Understanding Bitwise Operations in Go
Grace Collins
Solutions Engineer · Leapcell
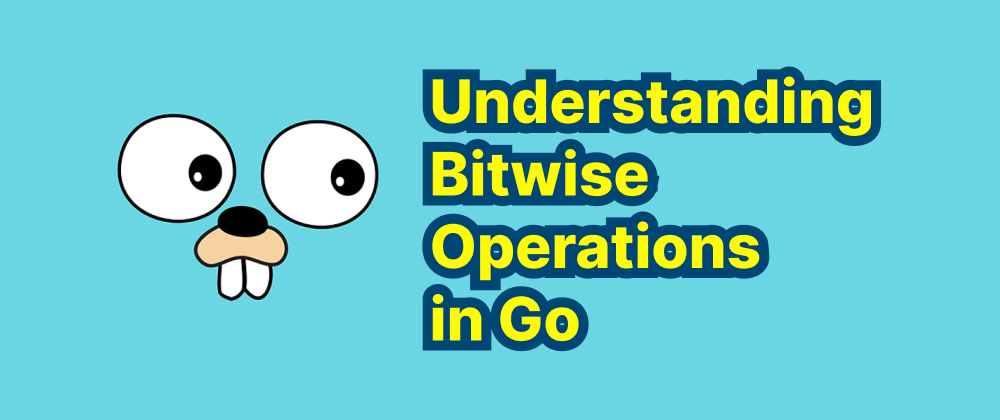
Key Takeaways
- Bitwise operators in Go enable efficient manipulation of integer values at the binary level.
- Common bitwise operations include AND, OR, XOR, AND NOT, and bit shifting.
- These operations are useful for optimizing arithmetic, data processing, and low-level programming.
Bitwise operations are fundamental to low-level programming and are often used in performance-critical applications. In Go, these operations allow manipulation of individual bits within an integer type, providing efficient data processing capabilities. This article explores the various bitwise operators available in Go and demonstrates their practical applications.
Bitwise Operators in Go
Go supports several bitwise operators, each performing a specific operation on the binary representation of integers:
&
(AND): Performs a bitwise AND operation.|
(OR): Performs a bitwise OR operation.^
(XOR): Performs a bitwise exclusive OR operation.&^
(AND NOT): Clears specific bits.<<
(Left Shift): Shifts bits to the left.>>
(Right Shift): Shifts bits to the right.
Bitwise AND (&
)
The bitwise AND operator compares each bit of two integers and returns a new integer where each bit is set to 1 only if both corresponding bits of the operands are 1.
a := 12 // 1100 in binary b := 10 // 1010 in binary result := a & b // 1000 in binary, which is 8
In this example, the binary representation of 12 (1100
) and 10 (1010
) are ANDed together, resulting in 8 (1000
).
Bitwise OR (|
)
The bitwise OR operator compares each bit of two integers and returns a new integer where each bit is set to 1 if at least one of the corresponding bits of the operands is 1.
a := 12 // 1100 in binary b := 10 // 1010 in binary result := a | b // 1110 in binary, which is 14
Here, the OR operation results in 14 (1110
).
Bitwise XOR (^
)
The bitwise XOR operator compares each bit of two integers and returns a new integer where each bit is set to 1 only if one of the corresponding bits is 1, but not both.
a := 12 // 1100 in binary b := 10 // 1010 in binary result := a ^ b // 0110 in binary, which is 6
The XOR operation yields 6 (0110
).
Bitwise AND NOT (&^
)
The AND NOT operator clears specific bits in the first operand where the corresponding bits of the second operand are set to 1.
a := 12 // 1100 in binary b := 10 // 1010 in binary result := a &^ b // 0100 in binary, which is 4
This operation results in 4 (0100
).
Left Shift (<<
) and Right Shift (>>
)
The left shift operator (<<
) shifts the bits of the first operand to the left by the number of positions specified by the second operand, effectively multiplying the number by 2 for each shift position. Conversely, the right shift operator (>>
) shifts the bits to the right, dividing the number by 2 for each shift position.
a := 3 // 0011 in binary leftShift := a << 2 // 1100 in binary, which is 12 rightShift := a >> 1 // 0001 in binary, which is 1
In this example, left-shifting 3 by 2 positions results in 12, and right-shifting 3 by 1 position results in 1.
Practical Applications
Bitwise operations are commonly used in scenarios such as:
- Setting, clearing, and toggling specific bits: For example, setting a particular bit to 1 or 0 in a bitmask.
- Performing efficient arithmetic operations: Multiplication or division by powers of two using shift operators.
- Implementing low-level protocols: Manipulating data at the bit level for tasks like checksum calculations or data compression.
Understanding and utilizing bitwise operations in Go can lead to more efficient and performant code, especially in systems programming and applications requiring direct hardware manipulation.
FAQs
They are used for bitwise masking, efficient arithmetic, and low-level protocol handling.
It shifts bits left, effectively multiplying the number by 2 for each shift.
It clears bits in the first operand where the corresponding bits in the second operand are 1.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ