Understanding Classes in Go
James Reed
Infrastructure Engineer · Leapcell
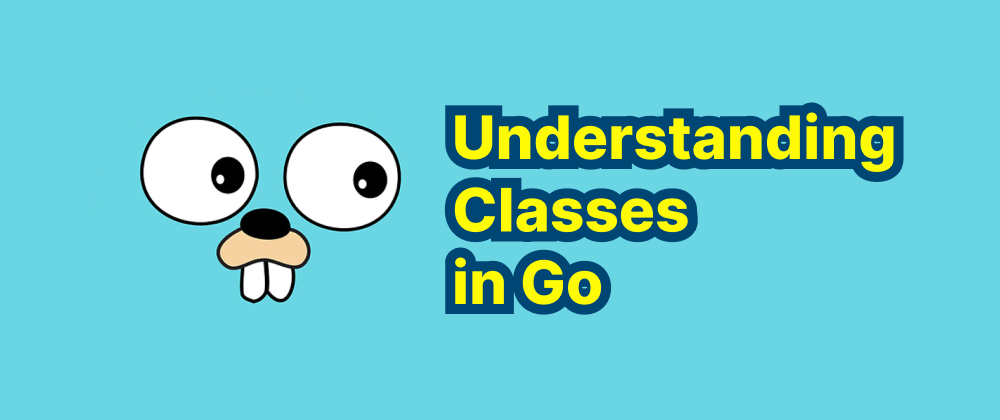
Key Takeaways
- Go does not have traditional classes but uses structs and methods to structure data and behavior.
- Interfaces in Go define behavior without enforcing a specific data structure.
- Go promotes composition over inheritance for code reuse and maintainability.
Go, often referred to as Golang, is a statically typed, compiled programming language designed for simplicity and efficiency. One common question among developers transitioning to Go is how the language handles classes, a fundamental concept in many object-oriented languages.
Absence of Classes in Go
Unlike traditional object-oriented languages such as Java or C++, Go does not have classes. This design choice aligns with Go's philosophy of simplicity and clarity. Instead of classes, Go provides other constructs to achieve similar functionality.
Structs: The Building Blocks
In Go, the primary construct for grouping data is the struct. A struct allows you to combine data fields, known as fields, into a single entity. Here's an example:
type Person struct { Name string Age int }
In this example, Person
is a struct with two fields: Name
and Age
.
Methods: Associating Functions with Structs
While Go doesn't have classes, it allows you to define methods on types, including structs. This feature enables you to associate behavior with data. For instance:
func (p Person) Greet() string { return fmt.Sprintf("Hello, my name is %s and I am %d years old.", p.Name, p.Age) }
Here, Greet
is a method associated with the Person
struct. It behaves similarly to methods in class-based languages.
Interfaces: Defining Behavior
Go's interfaces provide a way to define behavior without specifying data structures. An interface is a set of method signatures that a type must implement. For example:
type Greeter interface { Greet() string }
Any type that implements the Greet
method satisfies the Greeter
interface. This approach allows for flexible and modular code design.
Composition Over Inheritance
Instead of class-based inheritance, Go promotes composition. You can embed structs within other structs to reuse code:
type Employee struct { Person Position string }
In this example, Employee
includes all fields and methods of Person
, promoting code reuse without the complexities of inheritance hierarchies.
Conclusion
While Go does not have classes in the traditional sense, it offers powerful features like structs, methods, and interfaces to structure code effectively. By embracing composition over inheritance, Go encourages a clean and maintainable approach to software design.
FAQs
Go favors simplicity and uses structs and interfaces instead of class-based inheritance.
You can define methods with a receiver of the struct type to associate functions with it.
Go uses composition, where one struct can embed another to reuse its fields and methods.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ