How to Write Switch Statements in Python (2025) - Switch Case Example
James Reed
Infrastructure Engineer · Leapcell
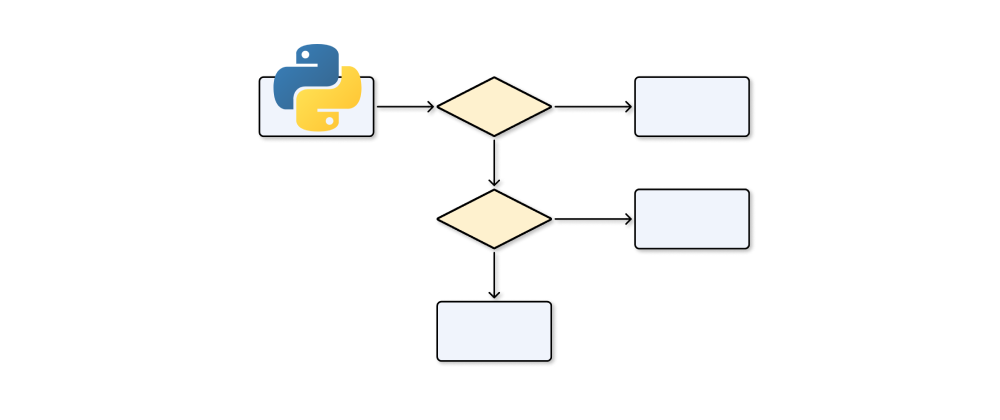
Introduction
In many programming languages, a switch statement is a control structure that allows the execution of one block of code out of many options based on the value of a variable or expression. While Python doesn’t have a built-in switch
statement like languages such as C, Java, or JavaScript, there are effective ways to mimic this functionality. With Python 3.10, the introduction of the match
statement provides an elegant and native solution for implementing switch-case behavior.
This tutorial explores traditional methods of mimicking switch statements and delves into the new match
statement.
Key Takeaways
- Python doesn’t have a native
switch
statement but offers several alternative approaches. - The
match
statement, introduced in Python 3.10, is the most Pythonic way to handle multiple cases. - For simple mappings, dictionaries remain an effective solution.
Traditional Methods
Before Python 3.10, developers often used dictionaries or if-elif-else
chains to mimic switch statements. Below are two common approaches:
1. Using Dictionaries
Dictionaries can store functions or values mapped to keys, effectively mimicking switch-case behavior.
# Example: Basic Switch using Dictionary def case_one(): return "Case 1 Executed" def case_two(): return "Case 2 Executed" def default_case(): return "Default Case" switch = { 1: case_one, 2: case_two } # Input value case_value = 1 # Execute the corresponding case result = switch.get(case_value, default_case)() print(result)
2. Using if-elif-else
Chains
An if-elif-else
chain is straightforward but can become cumbersome with many cases.
# Example: Basic Switch using if-elif-else case_value = 3 if case_value == 1: result = "Case 1 Executed" elif case_value == 2: result = "Case 2 Executed" else: result = "Default Case" print(result)
The Match Statement (Python 3.10+)
The match
statement, introduced in Python 3.10, provides a structured way to implement switch-case functionality. It supports pattern matching, making it more powerful than traditional switch statements in other languages.
Syntax
match variable: case pattern_1: # Code block case pattern_2: # Code block case _: # Default block
Example 1: Basic Match-Case
case_value = 2 match case_value: case 1: result = "Case 1 Executed" case 2: result = "Case 2 Executed" case _: result = "Default Case" print(result)
Example 2: Advanced Pattern Matching
Pattern matching allows for complex cases like matching data structures.
# Example: Matching Tuples data = ("action", 42) match data: case ("action", value) if value > 40: result = f"Action with value {value}" case ("action", value): result = f"Action with smaller value {value}" case _: result = "No match" print(result)
When to Use Each Method
- Dictionaries: Use when cases are simple and map directly to values or functions.
- If-Elif-Else: Use for small numbers of cases or when conditions involve complex expressions.
- Match Statement: Use for complex matching scenarios or when working with structured data (e.g., tuples, lists, dictionaries).
FAQs
The match
statement, introduced in Python 3.10, is a control structure for pattern matching, enabling clean and versatile case handling.
You can mimic a switch
statement using dictionaries, if-elif-else
chains, or the modern match
statement in Python 3.10+.
No, the match
statement is available only in Python 3.10 and later. For older versions, use dictionaries or if-elif-else
chains.
Conclusion
Python’s versatility allows multiple ways to implement switch-case behavior, ranging from dictionaries and if-elif-else
chains to the new and powerful match
statement. With Python 3.10 and later, the match
statement is the preferred and most Pythonic way to implement this functionality. By understanding the options available, you can choose the most appropriate approach for your project.
Additional Resources
We are Leapcell, your top choice for deploying Python projects to the cloud.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ