How to Integrate Momentum Strategy in Python: A Step-by-Step Guide
James Reed
Infrastructure Engineer · Leapcell
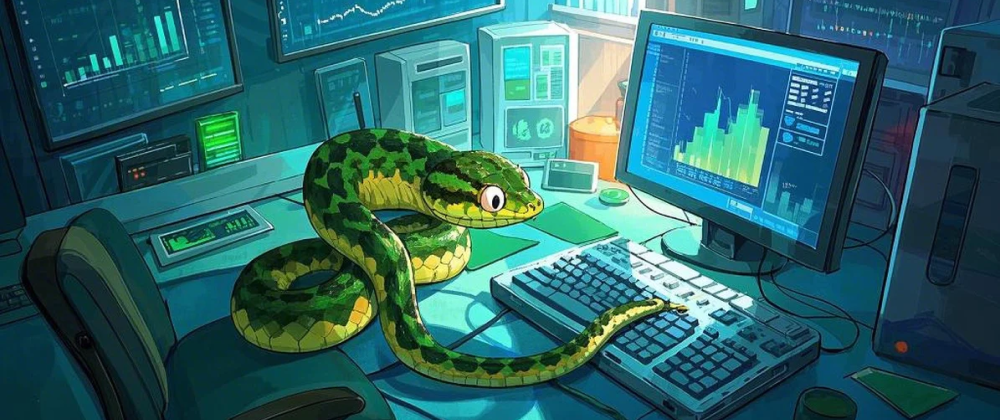
Momentum trading is a popular financial strategy that capitalizes on the continuance of an existing market trend. It involves buying assets when their prices are rising and selling when prices are falling. In this tutorial, we will demonstrate how to implement a simple momentum strategy in Python, using historical stock price data.
Key Takeaways
- Momentum trading involves capitalizing on the continuation of market trends using historical price data.
- Python libraries like
pandas
andnumpy
simplify momentum calculation and signal generation. - Backtesting is crucial to evaluate the performance of a momentum strategy.
Step 1: Setting Up the Environment
To begin, you need a Python environment with the necessary libraries installed. The key libraries for this task are:
pandas
: For data manipulationnumpy
: For numerical operationsmatplotlib
: For visualization
Install these libraries if you haven’t already:
pip install pandas numpy matplotlib
Step 2: Importing Required Libraries
Start by importing the necessary libraries:
import pandas as pd import numpy as np import matplotlib.pyplot as plt
Step 3: Loading Stock Price Data
You need historical stock price data to calculate momentum. For this example, let’s use synthetic data. In a real scenario, you can fetch data from sources like Yahoo Finance or Alpha Vantage.
# Generate synthetic stock price data np.random.seed(42) dates = pd.date_range(start='2020-01-01', periods=500) prices = pd.Series(np.cumprod(1 + np.random.normal(0, 0.01, len(dates))), index=dates)
If you have real data, load it using Pandas:
# Example for loading CSV data prices = pd.read_csv('your_data.csv', index_col='Date', parse_dates=True)['Close']
Step 4: Calculating Momentum
Momentum is calculated as the percentage change in price over a specified lookback period. For example, a 20-day momentum is computed as:
# Define lookback period lookback_period = 20 # Calculate momentum momentum = prices.pct_change(periods=lookback_period)
Step 5: Generating Trading Signals
Trading signals are generated based on the momentum values:
- Buy when momentum > 0
- Sell when momentum ≤ 0
# Generate trading signals signals = np.where(momentum > 0, 1, -1)
Step 6: Backtesting the Strategy
To evaluate the performance of the momentum strategy, compute the strategy’s returns and compare them to the market returns:
# Align signals with returns returns = prices.pct_change().iloc[1:] signals = signals[:-1] # Align dimensions # Calculate strategy returns strategy_returns = signals * returns.values # Calculate cumulative returns cumulative_strategy_returns = (1 + strategy_returns).cumprod() cumulative_market_returns = (1 + returns).cumprod()
Step 7: Visualizing the Results
Plot the cumulative returns of the momentum strategy against the market returns:
# Plot cumulative returns plt.figure(figsize=(12, 6)) plt.plot(cumulative_strategy_returns, label="Momentum Strategy") plt.plot(cumulative_market_returns, label="Market Returns", linestyle='--') plt.legend() plt.title("Momentum Strategy vs Market Returns") plt.xlabel("Date") plt.ylabel("Cumulative Returns") plt.grid(True) plt.show()
FAQs
Momentum indicates the trend direction, helping traders decide when to buy or sell.
Momentum is computed as the percentage change in price over a specified lookback period.
Yes, by replacing synthetic data with real historical data from sources like Yahoo Finance.
Conclusion
This tutorial demonstrates how to implement a basic momentum trading strategy in Python. While this is a simplified example, real-world applications can incorporate additional factors such as risk management, transaction costs, and more sophisticated momentum indicators like Relative Strength Index (RSI) or Moving Average Convergence Divergence (MACD).
By refining and testing your strategy on historical data, you can develop robust trading systems that may provide a competitive edge in the markets.
Happy coding and trading!
We are Leapcell, your top choice for deploying Python projects to the cloud.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ