Understanding Python's `replace()` Method for String Manipulation
Grace Collins
Solutions Engineer · Leapcell
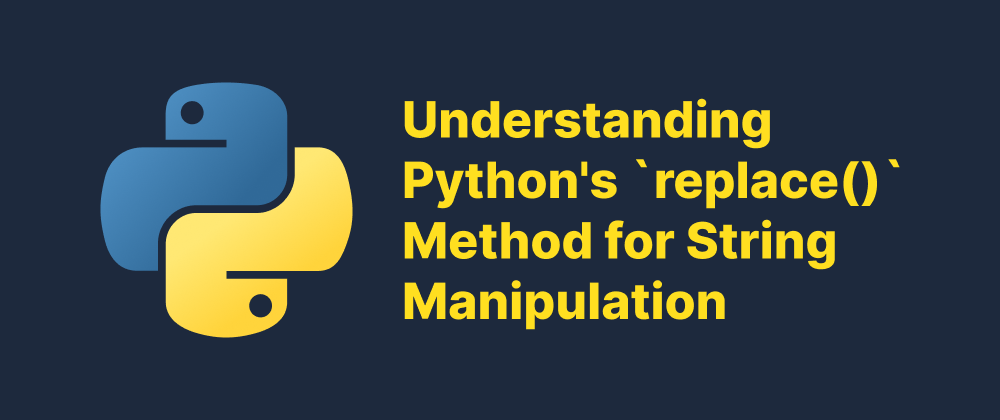
Key Takeaways
- The
replace()
method substitutes a target substring with another in a string. - It is case-sensitive and returns a new string.
- An optional
count
parameter limits how many replacements occur.
In Python, string manipulation is a fundamental aspect of programming, and the replace()
method stands out as a particularly useful tool. This method allows developers to substitute occurrences of a specified substring with another substring, facilitating tasks such as data cleaning, text processing, and content modification.
Syntax of the replace()
Method
The replace()
method is invoked on a string object and follows this syntax:
new_string = original_string.replace(old, new[, count])
original_string
: The initial string where the replacement will occur.old
: The substring that you want to replace.new
: The substring that will replace theold
substring.count
(optional): Specifies the maximum number of occurrences to replace. If omitted, all occurrences are replaced.
Basic Usage
Consider a simple example where we replace all instances of the word "apples" with "oranges":
text = "I love apples. Apples are my favorite fruit." updated_text = text.replace("apples", "oranges") print(updated_text)
Output:
I love oranges. Apples are my favorite fruit.
In this example, only the lowercase "apples" is replaced. The replace()
method is case-sensitive, so "Apples" remains unchanged.
Using the count
Parameter
The count
parameter limits the number of replacements. For instance, to replace only the first occurrence of "is" with "was":
sentence = "This is a test. This is only a test." updated_sentence = sentence.replace("is", "was", 1) print(updated_sentence)
Output:
Thwas is a test. This is only a test.
Here, only the first "is" is replaced, demonstrating how the count
parameter controls the scope of the replacement.
Important Considerations
-
Immutability of Strings: Strings in Python are immutable, meaning that methods like
replace()
do not alter the original string but instead return a new string with the desired modifications. Therefore, it's essential to assign the result to a new variable or overwrite the original one if you wish to retain the changes. -
Case Sensitivity: As noted earlier,
replace()
is case-sensitive. To perform case-insensitive replacements, you might need to use regular expressions with there
module or convert the string to a consistent case before performing replacements.
Advanced Usage with Regular Expressions
For more complex pattern matching and replacements, Python's re
module can be utilized. The re.sub()
function allows for pattern-based substitutions:
import re text = "The price is $100. Special offer: $80!" updated_text = re.sub(r'\$\d+', '$90', text) print(updated_text)
Output:
The price is $90. Special offer: $90!
In this example, re.sub()
replaces all dollar amounts with "$90", showcasing the power of regular expressions for pattern-based replacements.
Conclusion
The replace()
method is a straightforward yet powerful tool for string manipulation in Python. Its ability to replace specified substrings with new values makes it indispensable for tasks involving text modification. By understanding its syntax, parameters, and behavior, developers can effectively utilize replace()
to handle a wide range of string manipulation tasks.
FAQs
No, it returns a new string because strings in Python are immutable.
Yes, it only replaces substrings that exactly match the case of the target.
Use the re.sub()
function from Python's re
module with appropriate flags.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ