Understanding the 'break' Statement in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
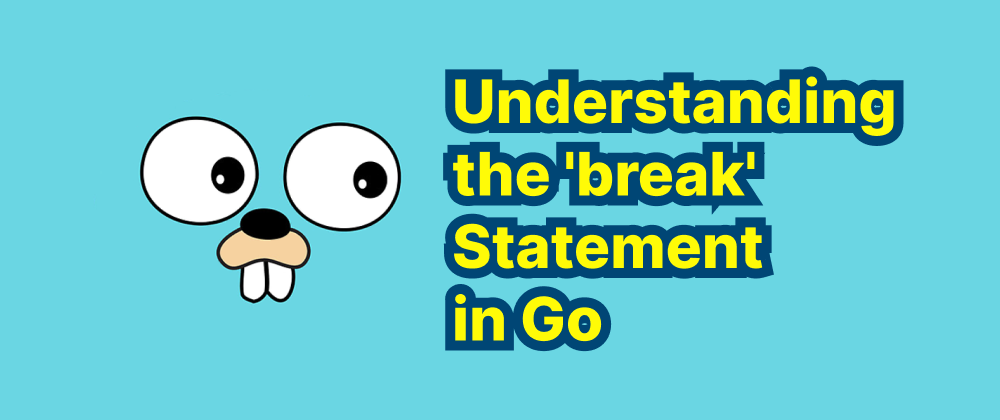
Key Takeaways
- The
break
statement is used to exit loops or switch statements prematurely in Go. - Labeled
break
allows for exiting specific nested loops. - In
switch
statements,break
is optional but can enhance clarity.
The break
statement in Go is a control flow mechanism that allows developers to exit loops or switch statements prematurely. This article delves into the syntax, usage, and practical examples of the break
statement in Go.
Syntax of the 'break' Statement
In Go, the break
statement is straightforward and does not require any additional parameters. Its primary function is to terminate the nearest enclosing loop or switch statement. The basic syntax is:
break
Using 'break' in Loops
The break
statement is commonly used within loops to exit before the loop's natural termination condition is met. This can be particularly useful when a specific condition is satisfied, and continuing the loop is unnecessary.
Example: Exiting a 'for' Loop
Consider the following example where we use break
to exit a for
loop when a certain condition is met:
package main import "fmt" func main() { for i := 0; i < 10; i++ { if i == 5 { break } fmt.Println(i) } }
In this example, the loop will print numbers 0 through 4. When i
equals 5, the break
statement is executed, terminating the loop.
Using 'break' in 'switch' Statements
In Go, each case in a switch
statement is automatically terminated, meaning there's no implicit fall-through as in some other languages. However, the break
statement can still be used within a switch
to exit not just the current case but an enclosing loop or to provide clarity in code structure.
Example: Exiting a 'switch' Within a Loop
package main import "fmt" func main() { for i := 0; i < 3; i++ { switch i { case 0: fmt.Println("Zero") case 1: fmt.Println("One") case 2: fmt.Println("Two") break // This break exits the switch, not the for loop } } }
In this scenario, the break
statement exits the switch
statement when i
is 2, but the for
loop continues to its next iteration.
Labeled 'break' Statements
Go also supports labeled break
statements, which allow you to specify which loop to terminate, especially useful in nested loop scenarios.
Example: Using Labeled 'break' in Nested Loops
package main import "fmt" func main() { outerLoop: for i := 0; i < 3; i++ { for j := 0; j < 3; j++ { if i == 1 && j == 1 { break outerLoop } fmt.Printf("i = %d, j = %d\n", i, j) } } }
Here, when i
is 1 and j
is 1, the break outerLoop
statement terminates the outerLoop
, effectively exiting both loops. The output will be:
i = 0, j = 0
i = 0, j = 1
i = 0, j = 2
i = 1, j = 0
Conclusion
The break
statement in Go is a powerful tool for controlling the flow of loops and switch statements. Understanding its usage, including labeled breaks, enhances the ability to write efficient and readable Go code.
FAQs
Yes, by using a labeled break
, it can exit specific nested loops.
No, Go's switch
auto-terminates each case, but break
can be used for clarity.
It will exit only the nearest enclosing loop or switch
statement.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ