Understanding Closures in Go: Capturing Variables for Flexible Functions
James Reed
Infrastructure Engineer · Leapcell
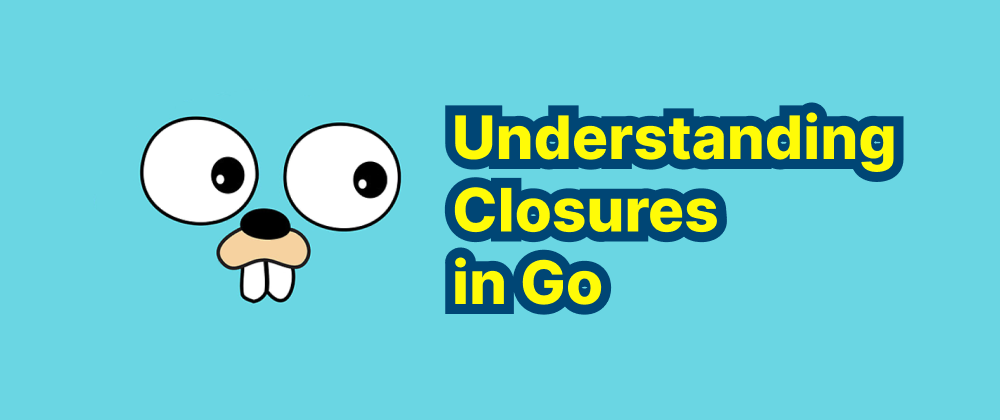
Key Takeaways
- Closures in Go capture variables from their surrounding scope, allowing flexible function behavior.
- They enable encapsulation, callbacks, and function factories for dynamic code.
- Be mindful of variable capture by reference and concurrency issues.
In Go, a closure is a function that captures variables from its surrounding lexical scope, allowing the function to access those variables even when invoked outside their original context. This feature enables the creation of more flexible and modular code.
Understanding Closures in Go
In Go, functions are first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions. When a function references variables from its surrounding scope, it forms a closure, capturing those variables for later use.
Here's a simple example to illustrate this concept:
package main import "fmt" func main() { // Define a local variable message := "Hello, World!" // Define a function literal (anonymous function) that captures 'message' greet := func() { fmt.Println(message) } // Invoke the closure greet() }
In this example, the anonymous function assigned to greet
captures the message
variable from its surrounding scope. When greet()
is called, it accesses and prints the message
, demonstrating how closures can retain access to variables even after their original scope has ended.
Practical Use Cases for Closures
Closures are particularly useful in scenarios such as:
-
Encapsulation: They allow encapsulation of state within a function, providing a way to hide and manage internal variables.
-
Callbacks and Higher-Order Functions: Closures can be passed as arguments to other functions, enabling callback mechanisms and the creation of higher-order functions.
-
Function Factories: They enable the creation of function factories—functions that return other functions with specific behaviors based on the captured variables.
Example: Function Factory
Consider a function that generates multiplier functions:
package main import "fmt" // multiplier returns a closure that multiplies its input by 'n' func multiplier(n int) func(int) int { return func(x int) int { return x * n } } func main() { double := multiplier(2) triple := multiplier(3) fmt.Println(double(5)) // Output: 10 fmt.Println(triple(5)) // Output: 15 }
In this example, the multiplier
function returns a closure that captures the variable n
. The returned function multiplies its input by n
, demonstrating how closures can be used to create specialized functions with retained state.
Key Considerations
-
Variable Capture: Closures capture variables by reference, not by value. If the captured variable changes after the closure is defined, the closure reflects those changes.
-
Concurrency: When using closures in concurrent programming, be cautious of shared variable access to avoid race conditions. Proper synchronization mechanisms, like mutexes, may be necessary.
Understanding and utilizing closures effectively can lead to more concise and expressive Go code, enabling patterns that manage state and behavior in a functional programming style.
FAQs
A closure is a function that captures and retains access to variables from its surrounding scope.
Closures help with state encapsulation, callbacks, and generating functions dynamically.
By reference, meaning changes to the captured variable affect the closure's execution.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ