Understanding Golang: Array vs. Slice
James Reed
Infrastructure Engineer · Leapcell
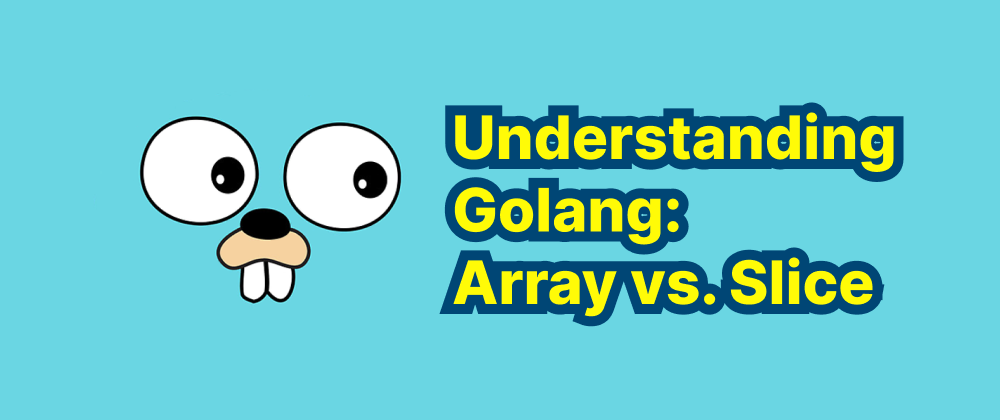
Key Takeaways
- Arrays in Go have a fixed size, while slices are dynamic and flexible.
- Slices are reference types, making them more memory-efficient than arrays.
- Slices provide powerful data manipulation capabilities without copying data.
In Go, arrays and slices are fundamental data structures used to store sequences of elements. While they may appear similar, they have distinct characteristics and behaviors that are crucial for developers to understand.
Arrays in Go
An array in Go is a fixed-size sequence of elements of the same type. Once an array is declared, its size cannot be changed. The syntax for declaring an array includes specifying its size:
var arr [5]int
In this example, arr
is an array of five integers. Arrays are value types in Go, meaning that assigning one array to another copies all its elements. Similarly, passing an array to a function results in copying the entire array. This behavior can impact performance, especially with large arrays.
Slices in Go
A slice is a more flexible, dynamic data structure that provides a window into an underlying array. Slices do not own the data they reference; instead, they describe a segment of an array. The syntax for declaring a slice is:
var s []int
Slices have three components:
- Pointer: References the underlying array's address.
- Length: The number of elements in the slice.
- Capacity: The number of elements in the underlying array, starting from the slice's first element.
Unlike arrays, slices are reference types. Assigning one slice to another or passing a slice to a function does not copy the underlying data; both slices refer to the same array segment. This behavior makes slices more efficient and versatile for dynamic data manipulation.
Creating Slices
Slices can be created in several ways:
-
From an existing array or slice:
a := [5]int{1, 2, 3, 4, 5} s := a[1:4] // s == []int{2, 3, 4}
-
Using the
make
function:s := make([]int, 5, 10)
This creates a slice of length 5 and capacity 10.
-
Slice literals:
s := []int{1, 2, 3}
This creates a slice with length and capacity 3.
Differences Between Arrays and Slices
-
Size: Arrays have a fixed size defined at compile-time, whereas slices are dynamic and can grow or shrink as needed.
-
Type: The size of an array is part of its type (
[3]int
is distinct from[4]int
), making arrays less flexible. Slices, however, do not include size in their type, enhancing flexibility. -
Memory Efficiency: Slices, being reference types, are more memory-efficient when passed to functions, as only the slice descriptor (pointer, length, capacity) is passed, not the entire data.
-
Use Cases: Arrays are suitable when the number of elements is known and fixed. Slices are preferable for dynamic or unknown numbers of elements, offering more flexibility and functionality.
Internal Mechanics of Slices
Understanding the internal mechanics of slices is essential for efficient Go programming. A slice is a descriptor containing a pointer to the underlying array, its length, and its capacity. When a slice is created from an array, it references a segment of that array:
a := [5]int{1, 2, 3, 4, 5} s := a[1:4]
Here, s
references elements {2, 3, 4}
of array a
. Modifying s
affects a
because they share the same underlying data. If the capacity of s
is exceeded (e.g., using append
), a new underlying array may be allocated, and the original array remains unchanged.
Conclusion
Arrays and slices are foundational to Go's data handling capabilities. Arrays provide a simple, fixed-size collection of elements, while slices offer dynamic, flexible views into arrays, enabling powerful data manipulation without the overhead of copying data. Understanding their differences and appropriate use cases is vital for writing efficient and effective Go programs.
FAQs
Slices offer dynamic resizing, better memory efficiency, and enhanced functionality.
No, slices are reference types, so they share the same underlying array.
A new underlying array is allocated, and the slice points to the new memory.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ