Golang: Read Local SSH Config to Connect to Remote Servers
James Reed
Infrastructure Engineer · Leapcell
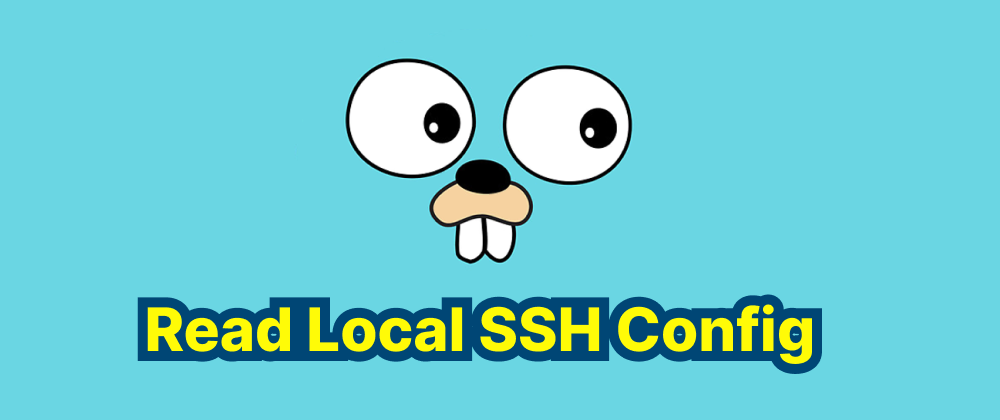
When developing Go applications that require connecting to remote servers via SSH, leveraging your local SSH configuration can streamline the process. This approach allows your Go application to utilize predefined connection settings, promoting consistency and efficiency.
Key Takeaways
- Go applications can utilize
~/.ssh/config
to streamline SSH connections. - The
github.com/kevinburke/ssh_config
package helps parse SSH configuration files in Go. - The
golang.org/x/crypto/ssh
package enables SSH client implementation in Go.
Understanding the Local SSH Config File
The SSH client configuration file, typically located at ~/.ssh/config
, enables users to define connection parameters for various hosts. A standard entry in this file might look like:
Host example HostName example.com User your-username Port 22 IdentityFile ~/.ssh/id_rsa
In this configuration:
- Host: An alias for the host; used as a shortcut in SSH commands.
- HostName: The actual domain or IP address of the remote server.
- User: The username for the SSH connection.
- Port: The port on which the SSH service is running (default is 22).
- IdentityFile: The path to the private key for authentication.
By configuring these settings, you can connect to the remote server using a simplified command:
ssh example
Integrating SSH Configurations in Go
To have your Go application read and utilize these SSH configurations, follow these steps:
-
Read the SSH Config File: Open and parse the
~/.ssh/config
file to retrieve the necessary host configurations. -
Extract Host Information: Identify the specific host entry your application needs to connect to and extract parameters such as
HostName
,User
,Port
, andIdentityFile
. -
Configure the SSH Client in Go: Use the extracted parameters to set up your SSH client within the Go application.
Implementing the Solution in Go
Go's golang.org/x/crypto/ssh
package facilitates SSH client implementations. However, it doesn't natively parse SSH config files. To bridge this gap, you can use the github.com/kevinburke/ssh_config
package, which provides functionality to parse SSH configuration files.
First, install the package:
go get github.com/kevinburke/ssh_config
Then, implement the following code to set up the SSH connection:
package main import ( "fmt" "io/ioutil" "log" "os" "path/filepath" "github.com/kevinburke/ssh_config" "golang.org/x/crypto/ssh" ) func main() { host := "example" // The alias defined in your SSH config // Locate the SSH config file sshConfigPath := filepath.Join(os.Getenv("HOME"), ".ssh", "config") // Open and parse the SSH config file configFile, err := os.Open(sshConfigPath) if err != nil { log.Fatalf("Failed to open SSH config file: %v", err) } defer configFile.Close() sshConfig, err := ssh_config.Decode(configFile) if err != nil { log.Fatalf("Failed to parse SSH config file: %v", err) } // Extract the relevant configurations for the host hostname := sshConfig.Get(host, "HostName") if hostname == "" { log.Fatalf("HostName not found for host: %s", host) } user := sshConfig.Get(host, "User") if user == "" { user = os.Getenv("USER") // Default to the current user if not specified } port := sshConfig.Get(host, "Port") if port == "" { port = "22" // Default SSH port } identityFile := sshConfig.Get(host, "IdentityFile") if identityFile == "" { identityFile = filepath.Join(os.Getenv("HOME"), ".ssh", "id_rsa") // Default key } // Read the private key key, err := ioutil.ReadFile(identityFile) if err != nil { log.Fatalf("Unable to read private key: %v", err) } // Create the Signer for the private key signer, err := ssh.ParsePrivateKey(key) if err != nil { log.Fatalf("Unable to parse private key: %v", err) } // Set up the SSH client configuration clientConfig := &ssh.ClientConfig{ User: user, Auth: []ssh.AuthMethod{ ssh.PublicKeys(signer), }, HostKeyCallback: ssh.InsecureIgnoreHostKey(), // For simplicity; consider implementing host key verification } // Connect to the SSH server connection, err := ssh.Dial("tcp", fmt.Sprintf("%s:%s", hostname, port), clientConfig) if err != nil { log.Fatalf("Failed to dial: %v", err) } defer connection.Close() // Now you can use 'connection' to interact with the remote server // For example, open a session: session, err := connection.NewSession() if err != nil { log.Fatalf("Failed to create session: %v", err) } defer session.Close() // Run a command on the remote server output, err := session.CombinedOutput("uname -a") if err != nil { log.Fatalf("Failed to run command: %v", err) } fmt.Println(string(output)) }
FAQs
Using SSH config improves security, avoids hardcoded credentials, and ensures consistency across environments.
The github.com/kevinburke/ssh_config
package can read and extract SSH configurations programmatically.
The parser will use the most specific match, following SSH's standard precedence rules.
Key Considerations
-
HostKeyCallback: In the example above,
ssh.InsecureIgnoreHostKey()
is used for simplicity, which bypasses host key verification. For production applications, implement proper host key verification to ensure security. -
Error Handling: Ensure robust error handling to manage scenarios where the SSH config file is missing entries or contains incorrect information.
-
Security: Always protect your private keys and avoid hardcoding sensitive information within your application.
By integrating your Go application with the local SSH configuration, you can manage SSH connections more efficiently, leveraging existing configurations and maintaining consistency across your development environment.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ