Sequelize vs TypeORM: Which JavaScript ORM Should You Choose?
James Reed
Infrastructure Engineer ยท Leapcell
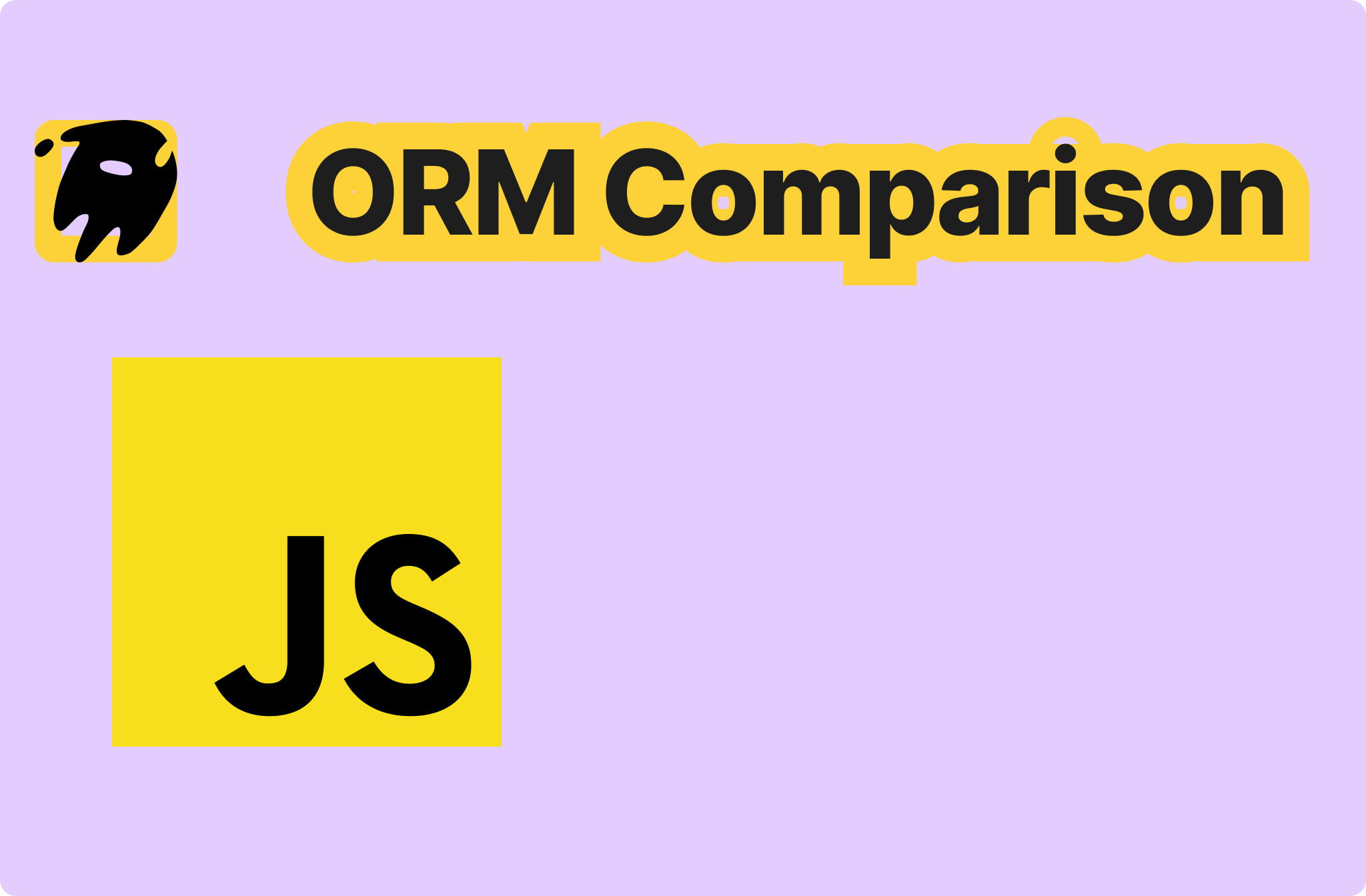
Comparison of Sequelize and TypeORM: A Guide to Choosing JavaScript ORM
1. Introduction
In today's Web development landscape, database operations are a core part of building applications. To simplify this process and improve development efficiency, various database operation libraries have emerged. This article focuses on comparing two popular JavaScript ORM (Object-Relational Mapping) tools: Sequelize and TypeORM. Both tools support multiple database systems and provide powerful ORM functions, helping developers handle database interactions more efficiently and intuitively. We will compare their characteristics from multiple dimensions and combine the advantages of the Leapcell cloud service deployment platform to provide a comprehensive selection reference for developers.
2. Introduction to Libraries and Community Status
2.1 Introduction to Sequelize
Sequelize is a Promise-based ORM based on Node.js, supporting multiple database systems such as MySQL, PostgreSQL, SQLite, and Microsoft SQL Server. With its powerful transaction processing capabilities, flexible association models, and easy-to-use API, Sequelize is widely recognized in the JavaScript community. Its query builder and migration tools enable developers to efficiently manage database schema changes.
2.2 Introduction to TypeORM
TypeORM is a decorator-based ORM that also supports multiple database systems. It is known for its type safety, modern decorator syntax, and extensive community support, particularly favored by TypeScript developers. TypeORM's design philosophy is "to operate the database as simply as writing code in TypeScript," providing powerful type checking and code organization capabilities for large projects.
The following are basic connection examples for the two ORMs:
// Sequelize connection example const { Sequelize } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql' }); // When deploying on the Leapcell platform, environment variables can be easily configured const sequelize = new Sequelize( process.env.DB_NAME, process.env.DB_USER, process.env.DB_PASSWORD, { host: process.env.DB_HOST, dialect: 'mysql' } );
// TypeORM connection example import { createConnection } from 'typeorm'; createConnection({ type: 'mysql', host: 'localhost', port: 3306, username: 'username', password: 'password', database: 'database' }); // Connection can be simplified through configuration files on the Leapcell platform import { createConnection } from 'typeorm'; import config from './ormconfig'; // Obtained from the Leapcell configuration center createConnection(config);
3. Comparison of Core Functions
3.1 Model Definition
Sequelize defines models using JavaScript classes and specifies attribute types and options through configuration objects:
const User = sequelize.define('user', { username: { type: Sequelize.STRING, allowNull: false }, birthday: { type: Sequelize.DATE } });
TypeORM uses decorator syntax, making model definitions more intuitive and type-safe:
import { Entity, PrimaryGeneratedColumn, Column } from 'typeorm'; @Entity() export class User { @PrimaryGeneratedColumn() id: number; @Column() username: string; @Column() birthday: Date; }
3.2 Query Building
Both ORMs support chained query building, but their syntaxes differ:
// Sequelize query example User.findAll({ where: { username: 'John Doe' }, attributes: ['username', 'birthday'] });
// TypeORM query example import { getRepository } from 'typeorm'; getRepository(User).createQueryBuilder('user') .select(['user.username', 'user.birthday']) .where('user.username = :username', { username: 'John Doe' }) .getMany();
On the Leapcell platform, regardless of the ORM used, real-time analysis of query performance and optimization of database operations can be achieved through its built-in monitoring tools.
3.3 Relationship Mapping
Sequelize defines relationships through model association methods:
const Post = sequelize.define('post', { /* ... */ }); User.belongsTo(Post); Post.hasMany(User);
TypeORM uses decorators to define relationships, making the code clearer:
import { Entity, OneToMany, ManyToOne } from 'typeorm'; @Entity() export class User { @OneToMany(() => Post, post => post.user) posts: Post[]; } @Entity() export class Post { @ManyToOne(() => User, user => user.posts) user: User; }
3.4 Migrations
Both ORMs provide database migration functions to help manage database schema changes:
// Sequelize migration example // Create a migration file npx sequelize-cli migration:generate --name=create-users // Execute migrations npx sequelize-cli db:migrate
// TypeORM migration example // Create a migration npx typeorm migration:create -n InitialMigration // Execute migrations npx typeorm migration:run
When deploying on the Leapcell platform, its automated deployment process can be used to integrate migration scripts into the CI/CD pipeline, achieving secure management of database changes.
4. Performance Comparison
Performance is a key consideration when selecting an ORM. We compare them from three aspects: query efficiency, memory usage, and execution speed:
4.1 Query Efficiency
Sequelize's query builder is flexible but may incur additional overhead when handling complex queries:
// Sequelize complex query example User.findAll({ include: [ { model: Post, include: [Comment] } ] });
TypeORM optimizes queries using the type system, catching some errors at compile time:
// TypeORM complex query example getRepository(User).createQueryBuilder('user') .leftJoinAndSelect('user.posts', 'post') .leftJoinAndSelect('post.comments', 'comment') .getMany();
4.2 Memory Usage
When processing large amounts of data, Sequelize's object serialization and deserialization may lead to higher memory usage, while TypeORM's type optimizations generally perform better.
4.3 Execution Speed
Due to differences in implementation, TypeORM typically has a slight advantage in execution speed, especially in complex query scenarios.
On the Leapcell platform, resource monitoring functions can be used to optimize performance for specific application scenarios and select the most suitable ORM.
5. Learning Curve and Community Support
5.1 Learning Curve
Sequelize has an intuitive API design and rich documentation, making it suitable for beginners to get started quickly:
// Sequelize quick start example const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('sqlite::memory:'); const User = sequelize.define('user', { username: DataTypes.STRING });
TypeORM requires developers to be familiar with TypeScript and decorator syntax, with a slightly steeper learning curve but stronger type safety:
// TypeORM quick start example import { Entity, PrimaryGeneratedColumn, Column } from 'typeorm'; @Entity() export class User { @PrimaryGeneratedColumn() id: number; @Column() username: string; }
5.2 Community Support
Both have active communities, but as a more mature project, Sequelize has richer community resources. TypeORM, on the other hand, is growing rapidly in the TypeScript community.
6. Analysis of Practical Application Cases
6.1 Social Media Platform Case
When dealing with complex data models such as users, posts, and follow relationships:
Sequelize's flexibility allows it to easily handle many-to-many relationships:
// Sequelize social media model example const User = sequelize.define('user', { /* ... */ }); const Post = sequelize.define('post', { /* ... */ }); const Follow = sequelize.define('follow', { /* ... */ }); User.belongsToMany(Post, { through: 'user_posts' }); Post.belongsToMany(User, { through: 'user_posts' }); User.belongsToMany(User, { as: 'follower', through: Follow });
TypeORM's type safety can effectively reduce type errors in large projects:
// TypeORM social media model example @Entity() export class User { @OneToMany(() => Post, post => post.author) posts: Post[]; @ManyToMany(() => User, user => user.following) @JoinTable() following: User[]; @ManyToMany(() => User, user => user.followers) followers: User[]; }
6.2 E-commerce Platform Case
When dealing with relationships between products, orders, and users:
Sequelize's transaction support ensures the atomicity of order processing:
// Sequelize e-commerce model example const Product = sequelize.define('product', { /* ... */ }); const Order = sequelize.define('order', { /* ... */ }); const OrderProduct = sequelize.define('order_product', { /* ... */ }); Order.belongsToMany(Product, { through: OrderProduct }); Product.belongsToMany(Order, { through: OrderProduct });
TypeORM's type system provides stronger data validation capabilities:
// TypeORM e-commerce model example @Entity() export class Product { @OneToMany(() => OrderProduct, orderProduct => orderProduct.product) orderProducts: OrderProduct[]; } @Entity() export class Order { @OneToMany(() => OrderProduct, orderProduct => orderProduct.order) orderProducts: OrderProduct[]; } @Entity() export class OrderProduct { @ManyToOne(() => Product, product => product.orderProducts) product: Product; @ManyToOne(() => Order, order => order.orderProducts) order: Order; }
When deploying such applications on the Leapcell platform, its microservices architecture and automatic scaling functions can be used to easily handle high-concurrency scenarios.
7. Security and Maintenance
7.1 Security
Both provide SQL injection protection:
// Sequelize security example const User = sequelize.define('user', { username: { type: Sequelize.STRING, allowNull: false, validate: { len: { args: [3, 254], msg: 'Username must be between 3 and 254 characters' } } } });
// TypeORM security example import { Entity, Column, BeforeInsert } from 'typeorm'; import { hash } from 'bcryptjs'; @Entity() export class User { @Column() username: string; @Column() password: string; @BeforeInsert() async hashPassword() { this.password = await hash(this.password, 10); } }
7.2 Maintainability
Sequelize has complete documentation and a stable API; TypeORM's modular design and type system make the code easier to maintain. On the Leapcell platform, code analysis and automatic testing functions can be used to further improve code quality.
8. Conclusion
In summary, Sequelize is suitable for projects that pursue rapid development, flexible APIs, and extensive community support; TypeORM is more suitable for TypeScript projects and large applications that require strong type safety guarantees.
When choosing an ORM, it is recommended to consider project requirements, team technology stack, and long-term maintenance. At the same time, by leveraging the advantages of the Leapcell cloud service deployment platform, applications can be managed and scaled more efficiently. Regardless of the ORM chosen, an excellent development experience and operational performance can be obtained.
Leapcell: The Best of Serverless Web Hosting
Finally, we recommend the best platform for deploying services: Leapcell
๐ Build with Your Favorite Language
Develop effortlessly in JavaScript, Python, Go, or Rust.
๐ Deploy Unlimited Projects for Free
Only pay for what you useโno requests, no charges.
โก Pay-as-You-Go, No Hidden Costs
No idle fees, just seamless scalability.
๐ Explore Our Documentation
๐น Follow us on Twitter: @LeapcellHQ