Understanding Golang Ticker: A Guide to Timed Operations
James Reed
Infrastructure Engineer · Leapcell
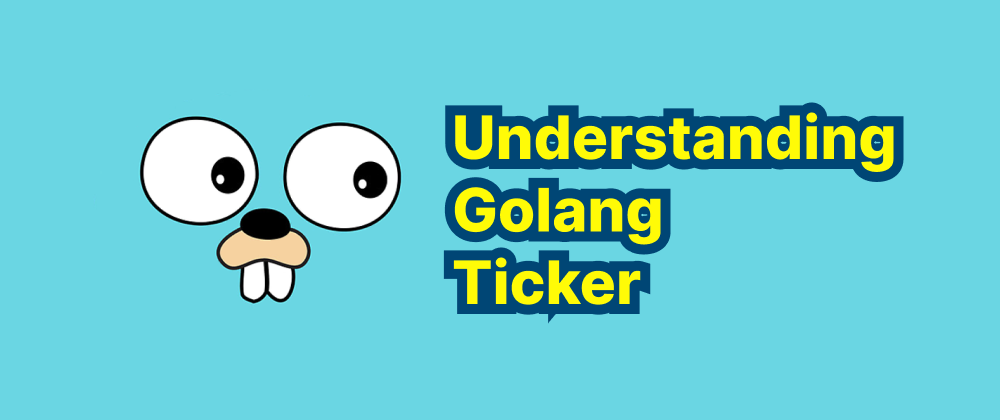
Key Takeaways
time.Ticker
enables periodic task execution in Golang by sending time events at fixed intervals.- Properly stopping a ticker using
ticker.Stop()
is essential to avoid resource leaks. Ticker
differs fromTimer
by continuously emitting events, whereasTimer
triggers only once.
Golang’s time.Ticker
is a useful tool for executing periodic tasks at fixed intervals. Unlike time.After
, which triggers a one-time delay, time.Ticker
continuously sends signals at regular intervals, making it ideal for scenarios like polling, monitoring, or scheduling repeated executions.
What Is a Golang Ticker?
A time.Ticker
is a struct from the time
package that delivers time events on a channel at a specified interval. It helps developers run code periodically without writing complex loops or manually handling timing logic.
The ticker works by sending the current time to its associated channel after every tick. This allows the receiving goroutine to execute tasks in response.
Creating and Using a Ticker
Here’s a simple example of using a time.Ticker
in Golang:
package main import ( "fmt" "time" ) func main() { ticker := time.NewTicker(2 * time.Second) // Create a ticker that ticks every 2 seconds defer ticker.Stop() // Ensure the ticker stops when done done := make(chan bool) go func() { for { select { case <-done: return case t := <-ticker.C: fmt.Println("Tick at", t) } } }() time.Sleep(10 * time.Second) // Let the ticker run for 10 seconds done <- true }
Explanation:
time.NewTicker(duration)
: Creates a ticker that emits values at regular intervals.ticker.C
: The ticker’s channel sends the current time at each tick.- Goroutine and
select
: A separate goroutine listens for ticker signals and performs a task. - Stopping the ticker:
ticker.Stop()
should always be called when the ticker is no longer needed to prevent memory leaks.
Common Use Cases
1. Periodic Logging
ticker := time.NewTicker(5 * time.Second) for t := range ticker.C { fmt.Println("Logging data at:", t) }
This pattern is useful for logging system status at fixed intervals.
2. API Polling
ticker := time.NewTicker(1 * time.Minute) defer ticker.Stop() for range ticker.C { checkAPIStatus() }
Here, checkAPIStatus()
is a function that fetches updates from an API every minute.
3. Background Tasks
ticker := time.NewTicker(30 * time.Second) go func() { for range ticker.C { cleanupOldFiles() } }()
This example runs cleanupOldFiles()
every 30 seconds in the background.
Stopping a Ticker
It is crucial to stop a ticker when it's no longer needed. Otherwise, it continues running indefinitely, consuming resources.
Example:
ticker := time.NewTicker(10 * time.Second) time.Sleep(30 * time.Second) ticker.Stop()
After stopping, the ticker no longer emits values, and its channel is not closed. Attempting to read from it after stopping results in a deadlock.
Difference Between Ticker
and Timer
Golang’s time.Timer
is another timing mechanism, but it differs from time.Ticker
:
Feature | time.Ticker | time.Timer |
---|---|---|
Execution | Repeats indefinitely | Fires once |
Use case | Periodic execution | Delayed execution |
Stopping | ticker.Stop() | timer.Stop() |
Conclusion
Golang’s time.Ticker
is a powerful tool for scheduling periodic tasks efficiently. Whether it’s logging, polling, or background job execution, Ticker
simplifies time-based execution while ensuring proper resource management through Stop()
.
By understanding how Ticker
works and when to use it, developers can build robust and efficient applications in Golang.
FAQs
Use time.NewTicker(duration)
to create a ticker that sends time events at the specified interval.
Call ticker.Stop()
to release resources and stop the ticker.
Ticker
emits repeated events, while Timer
triggers once after a delay.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ