Extracting Substrings in Go
Ethan Miller
Product Engineer · Leapcell
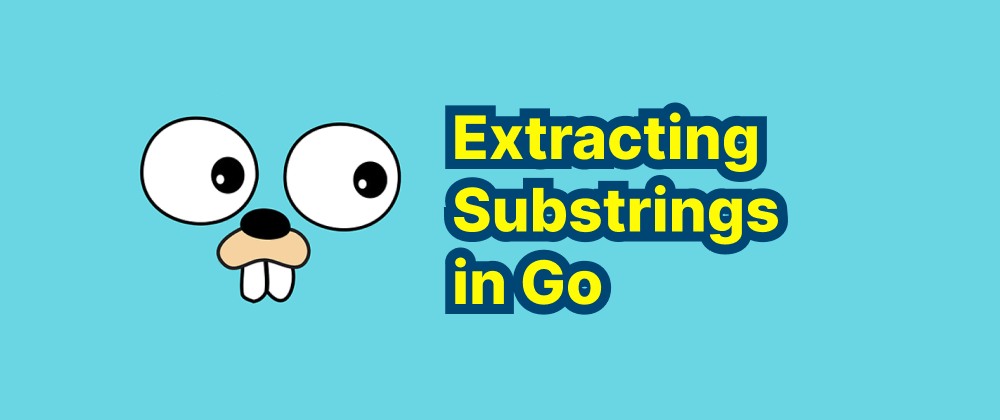
Key Takeaways
- Go strings can be sliced using index ranges for substring extraction.
- The
strings
package provides functions likeContains
,Index
, andSplit
for substring operations. strings.Builder
offers efficient string manipulation for performance-critical applications.
In Go, extracting substrings can be achieved using slices and the strings
package. This article explores various methods to extract substrings in Go, providing examples for clarity.
Using Slices
Go strings are essentially slices of bytes. You can extract a substring by specifying the start and end indices:
package main import ( "fmt" ) func main() { str := "Hello, World!" substr := str[7:12] fmt.Println(substr) // Output: World }
In this example, str[7:12]
extracts the substring from index 7 up to, but not including, index 12.
Note: Go uses UTF-8 encoding, so indexing may not work as expected with multi-byte characters. For such cases, consider using the utf8
package.
Using the strings
Package
The strings
package offers functions like HasPrefix
, HasSuffix
, Contains
, Index
, and Split
to work with substrings:
package main import ( "fmt" "strings" ) func main() { str := "Hello, World!" // Check if the string contains a substring contains := strings.Contains(str, "World") fmt.Println(contains) // Output: true // Find the index of a substring index := strings.Index(str, "World") fmt.Println(index) // Output: 7 // Split the string parts := strings.Split(str, ", ") fmt.Println(parts) // Output: [Hello World!] }
Using the strings.Builder
for Efficient String Manipulation
For scenarios involving multiple string concatenations or manipulations, strings.Builder
provides an efficient way to build strings:
package main import ( "fmt" "strings" ) func main() { var builder strings.Builder builder.WriteString("Hello") builder.WriteString(", ") builder.WriteString("World!") result := builder.String() fmt.Println(result) // Output: Hello, World! }
Conclusion
Extracting substrings in Go can be performed using slices for direct indexing or the strings
package for more advanced operations. Understanding these methods allows for effective string manipulation in Go applications.
FAQs
Use slicing with index ranges, e.g., str[7:12]
.
Use the utf8
package to correctly manage multi-byte characters.
It optimizes string concatenation and reduces memory allocations.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ