How to Replace Substrings in Go Using `strings.Replace`
James Reed
Infrastructure Engineer · Leapcell
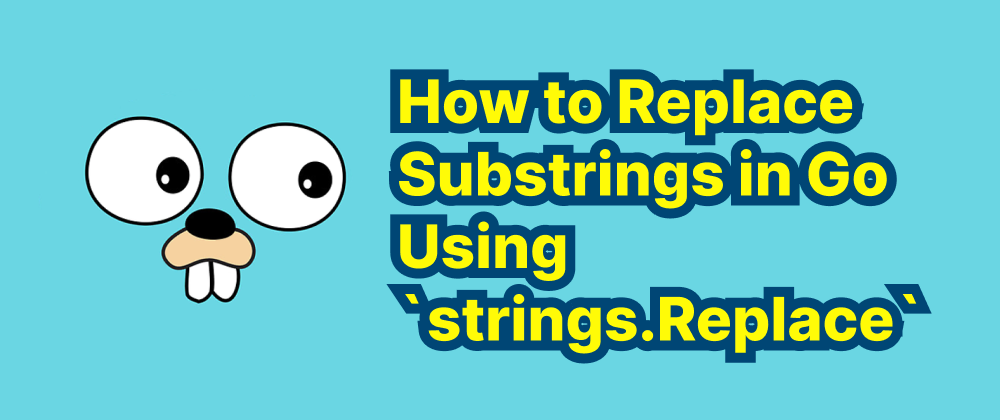
Key Takeaways
- The
strings.Replace
function allows replacing substrings in a case-sensitive manner. - Setting
n
to-1
ensures all occurrences are replaced. strings.NewReplacer
enables multiple substring replacements efficiently.
In Go programming, the strings
package provides the Replace
function to substitute occurrences of a specified substring within a string. The function signature is:
func Replace(s, old, new string, n int) string
Where:
s
is the original string.old
is the substring to be replaced.new
is the substring to replaceold
.n
specifies the number of non-overlapping instances to replace; ifn
is less than 0, all occurrences are replaced.
Usage Examples:
package main import ( "fmt" "strings" ) func main() { original := "Hello, World! Hello, Golang!" // Replace all occurrences result := strings.Replace(original, "Hello", "Hi", -1) fmt.Println(result) // Output: Hi, World! Hi, Golang! // Replace the first occurrence result = strings.Replace(original, "Hello", "Hi", 1) fmt.Println(result) // Output: Hi, World! Hello, Golang! // Replace the first two occurrences result = strings.Replace(original, "Hello", "Hi", 2) fmt.Println(result) // Output: Hi, World! Hi, Golang! }
Case Sensitivity:
The Replace
function is case-sensitive. For example:
package main import ( "fmt" "strings" ) func main() { original := "Hello, World! HELLO, Golang!" // Attempt to replace "hello" (lowercase) result := strings.Replace(original, "hello", "Hi", -1) fmt.Println(result) // Output: Hello, World! HELLO, Golang! }
In this case, "hello" does not match "Hello" or "HELLO", so no replacements occur.
Replacing Multiple Substrings:
For multiple replacements, the strings
package offers the Replacer
type:
package main import ( "fmt" "strings" ) func main() { original := "Hello, World! Hello, Golang!" replacer := strings.NewReplacer("Hello", "Hi", "World", "Earth") result := replacer.Replace(original) fmt.Println(result) // Output: Hi, Earth! Hi, Golang! }
The Replacer
allows for efficient and readable multiple substring replacements within a string.
By utilizing the strings.Replace
function and the Replacer
type, Go developers can effectively manage string substitutions in their applications.
FAQs
Use strings.Replace(s, old, new, -1)
.
Yes, it only replaces exact matches of old
.
Use strings.NewReplacer
for multiple replacements.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ