Writing to Files in Go: A Comprehensive Guide
Daniel Hayes
Full-Stack Engineer · Leapcell
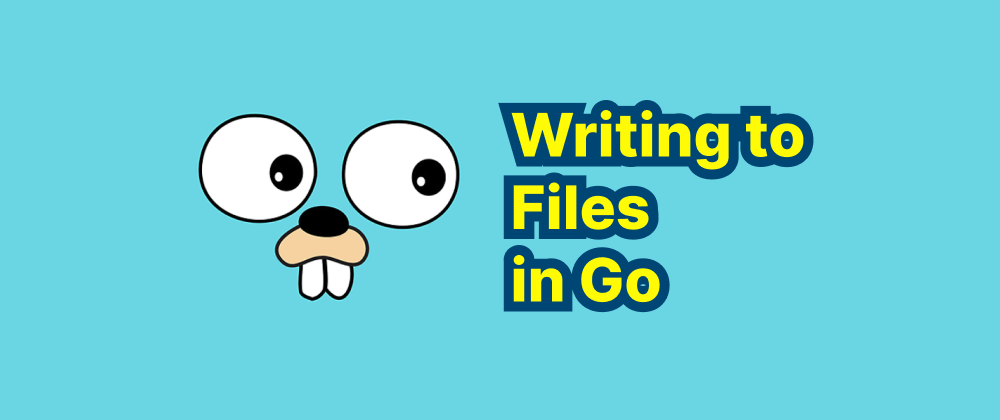
Key Takeaways
- Go provides multiple ways to write to files using
os
,bufio
, andioutil
(deprecated). - Proper error handling and resource management (
defer file.Close()
) are crucial. bufio
is best for efficient, buffered writes, reducing direct disk I/O.
Writing to a file in Go is a fundamental task that every developer should master. The Go standard library offers robust support for file operations through packages like os
, ioutil
, and bufio
. This article provides a comprehensive guide on how to write to files using these packages, complete with examples and best practices.
Using the os
Package
The os
package is the cornerstone for file operations in Go. It provides the necessary functions to create, open, and manipulate files. Here's how you can use it to write to a file:
package main import ( "fmt" "os" ) func main() { // Create a new file or open an existing one for writing file, err := os.Create("example.txt") if err != nil { fmt.Println("Error creating file:", err) return } // Ensure the file is closed after the function completes defer file.Close() // Write a string to the file _, err = file.WriteString("Hello, Gophers!") if err != nil { fmt.Println("Error writing to file:", err) return } fmt.Println("Data written successfully.") }
In this example:
os.Create
is used to create a new file or truncate an existing one. It returns anos.File
object and an error.defer file.Close()
ensures that the file is properly closed when the function exits, preventing resource leaks.file.WriteString
writes the specified string to the file. It returns the number of bytes written and an error, which should be checked to ensure the operation's success.
Using the ioutil
Package
The ioutil
package offers a more concise approach for simple file writing tasks. However, it's worth noting that as of Go 1.16, ioutil
has been deprecated, and its functions have been moved to other packages. For historical context, here's how ioutil.WriteFile
was used:
package main import ( "io/ioutil" "log" ) func main() { data := []byte("Hello, Gophers!") err := ioutil.WriteFile("example.txt", data, 0644) if err != nil { log.Fatal(err) } log.Println("Data written successfully.") }
In this snippet:
ioutil.WriteFile
writes data to the named file, creating it with the specified permissions if it doesn't exist, or truncating it before writing if it does.- The function takes three arguments: the filename, the data to write (as a byte slice), and the file permissions (in this case,
0644
).
Using the bufio
Package
For buffered writing, which can be more efficient for large amounts of data, the bufio
package is ideal:
package main import ( "bufio" "fmt" "os" ) func main() { // Open the file for writing file, err := os.OpenFile("example.txt", os.O_WRONLY|os.O_CREATE|os.O_TRUNC, 0644) if err != nil { fmt.Println("Error opening file:", err) return } // Ensure the file is closed after the function completes defer file.Close() // Create a buffered writer writer := bufio.NewWriter(file) // Write a string to the buffer _, err = writer.WriteString("Hello, Gophers!\n") if err != nil { fmt.Println("Error writing to buffer:", err) return } // Flush the buffer to ensure all data is written to the file err = writer.Flush() if err != nil { fmt.Println("Error flushing buffer:", err) return } fmt.Println("Data written successfully.") }
Here:
os.OpenFile
opens the file with specified flags (os.O_WRONLY
,os.O_CREATE
, andos.O_TRUNC
) and permissions (0644
).bufio.NewWriter
creates a buffered writer, which reduces the number of write operations by accumulating data in memory before writing it out.writer.Flush()
ensures that any buffered data is actually written to the file.
Best Practices
- Error Handling: Always check for errors after file operations to handle unexpected situations gracefully.
- Resource Management: Use
defer file.Close()
immediately after opening a file to ensure it gets closed properly, even if an error occurs later in the function. - Permissions: Set appropriate file permissions to ensure that the file is accessible only to intended users.
By understanding and utilizing these packages and practices, you can efficiently perform file write operations in Go, tailoring your approach to the specific needs of your application.
FAQs
Using os.Create
and file.WriteString()
.
It buffers data in memory before writing, improving performance for large writes.
Use defer file.Close()
immediately after opening the file.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ