How to Import a Local Package in Golang
Grace Collins
Solutions Engineer · Leapcell
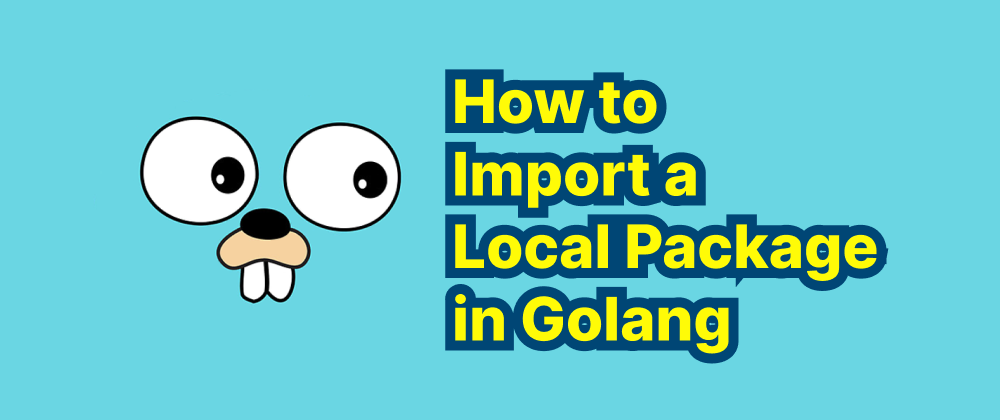
Key Takeaways
- Initialize a Go module using
go mod init
to manage local packages. - Import local packages using the module path (e.g.,
myproject/mypackage
). - Run the project from the root directory to ensure correct package resolution.
When working with Golang, structuring your code into multiple packages is a common practice for maintaining modularity and reusability. However, beginners often struggle with importing and using local packages correctly. This article will guide you through the process of importing local packages in Golang.
Understanding Go Modules and the GOPATH
Before importing a local package, it's important to understand how Go manages dependencies. Go uses a module system (go.mod
), which replaced the traditional GOPATH
method. The way you import local packages depends on whether your project is inside GOPATH
or a module-enabled workspace.
Setting Up a Golang Project with Local Packages
Let's create a simple Go project to demonstrate local package imports.
1. Create a Project Directory
First, create a new directory for your project:
mkdir myproject cd myproject
Initialize a Go module in the directory:
go mod init myproject
This command creates a go.mod
file, defining myproject
as the module name.
2. Create a Local Package
Inside the project directory, create a folder named mypackage
to hold the local package:
mkdir mypackage
Then, create a Go file inside mypackage
:
touch mypackage/mymodule.go
Edit mypackage/mymodule.go
to define a simple function:
package mypackage import "fmt" func Hello() { fmt.Println("Hello from mypackage!") }
3. Import the Local Package
Now, create a main.go
file in the root directory of the project:
touch main.go
Edit main.go
to import and use the local package:
package main import ( "myproject/mypackage" ) func main() { mypackage.Hello() }
4. Run the Code
Now, execute the program:
go run main.go
You should see the output:
Hello from mypackage!
Troubleshooting Common Issues
1. cannot find module providing package
If you see an error like:
cannot find module providing package myproject/mypackage
Make sure that:
- Your project has a valid
go.mod
file with the correct module path. - You are running
go run main.go
from the root directory of the project. - The package folder and file names match the import path.
2. go: cannot find main module
If your Go version is 1.17 or newer, ensure you are inside a module workspace:
go mod tidy
This command helps resolve dependencies.
Conclusion
Importing local packages in Golang is straightforward once you understand the module system. By structuring your code into separate packages, you can improve reusability and maintainability. Always ensure that your module paths are correct and that you initialize your Go project properly.
FAQs
Ensure the go.mod
file is correctly initialized and the import path matches the module structure.
No, with Go modules (go.mod
), GOPATH
is no longer required.
Run go mod tidy
to clean up and resolve module dependencies.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ