Understanding Go's Abstract Syntax Tree (AST)
Lukas Schneider
DevOps Engineer · Leapcell
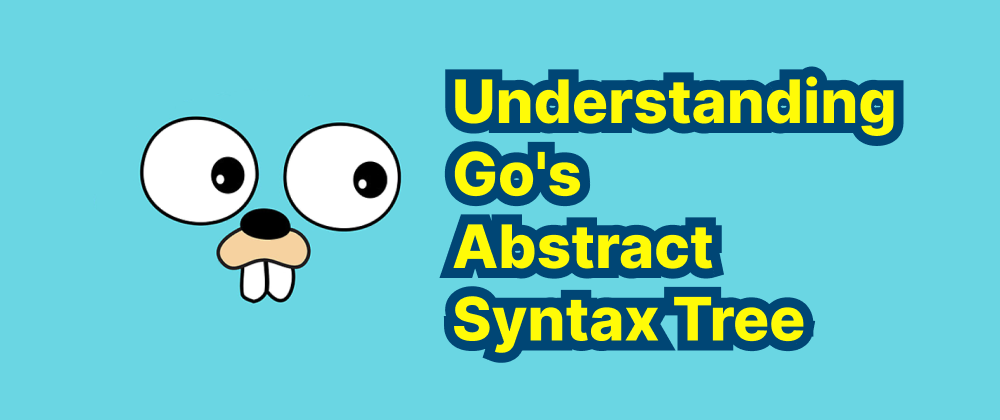
Key Takeaways
- Go's AST provides a structural representation of source code, enabling analysis and transformation.
- The
go/ast
package allows developers to parse, inspect, and modify Go code programmatically. - ASTs are crucial for tools like
go fmt
,go vet
, and automated code refactoring.
Go, often referred to as Golang, is a statically typed, compiled programming language designed for simplicity and efficiency. One of its powerful features is the ability to manipulate source code through its Abstract Syntax Tree (AST). This article delves into the concept of ASTs in Go, their significance, and how developers can leverage them for various applications.
What is an Abstract Syntax Tree (AST)?
An Abstract Syntax Tree (AST) is a tree representation of the abstract syntactic structure of source code. Each node in the tree denotes a construct occurring in the source code. The primary purpose of an AST is to provide a hierarchical, structural representation of the code, abstracting away specific syntax details like punctuation and formatting.
Importance of AST in Go
In Go, the AST serves several crucial purposes:
- Code Analysis: Tools can analyze Go code for potential issues, code quality metrics, or adherence to coding standards by traversing the AST.
- Code Transformation: Developers can programmatically modify Go code, enabling tasks like automated refactoring or code generation.
- Tooling Development: Many of Go's tools, such as
go fmt
andgo vet
, utilize the AST to perform their functions, ensuring code is formatted correctly and free of common mistakes.
Working with Go's AST
Go provides a robust package, go/ast
, within its standard library to work with ASTs. Here's a basic overview of how to use it:
Parsing Source Code
To construct an AST from Go source code, you can use the parser
package:
package main import ( "go/parser" "go/token" "log" ) func main() { src := ` package main import "fmt" func main() { fmt.Println("Hello, World!") }` fset := token.NewFileSet() node, err := parser.ParseFile(fset, "", src, parser.AllErrors) if err != nil { log.Fatal(err) } // 'node' now contains the AST of the source code }
In this snippet:
token.NewFileSet()
creates a set of source files.parser.ParseFile
parses the source code and returns the AST root node.
Traversing the AST
Once you have the AST, you might want to traverse it to analyze or modify the code. The ast.Inspect
function facilitates this:
package main import ( "fmt" "go/ast" "go/parser" "go/token" "log" ) func main() { src := ` package main import "fmt" func main() { fmt.Println("Hello, World!") }` fset := token.NewFileSet() node, err := parser.ParseFile(fset, "", src, parser.AllErrors) if err != nil { log.Fatal(err) } ast.Inspect(node, func(n ast.Node) bool { switch x := n.(type) { case *ast.BasicLit: fmt.Printf("Literal: %s\n", x.Value) } return true }) }
This code will output:
Literal: "Hello, World!"
Here, ast.Inspect
traverses the AST, and the provided function processes each node. In this case, it identifies and prints literal values.
Practical Applications
Understanding and manipulating Go's AST can lead to various practical applications:
- Code Formatting: Tools like
go fmt
use the AST to format code consistently. - Static Analysis:
go vet
analyzes code for potential errors by examining its AST. - Code Generation: Developers can generate Go code programmatically by constructing an AST and then printing it.
- Refactoring Tools: Automated tools can refactor codebases by modifying the AST directly.
Conclusion
The Abstract Syntax Tree is a foundational component in Go's tooling ecosystem. By providing a structured representation of source code, it enables powerful code analysis and transformation capabilities. Whether you're building developer tools, performing static analysis, or generating code, a solid understanding of Go's AST is invaluable.
FAQs
An AST is a tree representation of Go source code, abstracting syntax details for analysis and transformation.
Use the ast.Inspect
function to visit each node and analyze or modify specific elements.
Code formatting, static analysis, refactoring, and automated code generation.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ