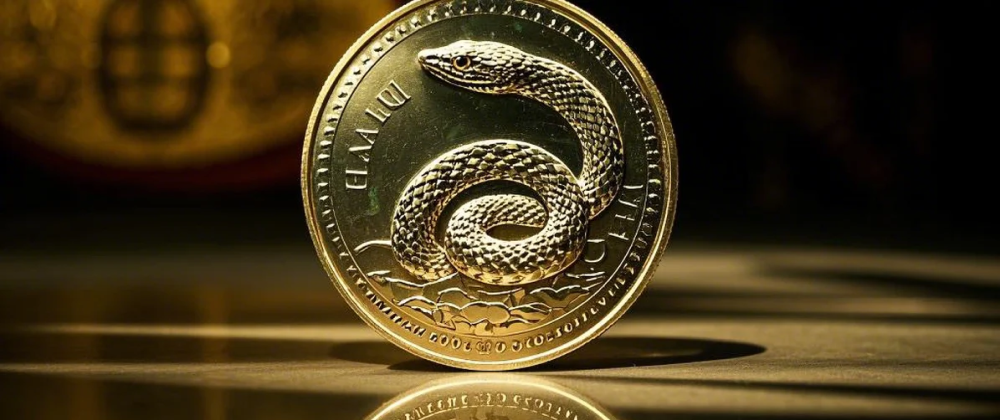
Chainstack is a robust platform that simplifies the deployment and management of blockchain nodes, providing developers with reliable infrastructure for building and interacting with decentralized applications (DApps). By integrating Chainstack with Python, you can efficiently manage blockchain interactions within your Python applications. This tutorial will guide you through the process of setting up Chainstack and using Python to interact with blockchain networks.
Key Takeaways
- Chainstack simplifies blockchain node deployment and management for developers.
- Python, with Web3.py, enables interaction with blockchain nodes.
- Secure handling of credentials and private keys is critical for blockchain development.
Prerequisites
Before you begin, ensure you have the following:
-
Python: Installed on your machine. You can download it from the official website.
-
Chainstack Account: Sign up at Chainstack to access blockchain node services.
-
Web3.py Library: This is a Python library for interacting with Ethereum. Install it using pip:
pip install web3
Step 1: Deploy a Blockchain Node on Chainstack
-
Sign Up and Log In: Create an account on Chainstack and log in.
-
Deploy a Node:
- Navigate to the Projects section and create a new project.
- Within your project, click on Deploy a node.
- Select the desired blockchain protocol (e.g., Ethereum) and network (e.g., Mainnet, Testnet).
- Choose the node type and deployment options as per your requirements.
- Deploy the node and wait for the deployment to complete.
-
Access Node Credentials:
- Once deployed, go to the Nodes section in your project.
- Click on your node to view its details.
- Note the RPC endpoint URL; you'll need this to connect via Python.
Step 2: Connect to the Blockchain Node Using Python
-
Set Up Environment Variables:
-
Store your Chainstack node's RPC endpoint in an environment variable for security.
-
Create a
.env
file in your project directory and add:WEB3_PROVIDER_URI=YOUR_CHAINSTACK_RPC_ENDPOINT
-
Replace
YOUR_CHAINSTACK_RPC_ENDPOINT
with the actual endpoint URL.
-
-
Load Environment Variables:
-
Install the
python-dotenv
package to manage environment variables:pip install python-dotenv
-
In your Python script, load the environment variables:
import os from dotenv import load_dotenv load_dotenv() web3_provider_uri = os.getenv('WEB3_PROVIDER_URI')
-
-
Initialize Web3 Connection:
-
Use Web3.py to connect to your Chainstack node:
from web3 import Web3 web3 = Web3(Web3.HTTPProvider(web3_provider_uri)) if web3.isConnected(): print("Successfully connected to the blockchain.") else: print("Connection failed.")
-
Step 3: Interact with the Blockchain
With the connection established, you can perform various blockchain operations. Here are some examples:
-
Fetch the Latest Block Number:
latest_block = web3.eth.block_number print(f"Latest block number: {latest_block}")
-
Retrieve Block Details:
block = web3.eth.get_block(latest_block) print(f"Block details: {block}")
-
Send a Transaction:
- Ensure your account has sufficient funds and the private key is securely managed.
from web3.middleware import geth_poa_middleware from eth_account import Account # For networks like BNB Smart Chain, inject the PoA middleware web3.middleware_onion.inject(geth_poa_middleware, layer=0) # Replace with your private key and account address private_key = 'YOUR_PRIVATE_KEY' account = Account.from_key(private_key) to_address = 'RECIPIENT_ADDRESS' value = web3.to_wei(0.01, 'ether') # Amount to send # Create and sign the transaction transaction = { 'to': to_address, 'value': value, 'gas': 21000, 'gasPrice': web3.to_wei('5', 'gwei'), 'nonce': web3.eth.get_transaction_count(account.address), 'chainId': web3.eth.chain_id } signed_txn = web3.eth.account.sign_transaction(transaction, private_key=private_key) # Send the transaction tx_hash = web3.eth.send_raw_transaction(signed_txn.rawTransaction) print(f"Transaction sent with hash: {tx_hash.hex()}")
Note: Replace
'YOUR_PRIVATE_KEY'
and'RECIPIENT_ADDRESS'
with your actual private key and the recipient's address, respectively. Handle private keys securely and avoid hardcoding them in your scripts.
Additional Resources
-
Chainstack Documentation: Explore more about Chainstack's features and API capabilities in their Developer Portal.
-
Web3.py Documentation: For comprehensive details on interacting with Ethereum using Python, refer to the Web3.py documentation.
FAQs
Sign up, create a project, and deploy a node through the Chainstack platform.
Web3.py is a Python library used to interact with Ethereum-based blockchains.
Use environment variables or secure vaults instead of hardcoding keys in scripts.
Conclusion
By following this tutorial, you've set up a blockchain node using Chainstack and connected to it via Python. This setup allows you to interact with the blockchain, enabling functionalities such as querying data and sending transactions. For more advanced use cases, consider exploring Chainstack's API and additional services to enhance your blockchain development workflow.
We are Leapcell, your top choice for deploying Python projects to the cloud.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage โ no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead โ just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ