How to Check if an Array Contains a Specific Element in Go
James Reed
Infrastructure Engineer · Leapcell
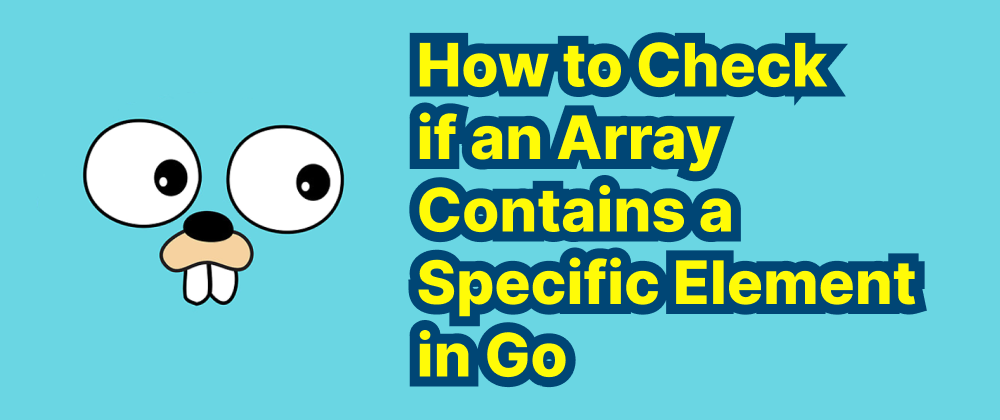
Key Takeaways
- Iterating over a slice is the simplest way to check for an element.
- Using a map provides faster lookups for frequent checks.
- Arrays are fixed-size, while slices are more flexible in Go.
In Go, arrays have a fixed size and are not commonly used in situations where the size of the collection can change. Instead, slices, which are dynamically-sized, are more commonly used. To check if a slice contains a specific element, you can iterate over the slice and compare each element with the target value.
Here's an example of how to do this:
package main import "fmt" func contains(slice []int, element int) bool { for _, v := range slice { if v == element { return true } } return false } func main() { numbers := []int{1, 2, 3, 4, 5} fmt.Println(contains(numbers, 3)) // Output: true fmt.Println(contains(numbers, 6)) // Output: false }
In this example, the contains
function iterates over each element in the slice. If it finds an element that matches the target value, it returns true
. If it completes the loop without finding a match, it returns false
.
This approach works for slices of any type. For example, to check for a string in a slice of strings, you can modify the function as follows:
package main import "fmt" func contains(slice []string, element string) bool { for _, v := range slice { if v == element { return true } } return false } func main() { words := []string{"apple", "banana", "cherry"} fmt.Println(contains(words, "banana")) // Output: true fmt.Println(contains(words, "date")) // Output: false }
If you need to perform this check frequently or on large slices, you might consider using a map for faster lookups, as map lookups have average-case constant time complexity. Here's how you can do that:
package main import "fmt" func main() { elements := map[int]struct{}{ 1: {}, 2: {}, 3: {}, 4: {}, 5: {}, } _, exists := elements[3] fmt.Println(exists) // Output: true _, exists = elements[6] fmt.Println(exists) // Output: false }
In this example, we use a map with keys of the element type and empty struct values. The empty struct occupies zero bytes, making it a memory-efficient choice when you don't need to store additional information.
Remember, while arrays are part of Go's type system, slices are more commonly used due to their flexibility and dynamic sizing.
FAQs
Slices in Go do not have built-in containment checks, so iteration is a simple and direct approach.
Use a map when you need fast lookups, as map operations are more efficient than iterating over large slices.
Yes, you can modify the function to work with different types like string
or float64
.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ