Functional Programming in Go: Concepts and Applications
Grace Collins
Solutions Engineer · Leapcell
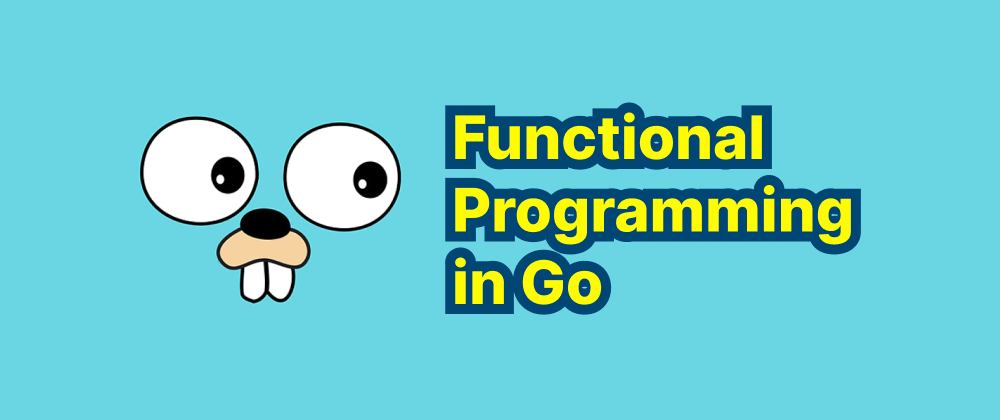
Key Takeaways
- Go supports functional programming through first-class functions, closures, and higher-order functions.
- Functional techniques like immutability, map, filter, and reduce improve code readability and maintainability.
- Libraries like
fp-go
andgo-functional
enhance Go's functional programming capabilities.
Functional programming (FP) is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing state or mutable data. While Go is primarily an imperative language, it offers features that enable developers to apply functional programming techniques, leading to more concise, maintainable, and testable code.
First-Class Functions
In Go, functions are first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions. This capability allows for the creation of higher-order functions—functions that operate on other functions.
package main import "fmt" // applyFunc applies a given function to two integers. func applyFunc(a, b int, fn func(int, int) int) int { return fn(a, b) } func main() { sum := func(x, y int) int { return x + y } product := func(x, y int) int { return x * y } fmt.Println(applyFunc(3, 4, sum)) // Output: 7 fmt.Println(applyFunc(3, 4, product)) // Output: 12 }
In this example, applyFunc
takes a function fn
as an argument and applies it to the integers a
and b
. This demonstrates Go's support for higher-order functions.
Anonymous Functions and Closures
Go supports anonymous functions, which are functions without a name, often used for short operations or closures—functions that reference variables from outside their body.
package main import "fmt" func main() { increment := func() func() int { i := 0 return func() int { i++ return i } }() fmt.Println(increment()) // Output: 1 fmt.Println(increment()) // Output: 2 }
Here, the anonymous function forms a closure over the variable i
, maintaining its state between calls.
Immutability
While Go does not enforce immutability, developers can adopt practices to emulate it, such as returning new values instead of modifying existing ones.
package main import "fmt" // addElement returns a new slice with the added element. func addElement(s []int, e int) []int { newSlice := make([]int, len(s)+1) copy(newSlice, s) newSlice[len(s)] = e return newSlice } func main() { original := []int{1, 2, 3} updated := addElement(original, 4) fmt.Println(original) // Output: [1 2 3] fmt.Println(updated) // Output: [1 2 3 4] }
This approach ensures that the original data remains unchanged, promoting immutability.
Functional Libraries in Go
Several libraries facilitate functional programming in Go:
-
fp-go: Inspired by the
fp-ts
library in TypeScript,fp-go
provides data types and functions to write maintainable and testable code in Go. It encourages writing small, pure functions and offers helpers to isolate side effects into lazily executed functions. citeturn0search3 -
go-functional: This library offers iterators and consumers to augment Go's slices and maps packages, enabling functional operations like mapping and filtering over collections. citeturn0search7
Practical Examples
Map, Filter, and Reduce
Implementing functional operations like map
, filter
, and reduce
can lead to more expressive code.
package main import "fmt" // Map applies a function to each element of a slice. func Map[T any](s []T, fn func(T) T) []T { result := make([]T, len(s)) for i, v := range s { result[i] = fn(v) } return result } // Filter returns a new slice containing elements that satisfy a predicate. func Filter[T any](s []T, fn func(T) bool) []T { result := []T{} for _, v := range s { if fn(v) { result = append(result, v) } } return result } // Reduce aggregates elements of a slice using a function. func Reduce[T any](s []T, fn func(T, T) T, initial T) T { result := initial for _, v := range s { result = fn(result, v) } return result } func main() { numbers := []int{1, 2, 3, 4, 5} // Double each number. doubled := Map(numbers, func(n int) int { return n * 2 }) fmt.Println(doubled) // Output: [2 4 6 8 10] // Filter out odd numbers. even := Filter(numbers, func(n int) bool { return n%2 == 0 }) fmt.Println(even) // Output: [2 4] // Sum all numbers. sum := Reduce(numbers, func(a, b int) int { return a + b }, 0) fmt.Println(sum) // Output: 15 }
These functions—Map
, Filter
, and Reduce
—demonstrate how functional programming concepts can be applied in Go to process collections in a declarative manner.
Conclusion
While Go is not a purely functional language, it provides features that allow developers to incorporate functional programming techniques. By leveraging first-class functions, closures, and higher-order functions, Go developers can write code that is modular, testable, and expressive. Additionally, community-contributed libraries further enhance Go's functional programming capabilities, offering tools and abstractions that align with functional paradigms.
FAQs
Go is primarily imperative but provides functional features like first-class functions and closures.
By avoiding in-place modifications and using functions that return new values instead of altering existing ones.
Higher-order functions, closures, and operations like map, filter, and reduce.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ