How to Copy a Slice in Go
Grace Collins
Solutions Engineer · Leapcell
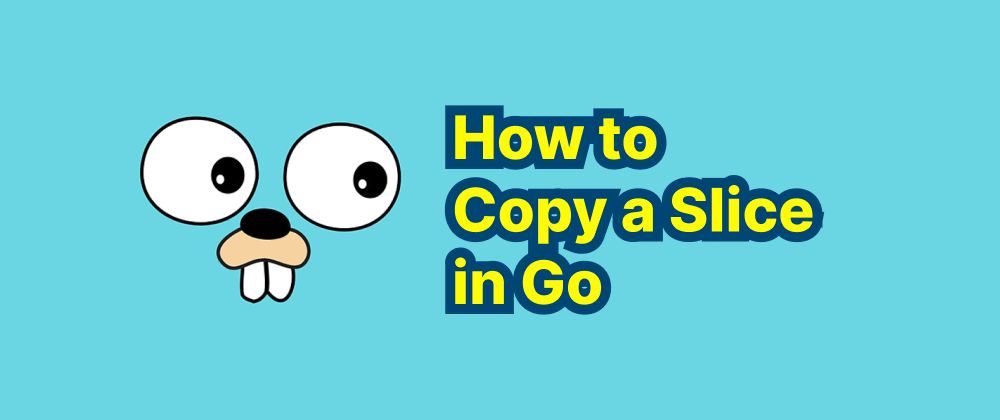
Key Takeaways
- Use the built-in
copy
function for efficient slice duplication. append
can also be used to create a new slice copy.- Be mindful of shallow vs. deep copies when handling reference types.
In Go, slices are references to underlying arrays, making copying them a nuanced task. Here's how you can effectively copy slices in Go.
Using the copy
Function
Go provides the built-in copy
function to duplicate slices. This function copies elements from a source slice to a destination slice. Here's how to use it:
package main import "fmt" func main() { // Original slice original := []int{1, 2, 3, 4, 5} // Create a new slice with the same length as the original copySlice := make([]int, len(original)) // Copy elements from the original slice to the new slice copy(copySlice, original) // Display the copied slice fmt.Println(copySlice) // Output: [1 2 3 4 5] }
In this example:
- We define an
original
slice containing integers. - We create a
copySlice
with the same length asoriginal
using themake
function. - The
copy
function duplicates the elements fromoriginal
tocopySlice
.
Copying a Portion of a Slice
You can also copy a subset of a slice by specifying the desired range:
package main import "fmt" func main() { // Original slice original := []int{1, 2, 3, 4, 5} // Create a new slice to hold a portion of the original copySlice := make([]int, 3) // Copy a subset of the original slice copy(copySlice, original[1:4]) // Display the copied slice fmt.Println(copySlice) // Output: [2 3 4] }
Here, we copy elements from index 1 to 3 (excluding index 4) of the original
slice into copySlice
.
Using Append to Copy a Slice
Alternatively, you can use the append
function to create a copy of a slice:
package main import "fmt" func main() { // Original slice original := []int{1, 2, 3, 4, 5} // Copy using append copySlice := append([]int{}, original...) // Display the copied slice fmt.Println(copySlice) // Output: [1 2 3 4 5] }
In this approach:
- We initialize an empty slice
[]int{}
. - We use
append
with the variadic argumentoriginal...
to append all elements oforiginal
to the new slice.
Important Considerations
-
Deep Copy vs. Shallow Copy: The methods above perform shallow copies, meaning they duplicate the slice structure but not the underlying data if the slice contains references (e.g., pointers, other slices). For deep copies, you'll need to manually copy each referenced element.
-
Capacity of the New Slice: When using
make
to create the destination slice, you can also specify its capacity. If the capacity is greater than the length, the new slice can accommodate additional elements without reallocating.
By understanding these methods, you can effectively manage slice copying in your Go programs.
FAQs
Only as many elements as the destination can hold will be copied.
append
creates a new slice with its own backing array, while copy
requires a pre-allocated slice.
No, only the elements are copied; modifying the new slice does not affect the original.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ