Understanding Struct Embedding in Go
James Reed
Infrastructure Engineer · Leapcell
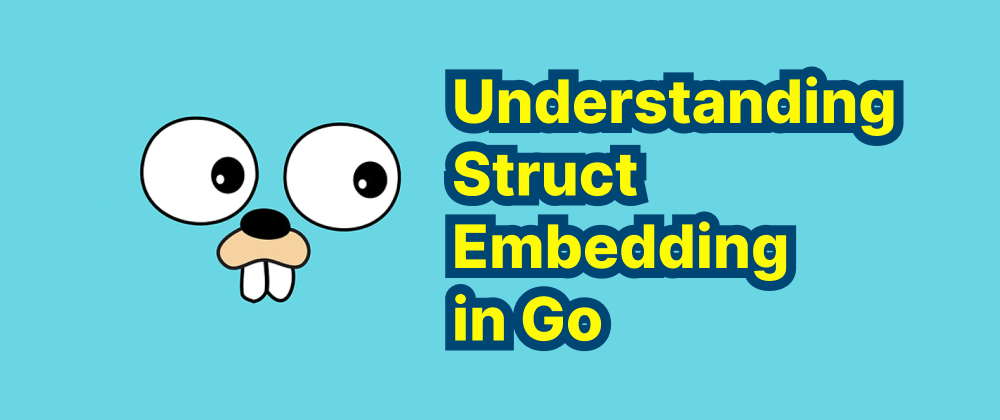
Key Takeaways
- Struct embedding allows for field and method promotion, enabling code reuse without traditional inheritance.
- Methods of embedded structs can be accessed directly and even overridden in the outer struct.
- Embedding promotes Go’s composition-over-inheritance philosophy for flexible and maintainable code.
In Go programming, struct embedding is a powerful feature that allows developers to compose types in a flexible and reusable manner. By embedding one struct within another, you can promote the fields and methods of the embedded struct to the outer struct, facilitating code reuse and the creation of more complex data structures.
What is Struct Embedding?
Struct embedding in Go is a form of composition where one struct is included within another. Unlike traditional inheritance found in other programming languages, Go's embedding promotes the fields and methods of the embedded struct to the containing struct. This means that the outer struct can directly access the embedded struct's fields and methods as if they were its own.
How to Embed Structs in Go
To embed a struct within another struct, simply declare the embedded struct type without a field name in the outer struct. Here's an example:
package main import "fmt" // Define a base struct type Person struct { Name string Age int } // Define another struct that embeds Person type Employee struct { Person EmployeeID string } func main() { // Initialize an Employee instance e := Employee{ Person: Person{ Name: "Alice", Age: 30, }, EmployeeID: "E12345", } // Access fields from the embedded Person struct fmt.Println("Name:", e.Name) // Output: Name: Alice fmt.Println("Age:", e.Age) // Output: Age: 30 fmt.Println("Employee ID:", e.EmployeeID) // Output: Employee ID: E12345 }
In this example, the Employee
struct embeds the Person
struct. As a result, Employee
inherits the fields Name
and Age
from Person
, allowing direct access to these fields through an Employee
instance.
Method Promotion with Embedded Structs
Embedding also promotes the methods of the embedded struct to the outer struct. This allows the outer struct to call methods defined on the embedded struct without explicit delegation. Consider the following example:
package main import "fmt" // Define a base struct with a method type Person struct { Name string } func (p Person) Greet() { fmt.Println("Hello, my name is", p.Name) } // Define another struct that embeds Person type Employee struct { Person EmployeeID string } func main() { // Initialize an Employee instance e := Employee{ Person: Person{ Name: "Bob", }, EmployeeID: "E67890", } // Call the Greet method from the embedded Person struct e.Greet() // Output: Hello, my name is Bob }
Here, the Greet
method defined on the Person
struct is promoted to the Employee
struct through embedding. This allows an Employee
instance to call the Greet
method directly.
Overriding Embedded Methods
If the outer struct defines a method with the same name as one in the embedded struct, the outer struct's method overrides the embedded one. Here's an example:
package main import "fmt" // Define a base struct with a method type Person struct { Name string } func (p Person) Greet() { fmt.Println("Hello, my name is", p.Name) } // Define another struct that embeds Person and overrides Greet type Employee struct { Person EmployeeID string } func (e Employee) Greet() { fmt.Println("Hello, I am employee", e.EmployeeID) } func main() { // Initialize an Employee instance e := Employee{ Person: Person{ Name: "Charlie", }, EmployeeID: "E54321", } // Call the overridden Greet method e.Greet() // Output: Hello, I am employee E54321 // Call the original Greet method from Person e.Person.Greet() // Output: Hello, my name is Charlie }
In this case, the Employee
struct defines its own Greet
method, which overrides the Greet
method from the embedded Person
struct. However, you can still access the original Greet
method by qualifying it with the embedded struct's name (e.Person.Greet()
).
Conclusion
Struct embedding in Go provides a mechanism for composing types that promotes code reuse and flexibility. By embedding structs, you can create complex data structures that inherit fields and methods from other structs without the need for explicit inheritance. This feature aligns with Go's design philosophy of simplicity and composability, allowing developers to build robust and maintainable applications.
FAQs
Struct embedding is a way to include one struct within another, allowing field and method promotion.
Yes, the outer struct can define a method with the same name, overriding the embedded one.
Use the embedded struct's name, e.g., e.Person.Greet()
, to call its original method.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ