Mastering Time Parsing in Go with `time.Parse`
James Reed
Infrastructure Engineer · Leapcell
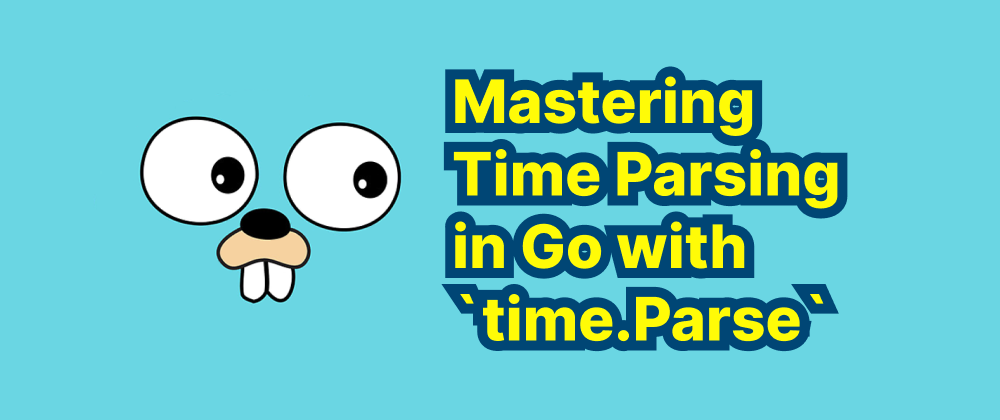
Key Takeaways
- Go's
time.Parse
uses a reference time format to interpret date strings. - Mismatched layouts and time zones can lead to parsing errors.
- Proper formatting ensures accurate time manipulation in applications.
In Go programming, parsing strings into time objects is a common task, often required when handling dates and times in various formats. The time
package provides the Parse
function to facilitate this conversion. Understanding how to utilize time.Parse
effectively is essential for accurate time manipulation in Go applications.
Understanding time.Parse
The time.Parse
function in Go interprets a given string according to a specified layout, returning the corresponding time.Time
value. The function signature is:
func Parse(layout, value string) (Time, error)
layout
: A string that defines the format of the input time string.value
: The actual time string to be parsed.
Defining the Layout
Go uses a unique approach to define the layout: a reference time is formatted in the specific pattern you expect your input string to follow. The reference time is:
Mon Jan 2 15:04:05 MST 2006
Each component of this reference time corresponds to a specific time or date element:
2006
: Year01
: Month02
: Day15
: Hour (24-hour clock)04
: Minute05
: SecondMST
: Time zone
For example, if you have a date in the format "Day/Month/Year Hour:Minute
layout := "02/01/2006 15:04:05"
Parsing a Time String
To parse the string "07/25/2019 13:45:00" using the layout defined above:
package main import ( "fmt" "time" ) func main() { layout := "02/01/2006 15:04:05" timeStr := "25/07/2019 13:45:00" t, err := time.Parse(layout, timeStr) if err != nil { fmt.Println("Error parsing time:", err) return } fmt.Println("Parsed time:", t) }
This will output:
Parsed time: 2019-07-25 13:45:00 +0000 UTC
Handling Different Time Formats
If your time string includes different components, adjust the layout accordingly. For instance, to parse a time in "hh
layout := "03:04 PM" timeStr := "11:22 PM" t, err := time.Parse(layout, timeStr)
In this case, the layout uses 03
for the hour, 04
for the minute, and PM
to denote the period of the day. This approach is particularly useful when dealing with time durations. For example, to calculate the number of seconds since midnight:
package main import ( "fmt" "time" ) func main() { layout := "03:04 PM" ref, _ := time.Parse(layout, "12:00 AM") t, err := time.Parse(layout, "11:22 PM") if err != nil { fmt.Println("Error parsing time:", err) return } fmt.Println("Seconds since midnight:", t.Sub(ref).Seconds()) }
This will output:
Seconds since midnight: 84120
This calculation determines the total number of seconds from midnight to 11:22 PM.
Common Pitfalls
- Mismatched Layout and Value: Ensure that the layout string accurately matches the format of the time string. A mismatch will result in a parsing error.
- Time Zones: If the time string includes a time zone, the layout must account for it using
MST
or-0700
formats. - Leading Zeros: The layout requires leading zeros for single-digit months, days, hours, minutes, and seconds. For example, January should be represented as
01
, not1
.
Conclusion
The time.Parse
function is a powerful tool in Go for converting strings into time.Time
objects. By defining the appropriate layout based on the reference time, you can accurately parse various date and time formats, enabling effective time manipulation in your applications.
FAQs
Go uses a fixed reference time (Mon Jan 2 15:04:05 MST 2006
) to interpret date formats.
Include the time zone in the layout, e.g., "02/01/2006 15:04:05 -0700"
.
time.Parse
returns an error, as it strictly follows the specified format.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ