Generating Random Numbers in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
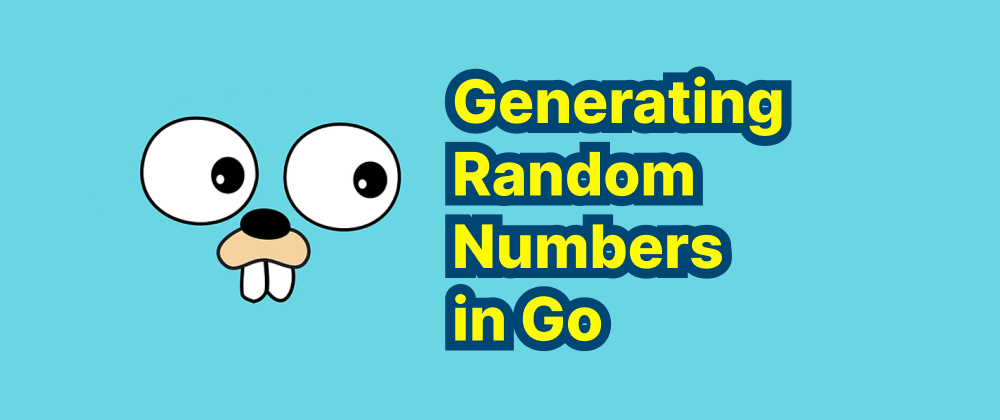
Key Takeaways
- The
math/rand
package provides pseudo-random number generation in Go. - Seeding with
time.Now().UnixNano()
ensures different sequences across runs. - Use
crypto/rand
for cryptographic randomness instead ofmath/rand
.
Random numbers are essential in various programming scenarios, such as simulations, games, and security protocols. In Go, the math/rand
package provides functionalities for generating pseudo-random numbers. This article explores how to utilize this package effectively.
Importing the math/rand
Package
To begin, import the necessary packages:
import ( "fmt" "math/rand" "time" )
Generating Integer Values
The rand.Intn
function returns a non-negative pseudo-random integer in the range [0, n)
. For example:
fmt.Print(rand.Intn(100), ",") fmt.Print(rand.Intn(100)) fmt.Println()
This code produces two random integers between 0 and 99.
Generating Floating-Point Values
For floating-point numbers, rand.Float64
generates a pseudo-random number in the range [0.0, 1.0)
:
fmt.Println(rand.Float64())
To generate a floating-point number within a specific range, adjust the output accordingly:
fmt.Print((rand.Float64()*5)+5, ",") fmt.Print((rand.Float64() * 5) + 5) fmt.Println()
This snippet produces numbers in the range [5.0, 10.0)
.
Seeding the Random Number Generator
By default, Go's random number generator produces the same sequence of numbers each time the program runs because it uses a deterministic seed. To ensure different sequences across runs, provide a unique seed using the current time:
s1 := rand.NewSource(time.Now().UnixNano()) r1 := rand.New(s1)
Now, using r1
will yield different sequences on each execution:
fmt.Print(r1.Intn(100), ",") fmt.Print(r1.Intn(100)) fmt.Println()
Reproducing Sequences with Fixed Seeds
For testing or debugging purposes, you might want to reproduce the same sequence of random numbers. This can be achieved by using a fixed seed:
s2 := rand.NewSource(42) r2 := rand.New(s2) fmt.Print(r2.Intn(100), ",") fmt.Print(r2.Intn(100)) fmt.Println()
Using the same seed value (e.g., 42
) ensures that r2
produces the same sequence every time the program runs.
Note on Cryptographic Use
The math/rand
package is suitable for general purposes but not for cryptographic applications. For cryptographic randomness, consider using the crypto/rand
package, which provides functions like rand.Read
to generate secure random data.
Conclusion
The math/rand
package in Go offers straightforward methods for generating pseudo-random numbers. By understanding how to seed the generator and produce numbers within desired ranges, developers can effectively incorporate randomness into their applications. For more details and examples, refer to the Go by Example: Random Numbers page.
FAQs
Because it uses a deterministic seed; use time.Now().UnixNano()
to randomize it.
Use (rand.Float64() * 5) + 5
to scale the output.
Use crypto/rand
for security-sensitive applications, such as password generation.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ