Understanding Golang's Format Strings
James Reed
Infrastructure Engineer · Leapcell
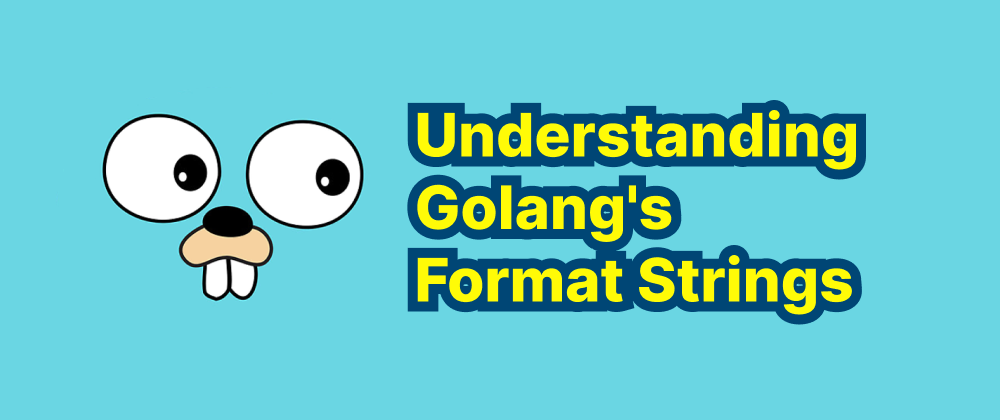
Key Takeaways
- The
fmt
package in Go provides flexible formatting for strings, numbers, and data types. - Format specifiers like
%s
,%d
, and%f
control the formatting of various data types. - Width, precision, and alignment options allow for customized output formatting.
Golang, commonly known as Go, provides a powerful and flexible way to format strings through its fmt
package. This package offers various functions that allow developers to format strings, numbers, and other data types in a readable and customizable manner.
Basic String Formatting
The fmt
package's Sprintf
function is commonly used to format strings. It takes a format specifier and corresponding values, returning the formatted string:
package main import ( "fmt" ) func main() { name := "Alice" age := 30 formattedString := fmt.Sprintf("Name: %s, Age: %d", name, age) fmt.Println(formattedString) }
Output:
Name: Alice, Age: 30
In this example:
%s
is a placeholder for a string.%d
is a placeholder for an integer.
Common Format Specifiers
Here are some frequently used format specifiers in Go:
%v
: Default format for the value.%#v
: Go-syntax representation of the value.%T
: Type of the value.%%
: A literal percent sign.
For integers:
%b
: Binary representation.%c
: Character corresponding to the Unicode code point.%d
: Decimal representation.%o
: Octal representation.%x
: Hexadecimal representation (with letters in lowercase).%X
: Hexadecimal representation (with letters in uppercase).
For floating-point and complex numbers:
%e
: Scientific notation (e.g.,1.23e+03
).%f
: Decimal point but no exponent (e.g.,123.456
).%g
: Uses%e
or%f
based on the value's precision.
Width and Precision
You can control the width and precision of the formatted output. For example:
package main import ( "fmt" ) func main() { pi := 3.141592653589793 formattedPi := fmt.Sprintf("%.2f", pi) fmt.Println(formattedPi) }
Output:
3.14
Here, %.2f
formats the floating-point number to two decimal places.
Padding and Alignment
Golang allows padding and aligning numbers and strings:
%6d
: Pads the integer with spaces to ensure it's at least 6 characters wide.%-6d
: Left-aligns the integer within the 6-character width.%06d
: Pads the integer with zeros to ensure it's at least 6 characters wide.
Example:
package main import ( "fmt" ) func main() { number := 42 fmt.Printf("%6d\n", number) // Right-aligned with spaces fmt.Printf("%-6d\n", number) // Left-aligned fmt.Printf("%06d\n", number) // Zero-padded }
Output:
42
42
000042
Formatting Strings
For strings, you can specify the width and alignment:
%10s
: Right-aligns the string within a 10-character width.%-10s
: Left-aligns the string within a 10-character width.
Example:
package main import ( "fmt" ) func main() { str := "Go" fmt.Printf("%10s\n", str) // Right-aligned fmt.Printf("%-10s\n", str) // Left-aligned }
Output:
Go
Go
Conclusion
Golang's fmt
package provides a comprehensive set of tools for formatting strings, numbers, and other data types. By mastering format specifiers, width, precision, and alignment options, developers can produce clear and well-structured output tailored to their specific needs.
FAQs
%v
prints the default format for any value.
Use %.2f
to format the float with two decimal places.
Use %06d
to format the integer with zero-padding.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ