How to Delete a File in Golang
Grace Collins
Solutions Engineer · Leapcell
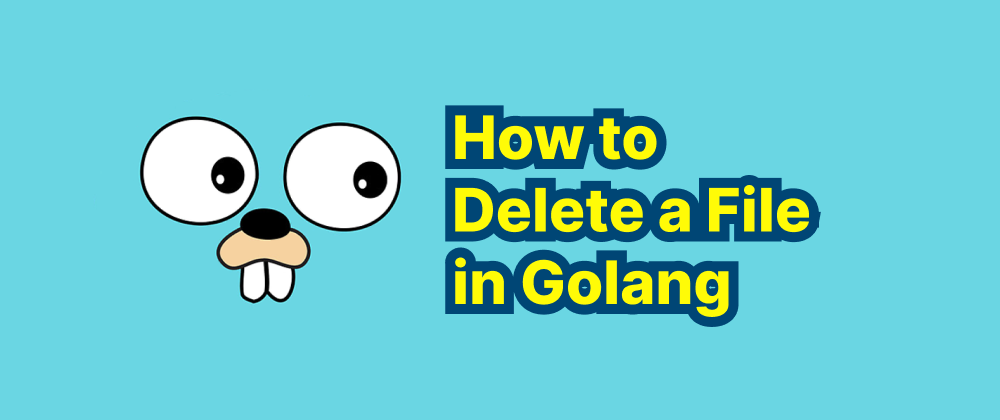
Key Takeaways
- Use
os.Remove
to delete files and empty directories. - Use
os.RemoveAll
to recursively delete directories and contents. - Always handle errors to manage missing files and permission issues.
Deleting a file is a common task in programming, and Golang provides a straightforward way to accomplish this using its standard library. This article will guide you through the process of deleting a file in Golang, handling potential errors, and considering best practices.
Using os.Remove
to Delete a File
Golang's os
package offers the Remove
function, which is designed to delete files and directories. To delete a file, you can use os.Remove
by passing the file's path as an argument. Here's a basic example:
package main import ( "fmt" "os" ) func main() { filePath := "example.txt" // Attempt to remove the file err := os.Remove(filePath) if err != nil { fmt.Println("Error deleting file:", err) return } fmt.Println("File successfully deleted") }
In this example:
- We import the necessary packages:
fmt
for formatted I/O operations andos
for file system operations. - We define the
filePath
variable with the path to the file we want to delete. - We call
os.Remove
withfilePath
as its argument. - If an error occurs during the deletion process,
os.Remove
returns an error, which we check and handle by printing an error message. - If no error occurs, we confirm that the file has been successfully deleted.
Handling Errors
It's crucial to handle errors when attempting to delete a file, as several issues can prevent successful deletion:
- File Does Not Exist: If the specified file doesn't exist,
os.Remove
will return an error. - Permission Denied: If the program lacks the necessary permissions to delete the file, an error will occur.
- File in Use: On some operating systems, attempting to delete a file that is currently in use by another process will result in an error.
To handle these scenarios gracefully, always check the error returned by os.Remove
and implement appropriate error-handling logic based on your application's requirements.
Deleting Directories
The os.Remove
function can also delete directories. However, it will only succeed if the directory is empty. Attempting to delete a non-empty directory with os.Remove
will result in an error. To delete a directory and its contents recursively, you can use the os.RemoveAll
function:
package main import ( "fmt" "os" ) func main() { dirPath := "example_directory" // Attempt to remove the directory and its contents err := os.RemoveAll(dirPath) if err != nil { fmt.Println("Error deleting directory:", err) return } fmt.Println("Directory and its contents successfully deleted") }
In this example, os.RemoveAll
is used to delete the specified directory and all of its contents, including subdirectories and files. As with file deletion, it's essential to handle any errors that may occur during this process.
Conclusion
Deleting files and directories in Golang is straightforward using the os.Remove
and os.RemoveAll
functions. Always ensure that you handle potential errors gracefully to make your application robust and reliable. Additionally, exercise caution when using os.RemoveAll
, as it will irreversibly delete all contents within the specified directory.
FAQs
os.Remove
returns an error if the file is missing.
No, use os.RemoveAll
to delete directories with contents.
Ensure the program has proper permissions or request elevated privileges.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ