Mastering Rollup.js: A Deep Dive
Grace Collins
Solutions Engineer · Leapcell
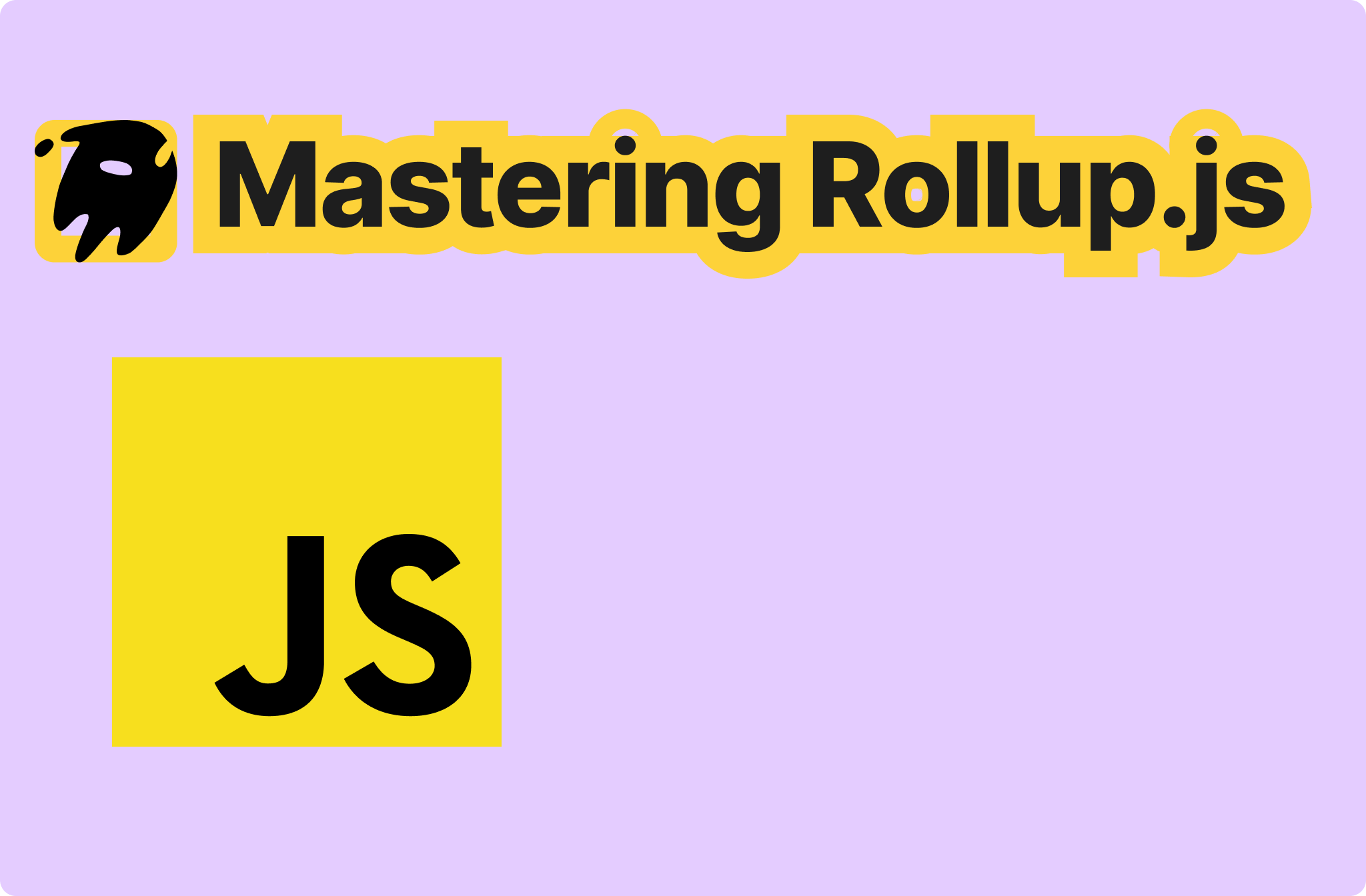
Introduction to the Basic Usage of Rollup.js
Rollup.js is a JavaScript bundling tool. This article will introduce its basic usage in detail.
I. Introduction
The Role of Bundling Tools
Bundling tools can combine multiple JavaScript scripts into one script for use in browsers. There are mainly three reasons why browsers need script bundling:
- Early Browser Module Support Issues: Early browsers did not support modules. For large - scale web projects, multiple scripts had to be combined into a single script before execution.
- Module Mechanism Compatibility: The module mechanism of Node.js is not compatible with browsers. It must be processed through a bundling tool to be used properly in browsers.
- Performance Optimization: From a performance perspective, it is better for a browser to load one large script than multiple small scripts. This is because it reduces the number of HTTP requests, thereby improving the loading efficiency.
Currently, the most commonly used bundling tool is Webpack. It is powerful and can almost meet all kinds of complex bundling requirements. However, its configuration is complex, the learning cost is high, and it is relatively troublesome to use, which has always been criticized by developers.
Rollup.js was developed with the original intention of creating an easy - to - use ES module bundling tool. Users can use it directly without complex configuration. In this regard, it does a really good job. With continuous development, Rollup.js has also gained the ability to bundle CommonJS modules. However, when bundling CommonJS modules, complex configuration is required. In this case, it is actually not much simpler than Webpack.
Therefore, it is recommended to only use Rollup.js for bundling ES modules to fully leverage its advantages. The following content will show how simple it is to bundle ES modules using Rollup.js. If your project uses CommonJS modules, Rollup.js is not highly recommended because its advantages are not obvious. If you don't know much about the differences between ES modules and CommonJS modules, you can refer to the ES6 tutorial.
II. Installation
In this article, Rollup.js is installed globally. The command is as follows:
$ npm install --global rollup
However, it can also be used without installation. The method is to replace rollup
in all commands with npx rollup
.
When using it for the first time, you can run the following command to view the help information:
$ rollup --help # Or $ npx rollup --help
III. Examples
Next, use Rollup.js to bundle two simple scripts: the library file add.js
and the entry script main.js
.
add.js
const PI = 3.14; const E = 2.718; export function addPi(x) { return x + PI; } export function addE(x) { return x + E; }
In the above code, the module add.js
exports two utility functions addPi()
and addE()
.
main.js
import { addPi } from './add.js'; console.log(addPi(10));
In this code, the entry script main.js
loads the utility function addPi()
from add.js
.
Bundling Operation
Use Rollup.js to bundle. The command is as follows:
$ rollup main.js
When bundling, only the entry script main.js
needs to be specified, and Rollup will automatically bundle in the dependencies. The bundling result is output to the screen by default, and the content is as follows:
const PI = 3.14; function addPi(x) { return x + PI; } console.log(addPi(10));
It can be seen that the import
and export
statements have disappeared and been replaced with the original code. In addition, the function addE()
was not bundled in because it was not used in main.js
. This feature is called tree - shaking, that is, automatically deleting unused code during bundling. Because of the above two points, the code output by Rollup is very clean and smaller in size than that of other bundling tools.
If you want to save the bundling result to a specified file, you can use the parameter --file [FILENAME]
. The command is as follows:
$ rollup main.js --file bundle.js
The above command will save the bundling result to the bundle.js
file.
IV. Usage Precautions
Multiple Entry Scripts
If there are multiple entry scripts, you need to fill in their file names in sequence and use the parameter --dir
to specify the output directory. For example:
$ rollup m1.js m2.js --dir dist
The above command will bundle and generate multiple files in the dist
directory, including m1.js
, m2.js
, and their common dependencies (if any).
Automatic Execution Function Wrapping
Using the parameter --format iife
will place the bundling result inside an immediately - invoked function expression. The command is as follows:
$ rollup main.js --format iife
Code Minimization
If you want to minimize the code after bundling, you can use the parameter --compact
. The command is as follows:
$ rollup main.js --compact
In addition, a specialized tool can also be used to achieve code minimization. For example:
$ rollup main.js | uglifyjs --output bundle.js
The above command is executed in two steps. The first step is to bundle with Rollup, and the second step is to use uglifyjs
for code minimization. Finally, the result is written to the bundle.js
file.
Configuration File
Rollup supports the use of a configuration file (rollup.config.js
), where all parameters are written. Here is an example:
// rollup.config.js export default { input: 'main.js', output: { file: 'bundle.js', format: 'es' } };
Use the parameter -c
to enable the configuration file. The command is as follows:
$ rollup -c
However, I do not recommend using the configuration file because it adds additional complexity. In the default scenario, command - line parameters are sufficient, and the expression of command - line parameters is also easier to read.
V. Conversion to CommonJS Modules
Finally, Rollup also supports converting ES modules to CommonJS modules. Just use the parameter --format cjs
. The command is as follows:
$ rollup add.js --format cjs
The converted CommonJS module code is as follows:
'use strict'; Object.defineProperty(exports, '__esModule', { value: true }); const PI = 3.14; const E = 2.718; function addPi(x) { return x + PI; } function addE(x) { return x + E; } exports.addE = addE; exports.addPi = addPi;
Leapcell: The Best Serverless Platform for Web Hosting, Async Tasks, and Redis
Finally, I would like to recommend the best platform for deploying JavaScript projects: Leapcell
1. Multi - Language Support
- Develop with JavaScript, Python, Go, or Rust.
2. Deploy unlimited projects for free
- Pay only for usage — no requests, no charges.
3. Unbeatable Cost Efficiency
- Pay - as - you - go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
4. Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real - time metrics and logging for actionable insights.
5. Effortless Scalability and High Performance
- Auto - scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the documentation!
Leapcell Twitter: https://x.com/LeapcellHQ