Understanding Golang's Garbage Collector
James Reed
Infrastructure Engineer · Leapcell
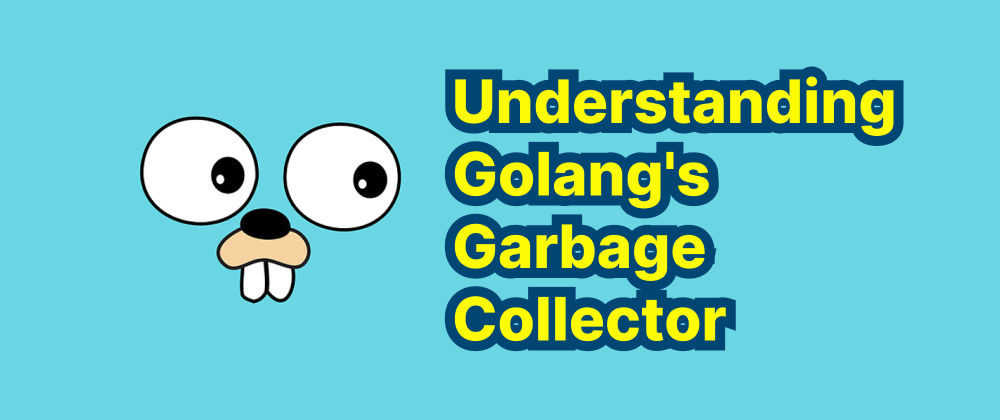
Key Takeaways
- Go's garbage collector is concurrent, non-generational, and non-compacting to optimize performance.
- It uses a mark-and-sweep algorithm with a three-color abstraction for efficient memory management.
- Write barriers help maintain memory integrity during concurrent execution.
The Go programming language, commonly known as Golang, incorporates an automatic memory management system known as the Garbage Collector (GC). The primary function of the GC is to identify and reclaim memory that is no longer in use by the program, thereby preventing memory leaks and optimizing available memory resources.
Design Principles of Go's Garbage Collector
Go's GC is characterized by three main design principles:
-
Non-generational: Unlike some languages that categorize objects based on their lifespan (e.g., young or old generations), Go treats all objects uniformly without such distinctions.
-
Non-compacting: During the garbage collection process, Go's GC does not relocate objects in memory. This approach avoids the overhead associated with moving objects and updating references.
-
Concurrent: Go's GC operates concurrently with the application code, allowing the program to continue running while garbage collection occurs, thereby minimizing pause times.
These design choices are influenced by Go's memory allocation strategy, which is based on tcmalloc
. This allocator effectively manages memory fragmentation, reducing the necessity for object compaction. Additionally, Go's compiler employs escape analysis to determine whether variables can be allocated on the stack, further optimizing memory management.
Mark-and-Sweep Algorithm
Go's GC utilizes the mark-and-sweep algorithm, a common technique in garbage collection. The process can be divided into two primary phases:
-
Mark Phase: Starting from a set of root objects (such as global variables and active stack frames), the GC traverses all reachable objects, marking them as alive.
-
Sweep Phase: The GC then scans the heap, identifying unmarked objects (those not reached during the mark phase) and reclaiming their memory for future allocations.
This method ensures that memory occupied by unreachable objects is efficiently reclaimed without necessitating the relocation of live objects.
Three-Color Abstraction
To facilitate concurrent garbage collection, Go's GC employs the three-color abstraction, categorizing objects into three distinct sets:
-
White: Objects that have not been examined by the GC and are considered candidates for reclamation.
-
Gray: Objects that have been identified by the GC but whose references have not yet been fully explored.
-
Black: Objects that have been fully processed by the GC, including all objects they reference.
The GC initiates by marking all objects as white. It then moves root objects to the gray set and begins processing them. As each gray object is processed, it is moved to the black set, and any white objects it references are moved to the gray set. This cycle continues until no gray objects remain. At this point, all remaining white objects are deemed unreachable and are subsequently reclaimed.
Write Barriers
During the concurrent marking phase, the program (mutator) may modify object references, potentially leading to scenarios where reachable objects are mistakenly identified as unreachable. To address this, Go's GC implements a mechanism known as write barriers.
A write barrier is a small piece of code that executes before or after a pointer in the program is modified. Its purpose is to maintain the integrity of the three-color invariant by ensuring that any changes to object references during the marking phase do not result in the premature reclamation of live objects.
Advantages of Go's Garbage Collection Approach
Go's GC design offers several benefits:
-
Reduced Pause Times: By operating concurrently with the application, the GC minimizes stop-the-world (STW) events, leading to smoother application performance.
-
Simplified Memory Management: Developers are relieved from manual memory management tasks, reducing the likelihood of memory leaks and related bugs.
-
Efficient Memory Utilization: The non-compacting nature of the GC, combined with Go's memory allocator, ensures efficient use of memory without significant fragmentation.
Conclusion
Go's garbage collector is a sophisticated system designed to manage memory efficiently while minimizing disruptions to program execution. Its concurrent, non-generational, and non-compacting design, along with the use of the mark-and-sweep algorithm and three-color abstraction, ensures that Go applications can run smoothly without the developer needing to manage memory manually.
FAQs
It operates concurrently with the application, reducing stop-the-world events.
It categorizes objects to efficiently mark and sweep unreachable memory.
Go relies on tcmalloc
, which reduces fragmentation and removes the need for compaction.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ