Understanding Dictionaries in Go: The `map` Data Structure
James Reed
Infrastructure Engineer · Leapcell
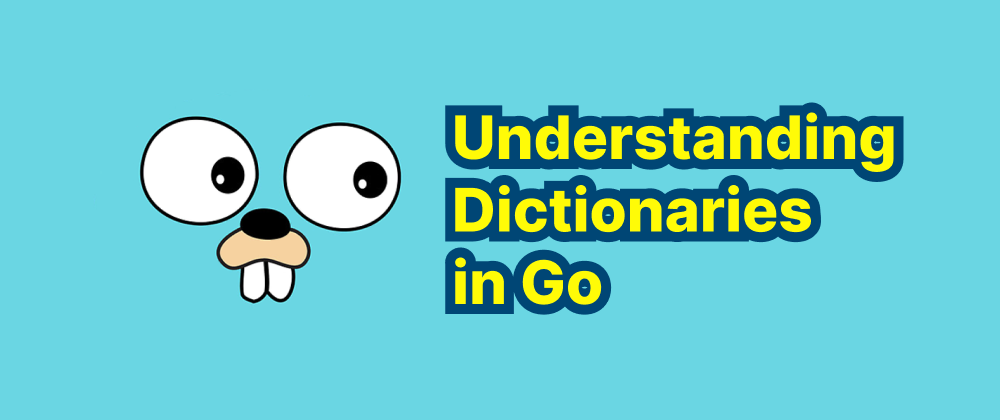
Key Takeaways
- Go's
map
type provides an efficient way to store and retrieve key-value pairs. - Maps require initialization before use to avoid runtime panics.
- Iteration order in Go maps is unpredictable.
In programming, a dictionary is a data structure that stores key-value pairs, allowing for efficient data retrieval based on unique keys. In Go, this functionality is provided by the built-in map
type.
Declaring and Initializing Maps
A map in Go is declared using the following syntax:
var m map[KeyType]ValueType
Here, KeyType
is the type of the keys, and ValueType
is the type of the values. For example, to create a map with string keys and integer values:
var m map[string]int
However, declaring a map this way initializes it to nil
. Attempting to add elements to a nil map will cause a runtime panic. Therefore, it's essential to initialize the map before use. This can be done using the make
function:
m = make(map[string]int)
Alternatively, you can declare and initialize a map with values using a map literal:
m := map[string]int{ "Alice": 30, "Bob": 25, }
Adding and Accessing Elements
To add or update elements in a map:
m["Charlie"] = 35
To retrieve a value:
age := m["Alice"]
If the key doesn't exist, m["NonExistentKey"]
returns the zero value of the map's value type. For an int
map, this would be 0
.
Checking for Key Existence
To determine if a key exists in a map and retrieve its value:
age, exists := m["Alice"] if exists { // Key exists, use 'age' } else { // Key does not exist }
Deleting Elements
To remove a key-value pair from a map:
delete(m, "Bob")
Using delete
on a non-existent key does not cause an error.
Iterating Over a Map
You can iterate over all key-value pairs in a map using a for
loop with the range
keyword:
for key, value := range m { fmt.Println(key, value) }
The iteration order over map elements is not specified and can vary.
Maps as Sets
Since map lookups return the zero value for missing keys, you can use a map as a set. For example, to track visited nodes:
visited := make(map[string]bool) visited["A"] = true if visited["B"] { // Node B has been visited } else { // Node B has not been visited }
In this scenario, visited["B"]
returns false
, indicating that node B has not been visited.
Nested Maps
Go supports nested maps, allowing you to create complex data structures. For example:
nestedMap := make(map[string]map[string]int) nestedMap["Alice"] = map[string]int{"Math": 90, "Science": 85}
When accessing or assigning values in nested maps, ensure that the inner map is initialized to avoid runtime panics.
Conclusion
The map
type in Go is a powerful and flexible data structure for storing and managing key-value pairs. By understanding its declaration, initialization, and operations, you can effectively utilize maps in your Go programs.
For more detailed information, refer to the official Go blog on maps: Go maps in action
FAQs
Use the syntax value, exists := m[key]
, where exists
is a boolean indicating key presence.
It returns the zero value of the map's value type (e.g., 0
for int
, ""
for string
).
Yes, by using map[Type]bool
, where true
indicates the presence of an element.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ